Stdio.h contains the basic input and output
Math. H contains mathematical knowledge
String. H contains a String of knowledge
The main main function to run the program to run the main function
Return0 said program runs out
Framework
# include
Int main ()
{
return 0;
}
1. The data typeThe basic data type
Integer
Integer (int) 4
Long integer (int) 8
Short integer (short int) 2
The decimal (floats)
Single number (fiot) 4
Double fine count (double) 8
Character (char) 1
Complex data types (resolve)
Structure (at most)
Enumeration
The appropriate
2 what is the variable
Nature: a storage space in memory
3 how to variable naming (study)
Name: by letters, Numbers, underscore composition and must be a letter or an underscore in the beginning, not the same as the keyword,
4 how to define a variable
Data type variable name=value;
Equivalent to
Data type variable names;
The variable name=value;
5 base
The decimal system: every 10 into 1
Binary: every 2 into 1
Octal: every 8 into 1
Hex. On 16 into 1
0 to 9 A to F
Conclusion: turn the decimal r hexadecimal: pour over r modulo
R turn hexadecimal decimal: pour access by 10 times the power (start from 0)
6 assignment amount how said
Integer
Output decimal to % d
Octal adding 0 to % o output
Hex adding 0 x % x to
Behind the front is 0 when the number is not greater than 8
The decimal
Traditional written 1.1 3.21
Scientific notation 3.2 e2==320
E==0.032 3.2
Characters
A single character 'a' must take bracketed by
'String
7 what is a byte
Byte is the unit of computer data storage, hardware can access the smallest unit of
1 b=8 bits (one for one)
1 KB=1024 b
1 m=1024 KB
1 gb=1024 m
1 TB=1024 g1 pb=1024 TB
Use printf output
1 printf (" the input control characters ")
Printf (" input control characters ", output parameters)
3 printf (" input control characters 1 input control characters 2 ", 1 output parameters, output parameters (2)
Printf (" the input control characters input control characters ", input parameters)
Input control operator
% d (decimal)
O (octal) % # % o
X (hexadecimal) % # % x
% f (output decimal)
% c (output characters)
Supplement: ACSSII code: specifies which integer with different characters a: 97 b: 98 z: 122 a: 65 b: 66 z: 90
Use the scanf output
Through the keyboard input: users will be assigned to the variable data
1 the scanf (" input control characters ", output parameters)
2 the scanf (" input control, the input control output parameter ")
Note: 1 statement as far as possible don't add the input control operator
2 use the scanf before, as far as possible to use printf prompt the user
The operator
The arithmetic operators
+ - */% (take over)
Relational operators
<>=& lt;===(equal to zero)!=(is not equal to)
Logical operators
! (a) & amp; & (and) | | (or)
True and false propositions false proposition
True proposition and true proposition true proposition
True or false proposition thesis
True proposition or true proposition true proposition
Supplement: zero in c language is true, zero false true in 1, said false expressed with 0
When two propositions' and ', the first a false proposition, the first proposition without
Perform when two propositions' or ', the first for the second proposition true proposition
Does not perform
The assignment operator
==a + +==/=*=3 is equivalent to a=a + 3=a - a - 3...
===for assignment is equal to
Supplement: since the increase: on the basis of its original plus one
Before the increase, said after add a value + + I
After the increase, said with a previous value i++
The decrement: on the basis of its original minus one
Before the decrement: it means the value of minus one after I
-After the decrement: plus one after the value of the I -
Supplement:/n line (not input control characters)
Process control:
Definition: code execution order
Executive order: from top to bottom
Condition: some code might not execute
If
1. If the simple use of the if (expression)
Statements;
2. If the scope of the problem if statements can only control one line if you want to control multiple lines, as long as add {... }
3. If... The use of the else
4. If... Else if... Established the else of usage: if don't have to perform the else
5. (program) to classify a score
Int main ()
{
Double sore;
Printf (" please enter your score: ");
The scanf (" % 1 f ", & amp; Sore)
If (sore> 100)
Printf (" dream! n");
Else if (sore>=90 & amp; & Sore<100)
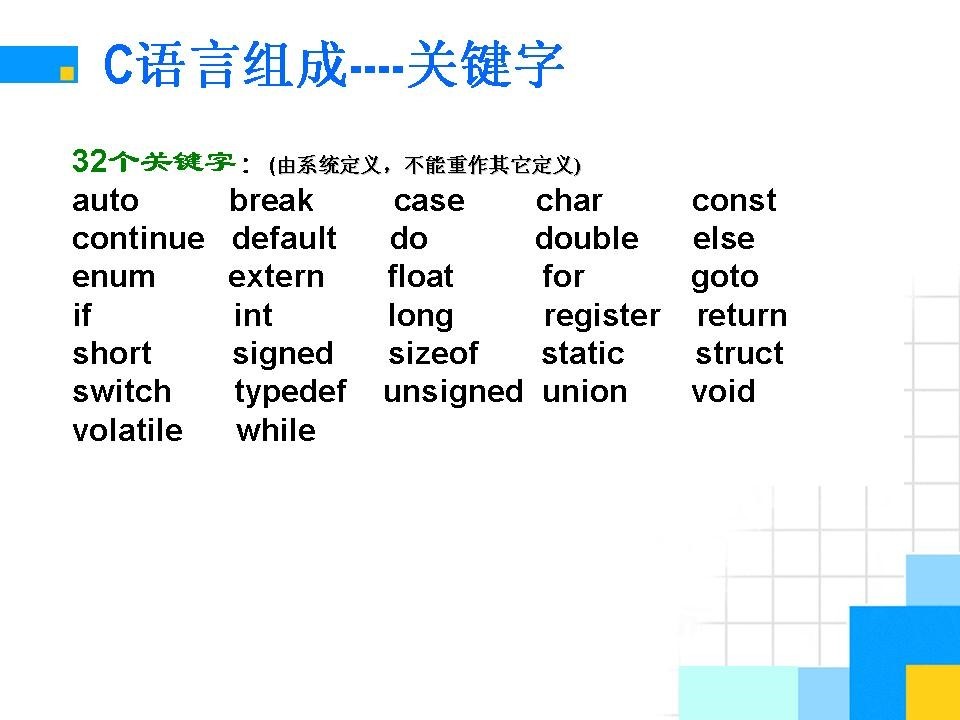
Else if (sore>=60 & amp; & Sore<90)
Printf (" qualified n ");
The else
Printf (" continue to work hard, not qualified! n");
return0;
Note: 1: empty statement
2: the true and false judgement
0 founded false non-zero for true expression is true, was not false
The switch
A yuan quadratic equation
#include
#include
Int main (void)//main function
{
Int a=1, b=5, c=2;
Float delt.
