I'm trying to figure out how to remove the background image of the selected area which I have already generated. To make it clear understanding, I attach a visualization about what I need to solve.
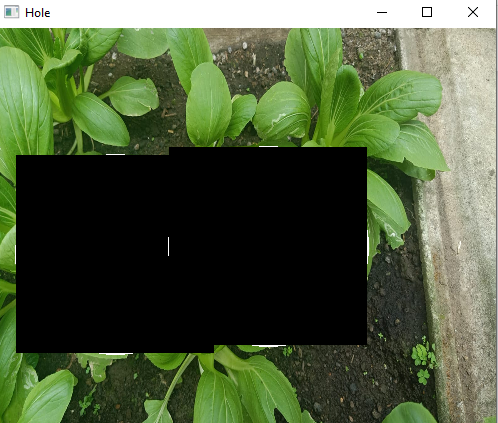
The black area is the selected area, and I want it to be reversed. So the selected area will become a colorful image, and the background will be black color.
Here's the simple code that I created already:
import cv2
import numpy as np
pic= cv2.imread('dataset/set.jpeg')
pic = cv2.resize(pic, dsize=(500, 400), interpolation=cv2.INTER_CUBIC)
gray=cv2.cvtColor(pic,cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray,(5,5),5)
_,thres = cv2.threshold(blur, 100,250, cv2.THRESH_TOZERO)
res = cv2.Canny(thres, 100, 200, L2gradient=True)
circles = cv2.HoughCircles(res,cv2.HOUGH_GRADIENT,1,20,param1=200,param2=15,minRadius=80,maxRadius=100)
for i in circles[0,:]:
# draw the outer circle
cv2.circle(pic,(int(i[0]),int(i[1])),int(i[2]),(255,255,255),2)
i = i.astype(int)
pic[i[1]-i[2]:i[1] i[2], i[0]-i[2]:i[0] i[2]] = 0
cv2.imshow('Hole',pic)
cv2.waitKey(0)
cv2.destroyAllWindows()
The problem is in this code:
pic[i[1]-i[2]:i[1] i[2], i[0]-i[2]:i[0] i[2]] = 0
I'm changing the selected area to become black (zero) but I don't know how to reverse it to become the only one who has color, and let the other become the black image. Please kindly help me to solve this, thank you!!
CodePudding user response:
The issue has been solved by creating masks and combine the foreground and background by these lines of code:
import cv2
import numpy as np
pic= cv2.imread('Assets/set.jpeg')
pic = cv2.resize(pic, dsize=(500, 400), interpolation=cv2.INTER_CUBIC)
gray=cv2.cvtColor(pic,cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray,(5,5),5)
_,thres = cv2.threshold(blur, 100,250, cv2.THRESH_TOZERO)
res = cv2.Canny(thres, 100, 250, L2gradient=True)
circles = cv2.HoughCircles(res,cv2.HOUGH_GRADIENT,1,20,param1=200,param2=15,minRadius=80,maxRadius=100)
circles = np.uint16(np.around(circles))
mask = np.full((res.shape[0], res.shape[1]), 1, dtype=np.uint8) # mask is only
clone = pic.copy()
for i in circles[0, :]:
cv2.circle(mask, (i[0], i[1]), i[2], (255, 255, 255), -1)
cv2.circle(clone, (i[0], i[1]), i[2], (255, 255, 255), 1)
# get first masked value (foreground)
fg = cv2.bitwise_or(res, res, mask=mask)
# get second masked value (background) mask must be inverted
mask = cv2.bitwise_not(mask)
background = np.full(res.shape, 255, dtype=np.uint8)
bk = cv2.bitwise_or(background, background, mask=mask)
# combine foreground background
final = cv2.bitwise_or(fg, bk)
result = np.concatenate((res,final),axis=1)
cv2.imshow('Hole',result)
cv2.waitKey(0)
cv2.destroyAllWindows()
Nothing to be asked anymore and I will close the question. Thank you!!