I have an issue with QML PathPolyLine "path" property. In documentation it said that:
It can be a JS array of points constructed with Qt.point()
Does it mean I can work with it like with JS array?
Here is an example that shows that .push() doesn't work for it "path" property, but I can set a custom array and then appoint my custom array to "path" property of PathPolyLine element.
So what is the correct way of editing this property?
Also setting alias to this property like this property alias myArray: PathPolyLineID.path
to work with "myArray" not affecting ".path" too.
main.qml
import QtQuick
import QtQuick.Controls
Window {
id: mainWinID
width: 640
height: 480
visible: true
title: qsTr("Hello World")
property var points: []
Button
{
property int i: 0
id: btnID
text: "Click Me!"
onClicked:
{
if (i == 2) pplID.path = mainWinID.points;
pplID.path.push(Qt.point(i,i 10));
mainWinID.points.push(Qt.point(i,i 10));
console.log('pplID.path:', pplID.path);
console.log('mainWinID.points:', mainWinID.points);
i ;
}
}
PathPolyline
{
id: pplID
path: []
}
}
Outcome is such:
qml: pplID.path: []
qml: mainWinID.points: [QPointF(0, 10)]
qml: pplID.path: []
qml: mainWinID.points: [QPointF(0, 10),QPointF(1, 11)]
qml: pplID.path: [QPointF(0, 10),QPointF(1, 11)]
qml: mainWinID.points: [QPointF(0, 10),QPointF(1, 11),QPointF(2, 12)]
qml: pplID.path: [QPointF(0, 10),QPointF(1, 11)]
qml: mainWinID.points: [QPointF(0, 10),QPointF(1, 11),QPointF(2, 12),QPointF(3, 13)]
CodePudding user response:
There isn't any secrets, really, just initialize a new JS array and push()
items based on Qt.point()
.
It's easier to test if it's working or not using a valid data range and displaying it for visualization purposes:
import QtQuick 2.15
import QtQuick.Window 2.15
import QtQuick.Controls 2.15
import QtQuick.Shapes 1.15
Window {
id: mainWinID
width: 640
height: 480
visible: true
property var points: [ ]
Button {
id: btnID
text: "Click Me!"
onClicked: {
// clear the JS array to delete existing points
mainWinID.points = [ ]
// initialize the JS array with data points
mainWinID.points.push(Qt.point(320, 240));
mainWinID.points.push(Qt.point(400, 360));
mainWinID.points.push(Qt.point(260, 360));
mainWinID.points.push(Qt.point(320, 240));
console.log('mainWinID.points=', mainWinID.points)
// use the array as the path for PathPolyline
pplID.path = mainWinID.points;
}
}
Shape {
anchors.fill: parent
anchors.centerIn: parent
ShapePath {
strokeWidth: 3
strokeColor: "black"
fillColor: "orange"
fillRule: ShapePath.OddEvenFill
PathPolyline {
id: pplID
}
}
}
}
Using this code, when the button is clicked an orange triangle is rendered:
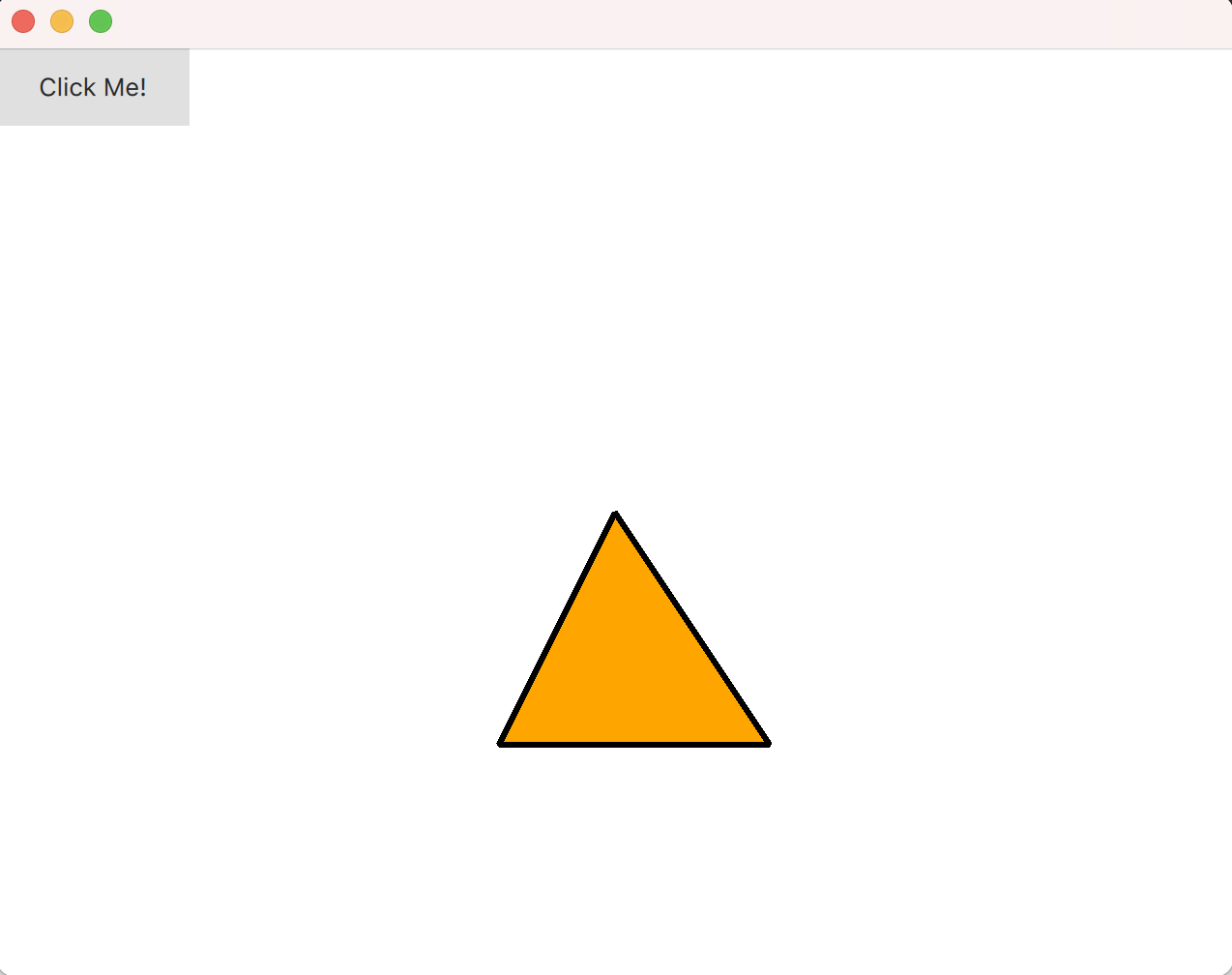
However, you can't push elements into the default array used by PathPolyline
. The best you can do in this case is initialize the entire array at once like this:
onClicked: {
// initialize PathPolyline.path
pplID.path = [
Qt.point(320, 240),
Qt.point(400, 360),
Qt.point(260, 360),
Qt.point(320, 240)
]
console.log('pplID.path=', pplID.path);
}