I'm trying to merge two bitmaps 640 pixel wide each, but the result is always a 1024 wide bitmap. How can i get android to create a 1280 wide bitmap?
I'm using the code below for scaling my bitmaps:
public static Bitmap scaleWidthImage(Bitmap image, int destWidth) {
int origWidth = image.getWidth();
int origHeight = image.getHeight();
int destHeight;
if (origWidth > destWidth) {
destHeight = origHeight / (origWidth / destWidth);
} else {
destHeight = origHeight * (destWidth / origWidth);
}
image = Bitmap.createScaledBitmap(image, destWidth, destHeight, false);
return image;
}
And this code for mergin both bitmaps in one
fun mergeBitmap(fr: Bitmap, sc: Bitmap): Bitmap? {
val comboBitmap: Bitmap
val width: Int = fr.width sc.width
val height: Int = fr.height
comboBitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888)
val comboImage = Canvas(comboBitmap)
comboImage.drawBitmap(fr, 0f, 0f, null)
comboImage.drawBitmap(sc, fr.width.toFloat(), 0f, null)
return comboBitmap
}
When the widths of both bitmaps are less than 1024 pixels, the merged bitmap has the desired width, but when the sum of both is greater than 1024, it does not.
CodePudding user response:
I've tried with the two images below :
Image 640x640 :
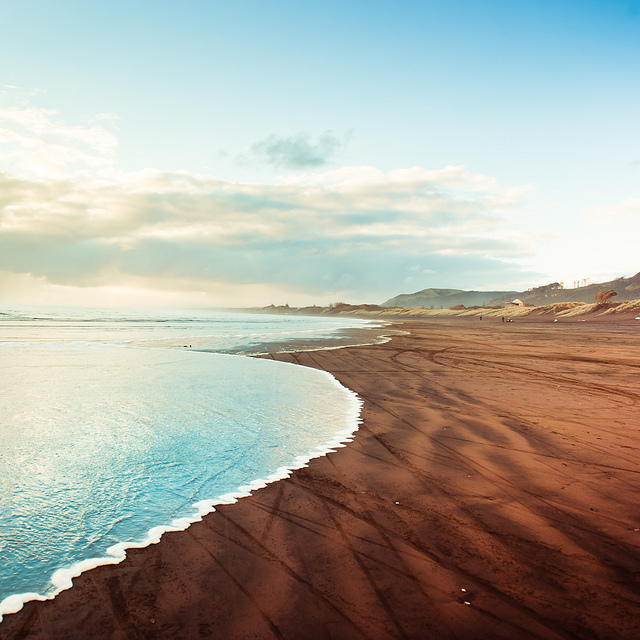
Image 640x800 :
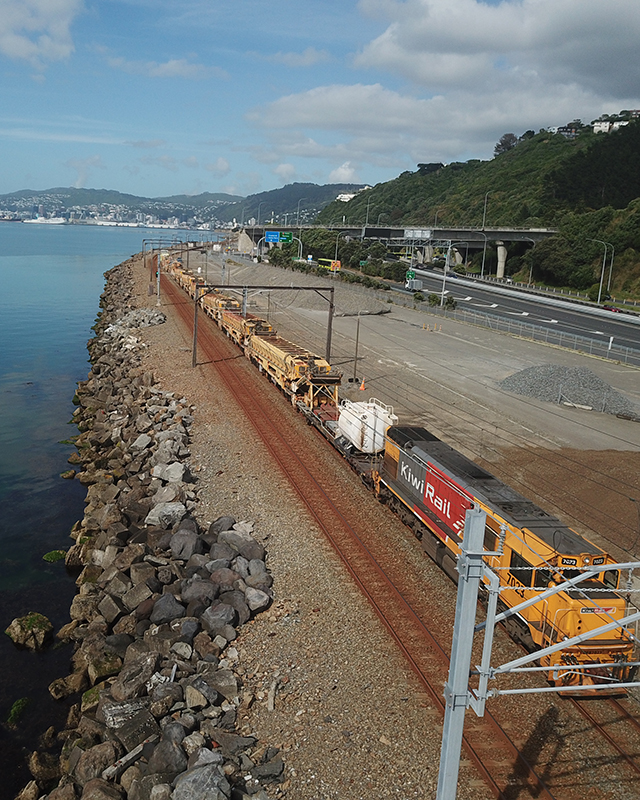
I've made this method to merge the two bitmaps into one:
public static Bitmap drawBitmapsHorizontally(Bitmap bitmapOne, Bitmap bitmapTwo) {
ArrayList<Bitmap> bitmaps = new ArrayList<>();
bitmaps.add(bitmapOne);
bitmaps.add(bitmapTwo);
int width = 0;
for (Bitmap map : bitmaps)
width = map.getWidth();
// you can set your favorite height (e.g. 720) instead of getting the max height of the two bitmaps.
