I'm looking for help with finding the coordinates (CGPoint) of the green dot given a circle like on the picture below.
I know the radius, theta, the coordinates of the red point and that I'd like the distance between the red and green points to be 25.
I'd appreciate any help - thank you!
CodePudding user response:
What you need is:
Polar to Cartesian conversion
To find the coordinates of a point given the radius & angle, we can use the following:
x = r × cos(θ)
y = r × sin(θ)
Important Note: iOS & macOS calculate angles clock-wise, starting from the right hand side (well, AppKit uses a coordinate system that's flipped in the Y axis, but that is a different story). To compensate for this, our whole canvas is rotated by 90°
and flipped in the X axis (to make it counter clock-wise and match your requirements):
Calculate the existing point:
struct ContentView: View {
let radius: Double = 100
var body: some View {
ZStack {
Circle()
.stroke()
.frame(width: radius * 2, height: radius * 2)
Circle()
.frame(width: 10, height: 10)
.offset(x: cos(Angle(degrees: 45).radians) * radius,
y: sin(Angle(degrees: 45).radians) * radius)
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
.rotationEffect(.radians(.pi / 2))
.scaleEffect(x: -1, y: 1)
}
}
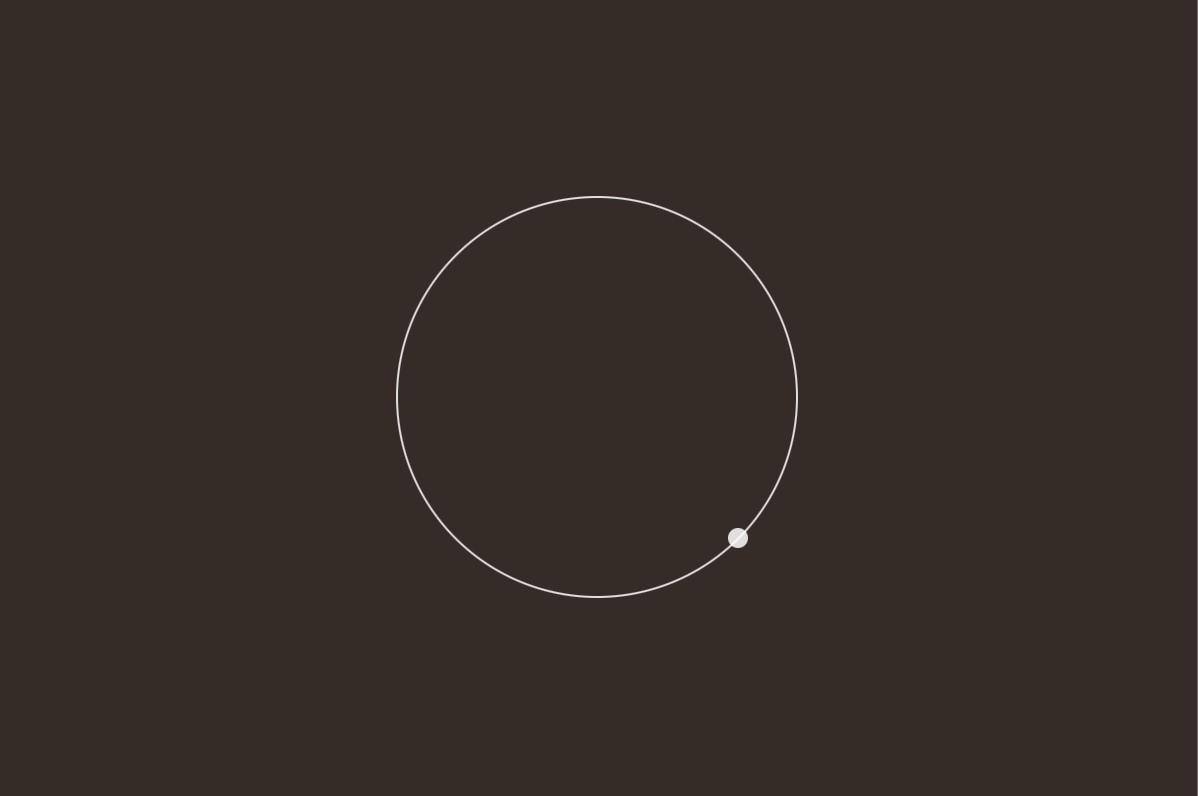
now, given that you want a 25
points smaller circle, the new radius is 75
(100 - 25).
