I would like to realize a console window like the console window in VS Code.
In VS Code, when we click Shift Command Y, a console window is opened below the code window. There are several features:
- The whole window is divided into 2 parts. Both the code window and the console window can have their scroller on the right.
- The appearance of the console window resizes the code window.
- There is a horizontal splitter that could resize the console window (and the code window).
I tried to create a codesandbox. But it doesn't have Feature 2 and Feature 3.
Could anyone help?
import { useState } from "react";
import { Button } from "@material-ui/core";
import "./styles.css";
export default function App() {
const [textState, setTextState] = useState("off");
const toggleText = () => {
setTextState((state) => (state === "On" ? "Off" : "On"));
};
return (
<>
<Button variant="contained" color="primary" onClick={toggleText}>
Toggle
</Button>
<div style={{ overflowY: "scroll", height: "300px", fontSize: "30px" }}>
<h1>code 1</h1>
<h1>code 2</h1>
<h1>code 3</h1>
</div>
<div>
{textState === "On" && (
<div
style={{ overflowY: "scroll", height: "200px", fontSize: "30px" }}
>
<h1>console 1</h1>
<h1>console 2</h1>
<h1>console 3</h1>
</div>
)}
</div>
</>
);
}
CodePudding user response:
For the 2nd question, you can set the code
area height to full height when console
view is hidden.
height: `${textState === "On" ? 500 - consoleAreaHeight : 500}px`
For the 3rd question, It could be achieved using a ref
and a mouse move handler.
const consoleRef = useRef(null);
const mouseMoveHandler = (e) => {
e.preventDefault();
if (resizing) {
const detla = consoleRef.current.getBoundingClientRect().top - e.clientY;
setConsoleAreaHeight(
(prevConsoleAreaHeight) => prevConsoleAreaHeight detla
);
}
};
Code sandbox => https://codesandbox.io/s/react-material-togglebuttonexample-forked-dk7lu?file=/src/App.jsx
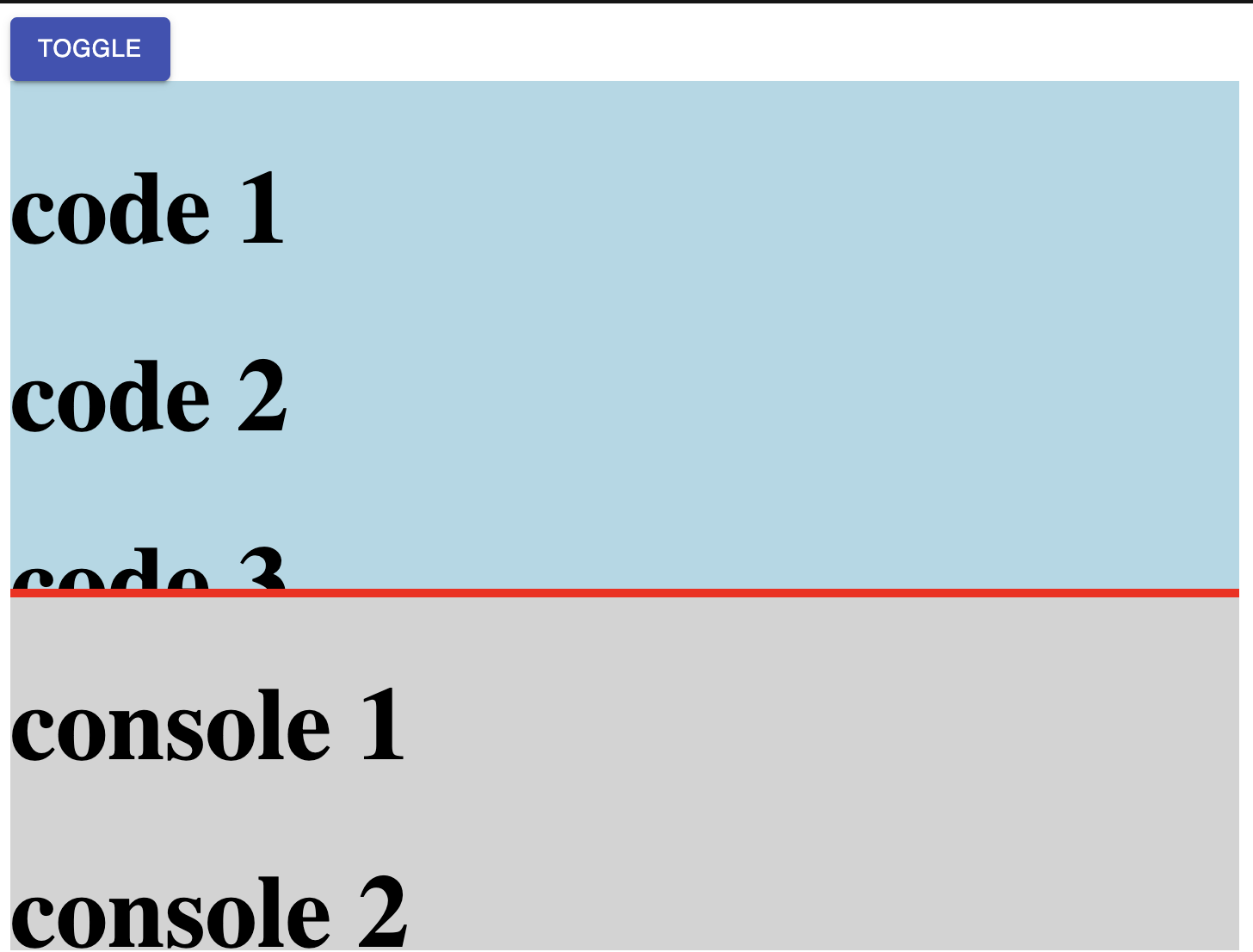