I am new in stack overflow.
I am using Vue3 tailwindcss.
I want to create dyamic grid like this
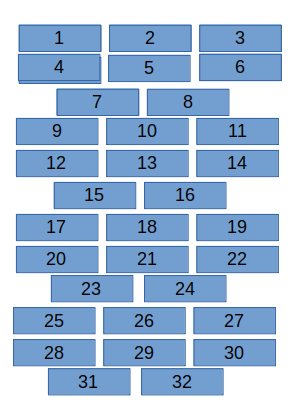
Corrrection: Here i want repetitive layout and the item of loop are dynamic
Here is My code:
<template>
<div >
<div >
<ul >
<template v-for="(i,index) in 8" :key="index">
<li
v-if="updateCounter(index) || (index 1) % 8 != 0"
>
<div > {{ index }} {{updateCounter(index) }}</div>
</li>
<li
v-else
>
<div >{{ index }} </div>
</li>
</template>
</ul>
</div>
</div>
</template>
<script setup lang="ts">
import { ref } from 'vue';
const count = ref<number>(2)
const checkForCols = ref<boolean>(false);
const total = ref<number>(6)
const updateCounter = (index: any) => {
if( total.value == index ){ // 0 = (0 1) , 1 = (1 1) 1, 2 = (2 1) 1, 3 = (3 1) 1,4 = (4 1) 1, 5 = (5 1) 1
total.value = total.value 6 count.value
return true
} else {
return false
}
}
</script>
But i get Wrong output.
I already spend 2 day but i can't find any solution.
So can anyone help me to how i do this?
Thank you in advance.
CodePudding user response:
What really complicates your solution is you're modifying the state while calculating the layout (in the v-for
loop). Never do that!
When you change the value of state, everything that uses that piece of reactive state gets recalculated (including the items that have already been looped through).
It's an anti-pattern, for a couple of reasons:
- it often leads to performance issues (changes triggering each other endlessly)
- it's particularly difficult to follow the mutations trail, while trying to debug it and everyone, including yourself, will have a hard time debugging it, should it start failing.
The fact you asked the question is proof to it. I'm confident you'd have figured out the function below by yourself, had you not modified the state inside the loop.
What you need is:
- the
count
- a function returning
md:col-span-${x}
correctly for the last items based oncount
andindex
, along these lines:
const count = ref(8)
const getMdClass = (index) => {
const rest = count.value % 3;
if (index > count.value - rest - 1) {
return `md:col-span-${12 / rest}`;
}
return "md:col-span-4";
}
This function returns md:col-span-4
all the time, except:
md:col-span-6
for the last 2 items with a(3 x n) 2
count,md:col-span-12
for the last item with a(3 x n) 1
count.
Here's a proof of concept (haven't used typescript or <script setup>
, but the logic is there). I made count
a prop so it could be interacted with.
Note: the function is slightly modified in the sandbox because I've used v-for="n in count"
, which is 1
-based, not 0
-based.