Why is the Card
showing extra space at the bottom?
If I set itemCount: 0
I get this:
import 'package:flutter/material.dart';
class TestScreen extends StatelessWidget {
const TestScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
List<String> words = ['hello', 'world', 'flutter'];
return Scaffold(
backgroundColor: Colors.green,
appBar: AppBar(
title: const Text('Test'),
centerTitle: true,
),
body: Card(
child: ListView.separated(
separatorBuilder: (context, index) {
return const Divider(
color: Colors.black,
);
},
itemCount: words.length,
shrinkWrap: true,
itemBuilder: (context, index) {
return ListTile(
title: Text(words[index]),
);
},
),
),
);
}
}
CodePudding user response:
According to ListView documentation, it has padding by default. You can disable this behavior with
ListView(
padding: const EdgeInsets.all(8),
...
Docs here:
/// By default, [ListView] will automatically pad the list's scrollable
/// extremities to avoid partial obstructions indicated by [MediaQuery]'s
/// padding. To avoid this behavior, override with a zero [padding] property.
///
/// {@tool snippet}
/// This example uses the default constructor for [ListView] which takes an
/// explicit [List<Widget>] of children. This [ListView]'s children are made up
/// of [Container]s with [Text].
///
/// 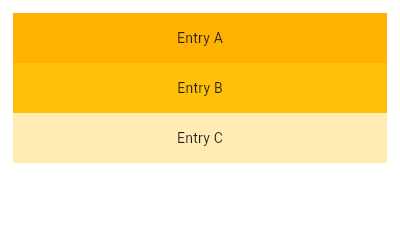
///
/// ```dart
/// ListView(
/// padding: const EdgeInsets.all(8),
/// children: <Widget>[
/// Container(
/// height: 50,
/// color: Colors.amber[600],
/// child: const Center(child: Text('Entry A')),
/// ),
/// Container(
/// height: 50,
/// color: Colors.amber[500],
/// child: const Center(child: Text('Entry B')),
/// ),
/// Container(
/// height: 50,
/// color: Colors.amber[100],
/// child: const Center(child: Text('Entry C')),
/// ),
/// ],
/// )
/// ```