I'm creating a random word generator in Next.js. I'm managing the state of the three randomly generated words with useState
. However, React gives me an error message when I write like this:
const [word1, setWord1] = React.useState(generateWord());
Error message:
Unhandled Runtime Error
Error: Text content does not match server-rendered HTML.
See more info here: https://nextjs.org/docs/messages/react-hydration-error
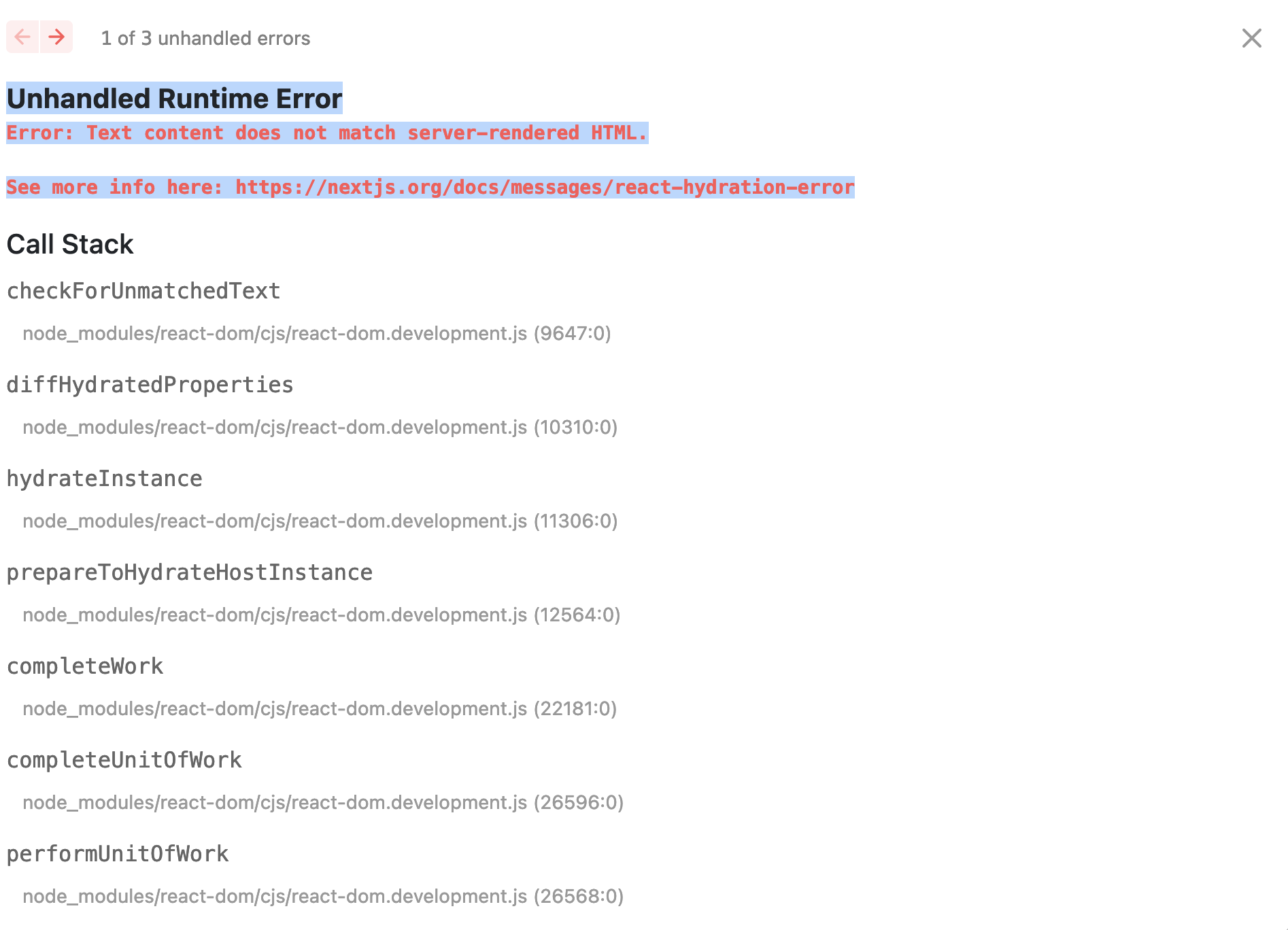
Why is this error happening? How do I write the above code properly?
I'm assuming this issue can be mitigated by using useEffect
, which runs in component lifecycle timings like componentDidMount
and componentDidUpdate
. However, I only want to set the initial values upon loading the page (only componentDidMount
). (The text fields will get new randomly generated words once the user clicks a button.)
CodePudding user response:
The mismatch happens because useState is run during SSR and so the server generates a random word but when the page is rehydrated on the client side a different random word is generated. This mismatch causes your React app to warn you about this inconsistency.
To remedy this, you can use an empty string in the useState hook, and then update the state with a randomly generated word in a useEffect hook with an empty dependency array, which only runs on the client side when your component is mounted:
const [word1, setWord1] = React.useState('');
// Only runs on the client
React.useEffect(() => {
setWord1(generateWord());
}, []);