1) reverse establish a singly linked list
(2) the traversal singly linked lists (output singly linked lists of each element value)
(3) in a singly linked list for the fifth element before inserting the elements of a value of 999.
(4) delete singly linked lists for the fifth element.
# include & lt; stdio.h>
#include
Typedef struct Lnode//define singly linked list storage structure
{
The int data;
Struct Lnode * next;
} Lnode * linklist;
Void CreatList_L (linklist L, int n);
Void ListTraverse (linklist L, int n);
Void ListInsert (linklist L, int, int, e int n);//n for the node number in the list, I for the ith element inserted before, e is the value of the new node
Void ListDelete (linklist L, int, int * node, int n);//n for the node number in the list, I to delete the node location, e used to return the value of delete node
Void visit ();
Int main ()
{
Linklist L;
Int n, I=5, e=999, * node;
Printf (" please enter the number you want to build a singly linked list of nodes: \ n ");
The scanf (" % d ", & amp; n);
CreatList_L (m, n);//reverse singly linked lists, and will be the head of a singly linked list address is assigned to a pointer s
ListTraverse (m, n);//traverse singly linked list
ListInsert (L, I, e, n);//insert
ListDelete (L, I, node, n);//delete
}
Void CreatList_L (linklist L, int n)//reverse established
{
Printf (" -- -- -- -- -- reverse build -- -- -- -- -- - \ n ");
Linklist p;
int i;
L=(Lnode *) malloc (sizeof (Lnode));//set up leading the singly linked list of nodes
L - & gt; Next==NULL;
for(i=n; I> 0; I -)
{
P=(Lnode *) malloc (sizeof (Lnode));
Printf (" both please enter a number: \ n ");
The scanf (" % d ", & amp; p-> The data);
p-> Next=L - & gt; Next;
L - & gt; Next=p;
}
}
Void ListTraverse (linklist L, int n)//traverse singly linked list
{
Printf (" \ n \ n -- -- -- -- -- -- -- traversal -- -- -- -- -- -- -- -- \ n ");
int i;
Linklist p;
P=L - & gt; Next;
for(i=0; i{
Visit (p);
P=p - & gt; Next;
}
}
Void visit (linklist p)//output
{
Printf (" % d ", p - & gt; The data);
}
Void ListInsert (linklist L, int, int, e int n)//before the fifth element insert element
{
Printf (" \ n \ n -- -- -- -- -- insert element -- -- -- -- -- - \ n ");
Linklist p, q;
P=L;
//e=999;
//I=5;
Int j=0;
While ((p - & gt; Next) & amp; & j{
P=p - & gt; Next;
j++;
}
If (p - & gt; Next==NULL)//insert position unreasonable
{
Printf (" ERROR! Insert position is unreasonable!" );
exit(0);
}
Q=(Lnode *) malloc (sizeof (Lnode));//create new node
Q - & gt; data=https://bbs.csdn.net/topics/e;
Q - & gt; Next=p - & gt; Next;//connected to list
p-> Next=q;
Printf (" \ n insert success! \n");
Printf (" \ n the new list is: ");
P=L;
for(i=0; i{
P=p - & gt; Next;
Visit (p);
}
}
Void ListDelete (linklist L, int, int * node, int n)//delete the ith element
{
Printf (" \ n \ n -- -- -- -- -- remove elements -- -- -- -- -- - \ n ");
Linklist p, q;
P=L;
//I=5;
Int j=0;
While (p - & gt; Next& & j{
P=p - & gt; Next;
j++;
}
If (p - & gt; Next==NULL)//delete position unreasonable
{
Printf (" ERROR! Remove position is unreasonable!" );
exit(0);
}
Q=p - & gt; Next;//delete node
p-> Next=q - & gt; Next;
* the node=q - & gt; data;
Free (q);//release node
Printf (" \ n deleted successfully! \n");
Printf (" \ n the new list is: ");
P=L;
for(i=0; i{
P=p - & gt; Next;
Visit (p);
}
}
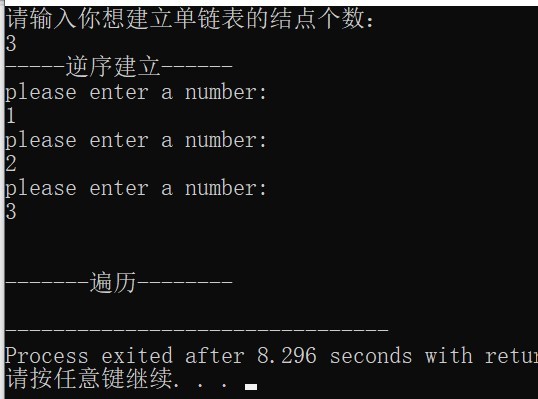
This is the screenshot, the traversal functions start to run and I don't know where there is wrong? Is logically?
Or grammar? (several programs are running half did not move,,,) hope to say about it! Thank you very much!!!!!!!!!!
CodePudding user response:
In VS the breakpoint, single step debugging,CodePudding user response:
# include & lt; stdio.h>
#include
Typedef struct Lnode//define singly linked list storage structure
{
The int data;
Struct Lnode * next;
} Lnode * linklist;
Void CreatList_L (linklist * L, int n);
Void ListTraverse (linklist L, int n);
Void ListInsert (linklist L, int, int, e int n);//n for the node number in the list, I for the ith element inserted before, e is the value of the new node
Void ListDelete (linklist L, int, int * node, int n);//n for the node number in the list, I to delete the node location, e used to return the value of delete node
Void visit linklist (p);
Int main ()
{
Linklist L;
Int n, I=5, e=999, * node;
Printf (" please enter the number you want to build a singly linked list of nodes: \ n ");
The scanf (" % d ", & amp; n);
CreatList_L (& amp; L, n);//reverse singly linked lists, and will be the head of a singly linked list address is assigned to a pointer s
ListTraverse (m, n);//traverse singly linked list
ListInsert (L, I, e, n);//insert
ListDelete (L, I, node, n);//delete
}
Void CreatList_L (linklist * L, int n)//reverse established
{
Printf (" -- -- -- -- -- reverse build -- -- -- -- -- - \ n ");
Linklist p;
int i;
* L=(Lnode *) malloc (sizeof (Lnode));//set up leading the singly linked list of nodes
//L - & gt; Next==NULL;
(* L) - & gt; Next=NULL;
for(i=n; I> 0; I -)
{
P=(Lnode *) malloc (sizeof (Lnode));
Printf (" both please enter a number: \ n ");
The scanf (" % d ", & amp; p-> The data);
p-> Next=(* L) - & gt; Next;
(* L) - & gt; Next=p;
}
}
Void ListTraverse (linklist L, int n)//traverse singly linked list
{
Printf (" \ n \ n -- -- -- -- -- -- -- traversal -- -- -- -- -- -- -- -- \ n ");
int i;
Linklist p;
P=L - & gt; Next;
for(i=0; i{
Visit (p);
P=p - & gt; Next;
}
}
Void visit (linklist p)//output
{
Printf (" % d ", p - & gt; The data);
}
Void ListInsert (linklist L, int, int, e int n)//before the fifth element insert element
{
Printf (" \ n \ n -- -- -- -- -- insert element -- -- -- -- -- - \ n ");
Linklist p, q;
P=L;
//e=999;
//I=5;
Int j=0;
While ((p - & gt; Next) & amp; & j