Bitmap bitmap = Bitmap.createBitmap(width,
Math.max(bitmapOne.getHeight(),bitmapTwo.getHeight()),
Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
canvas.setDensity(DisplayMetrics.DENSITY_MEDIUM);
bitmap.setDensity(DisplayMetrics.DENSITY_MEDIUM);
canvas.drawBitmap(bitmapOne, 0f, 0f, null);
canvas.drawBitmap(bitmapTwo, bitmapOne.getWidth(), 0f, null);
return bitmap;
}
Result 1280x800 :
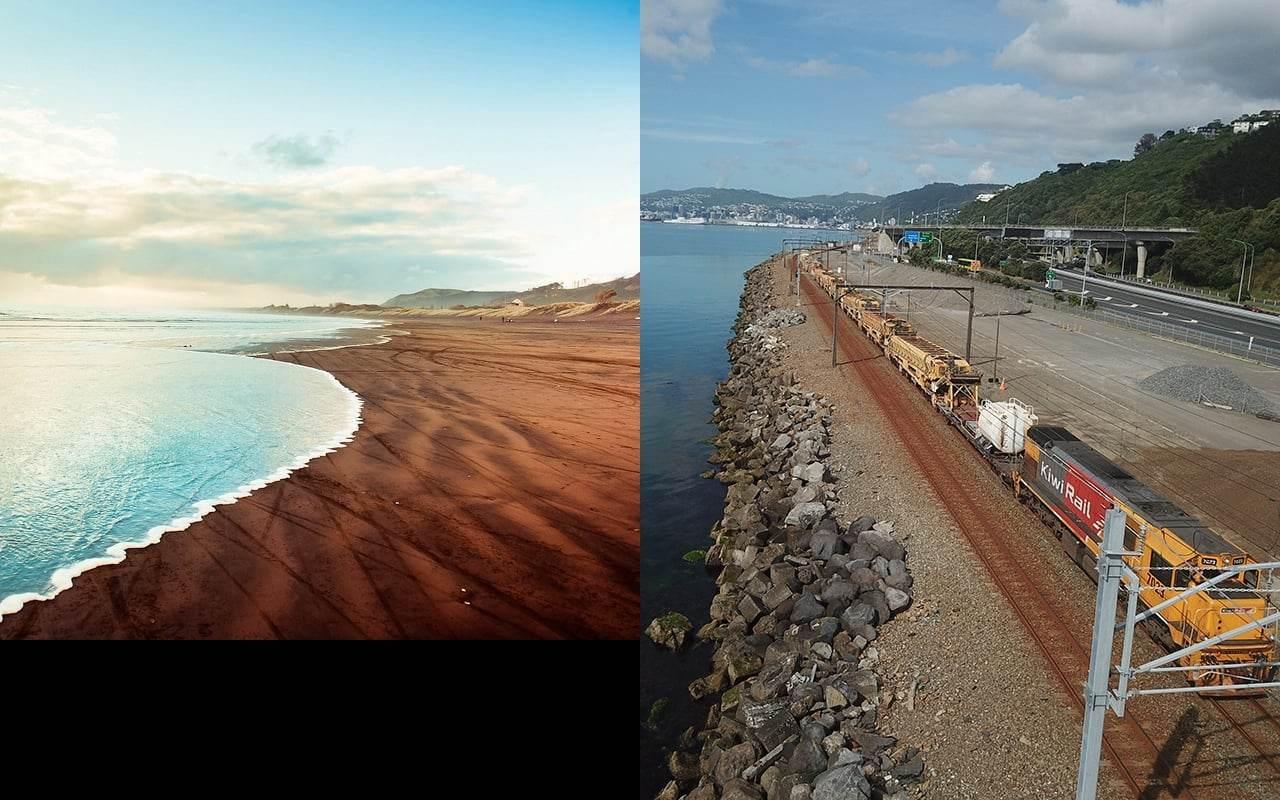