Delt=b * * a * b - 4 c.
If (delt> 0)
{
Printf (" this a yuan quadratic equation has two solutions!/n ");
X1=(a - b + SQRT (delt))/(2 * a);
X2=(a - b - SQRT (delt)/(2 * a);
Printf (" x1 x2=% f=% f ", x1, x2);
}
Else if (delt==0)
{
X1=(a - b)/(2 * a);
X2=x1;
Printf (" the a yuan quadratic equation has a solution, the x1 x2==% f/n ", the x1);
}
The else
{
Printf (" no solution/n ");
}
{
Supplement: the ternary operator
Cycle: some code is executed repeatedly
Cycle: 1. The While (expression, the loop body)
{
The loop body.
}
I=1... 100
Int I=1;
Int num=0;
While (i
=100){
Num=num + I;
i++;
}
Output num
Expression can't be empty, the non-zero is empty (zero false) does not comply with the conditions are not perform direct output
2. Do the while (first cycle again, no matter right or wrong)
{
The loop body. Num==num + I;
} while (expression) i++;
(i
=100)
Do not conform to the condition number to perform again first, then the output
3. For1 & gt; Assignment expression;
2> Relational expression;
3> Assignment expression;
(1) on the
(2) the decrement
For variant: it inside the loop condition can omit
Omit an assignment expression is the default starting from 1
Restrictions would omit relational expression without infinite loop
Number meaning is the abbreviation of digital it for NUM
99 times table
for(int i=1; i<=9; I++) {
For (int j=1; j<=I; J++) {
Printf (" ");
}
}
The for loop
#include
Int main ()
{
Int num=0;
for(int i=1; i<=100; I++)
{
Num=num + I;
}
Printf (" num=% d, num ");
return 0;
}
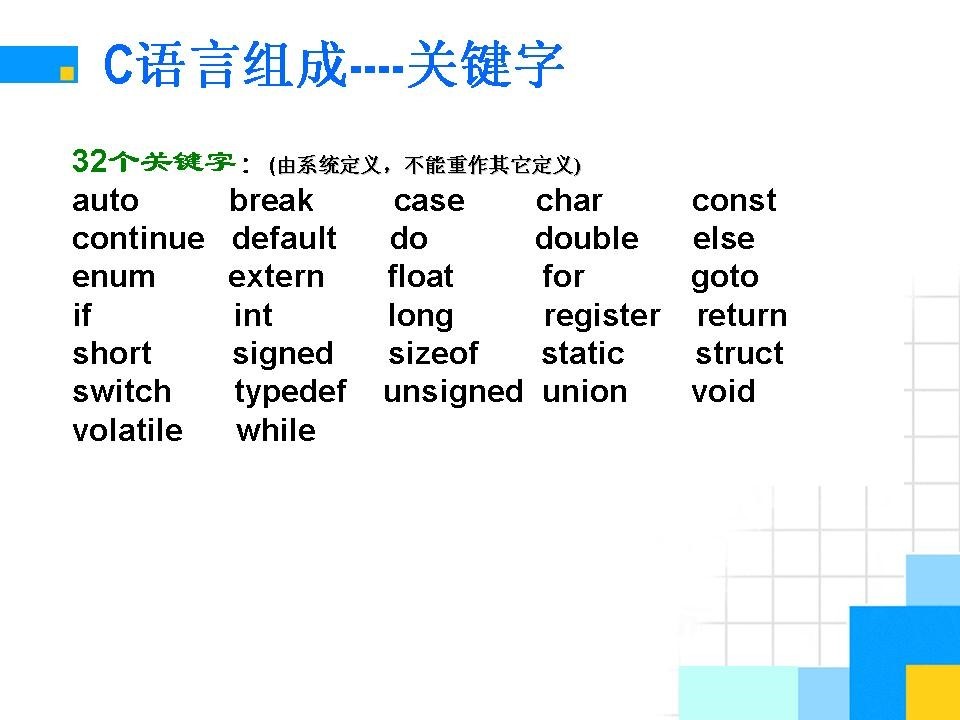