Just so we can visualize it, here it is:
Circle()
.stroke()
.frame(width: (radius - 25) * 2, height: (radius - 25) * 2)
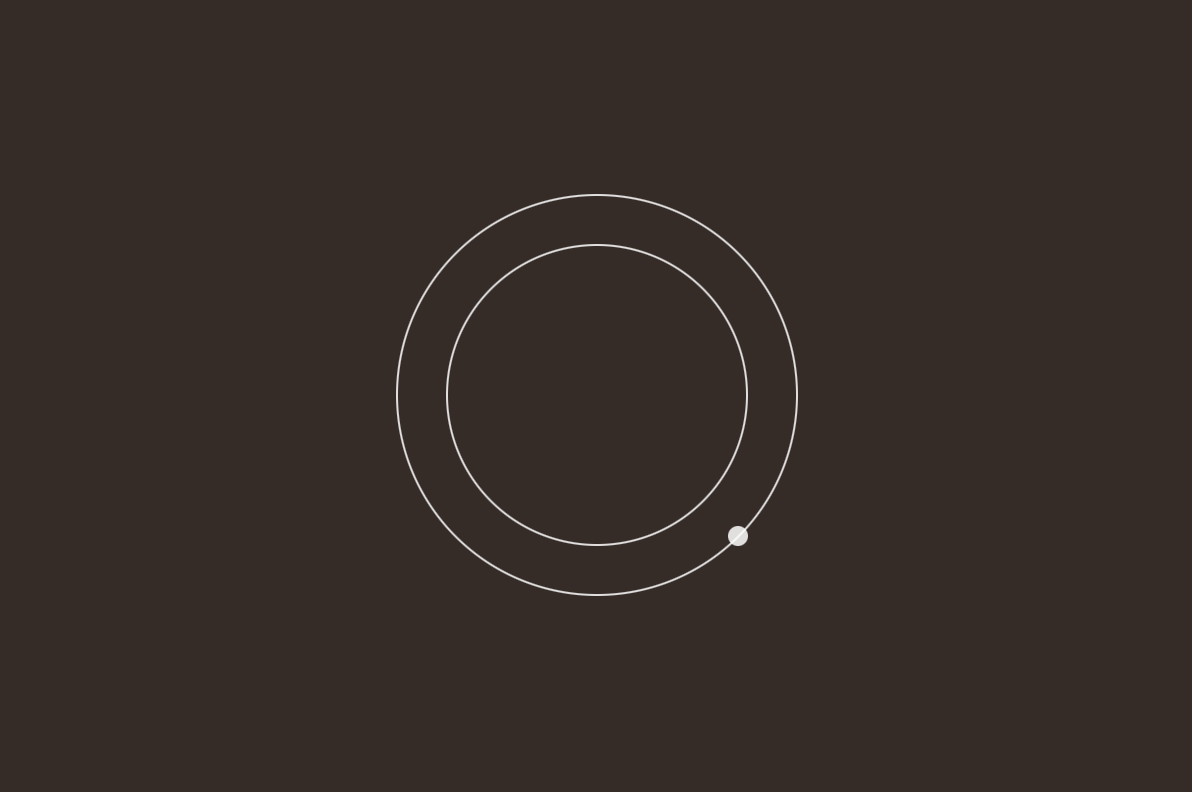
so it's now quite trivial to calculate any other point. In our case we want a point at 45°
at a radius of 75
:
Circle()
.frame(width: 10, height: 10)
.offset(x: cos(Angle(degrees: 45).radians) * 75,
y: sin(Angle(degrees: 45).radians) * 75)
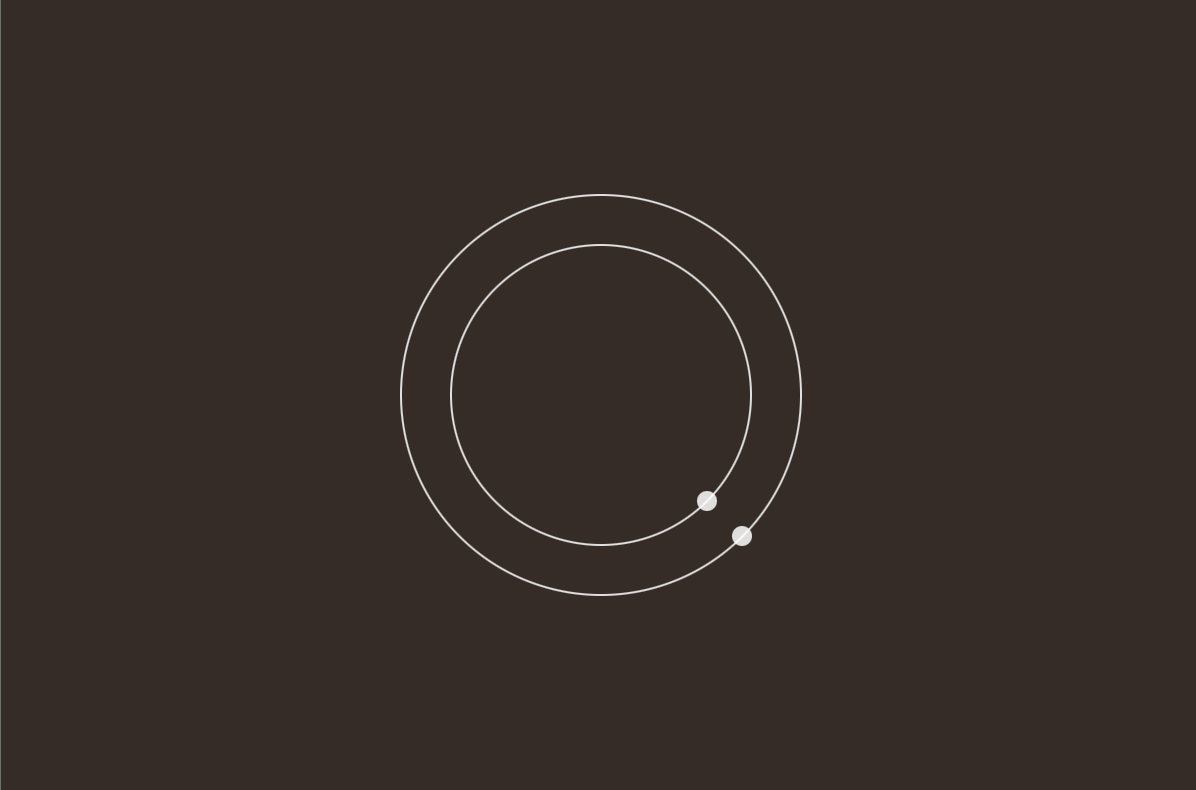
The code of course is just for visualization purposes, the main point is the Polar to Cartesian conversion.
I hope that this makes sense.
CodePudding user response:
Assuming theta is between 0 and 90 degrees (and is in the bottom right quarter) -- with theta defined how you have on that picture.
import UIKit
let redDot = CGPoint(x:70, y:71)
let theta = 45 * CGFloat.pi / 180.0
let redGreenDist: CGFloat = 25
let greenDot = CGPoint(x: redDot.x - sin(theta)*redGreenDist, y: redDot.y - cos(theta)*redGreenDist)
Note that you need to use radians and that the radius of the circle doesn't matter (or even that there is a circle). All that matters is the distance between the points and the angle.