In the code below, running on macOS 12.4, I'm trying to display a sheet
with a few stacked Text
views. As shown in the screenshot, the last line is not wrapping. Strangely, if I had a padding on the Text
two views above it (see comment in code), that triggers something, and the Text
wraps. This seems bizarre and buggy to me, so I don't think I can rely on it.
Can anyone explain this behavior, and suggest a way to ensure the text wraps instead of truncates?
struct ContentView: View {
@State var showSheet = false
var body: some View {
VStack {
Button("Test Sheet") {
showSheet = true
}
}
.frame(minWidth: 400, minHeight: 300)
.sheet(isPresented: $showSheet) {
TestSheet {
showSheet = false
}
}
}
}
struct TestSheet: View {
let dismiss: () -> Void
var body: some View {
VStack(spacing: 0) {
VStack(alignment: .leading) {
let msg = "Longer message. Blah blah blah blah blah, blah blah blah blah, blah blah blah blah blah blah blah blah, blah blah."
Text("Delete?").font(.title).padding(8).frame(maxWidth: .infinity, alignment: .center)
Text(verbatim: msg)
// .padding(.top, 6) <-- uncomment this and it works. ???
Text("Do you want to delete them?").padding(.top, 6)
Text(verbatim: "They can be undeleted later, until you 'Purge Deleted Items'.")
.lineLimit(nil)
.padding(.top, 6)
}
.padding()
Divider()
HStack {
Button("Cancel", role: .cancel) { dismiss() }
}.padding()
}
.frame(width: 300)
}
}
Screenshot of it not wrapping as desired:
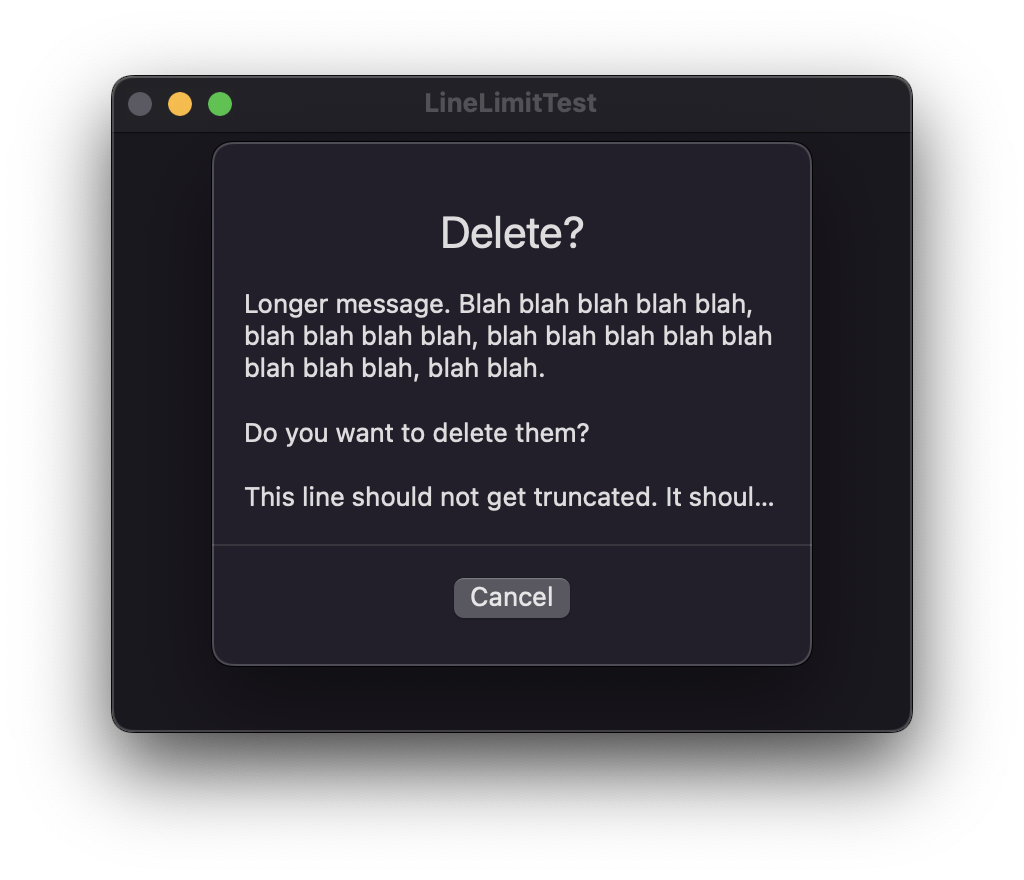
CodePudding user response:
Try tagging the following to the end of your Text
view:
.fixedSize(horizontal: false, vertical: true)
I know it looks counter-intuitive, 'I want my vertical size fixed rather than my horizontal size fixed, don't I?', but the text view gives it's 'ideal' width as its non-wrapped (i.e. single line) width. Therefore, you need to ignore that, but insist on its now wrapped vertical ideal size instead.