When i change the urls in my App.js
file the component does not render anymore, so if I go to the new url I get an error as if the page does not exist but if I go to the old url it loads the navbar which is in the parent component but not the component in the route
App.js
import React, { Component } from "react";
import { BrowserRouter, Route, Switch } from "react-router-dom";
import "antd/dist/antd.css";
import MainPage from "./mainpage";
import Details from "./Detail";
import SignUp from "../containers/Signup";
import AddItem from "./AddItem";
import Admin from "../containers/admin";
import CustomLayout from "../containers/Layout";
class App extends Component {
render() {
return (
<BrowserRouter>
<CustomLayout {...this.props}>
<Switch>
<Route exact path="/" component={MainPage} />
<Route exact path="/signup" component={SignUp} />
<Route exact path="/Add-Item" component={AddItem} />
<Route exact path="/admin" component={Admin} />
<Route exact path="/post/:id" component={Details} />
</Switch>
</CustomLayout>
</BrowserRouter>
);
}
}
export default App;
index.js
const store = createStore(reducers, {}, compose(applyMiddleware(thunk)));
const app = (
<Provider store={store}>
<App />
</Provider>
);
CodePudding user response:
You missed Switch tag, below code will work fine:
class App extends Component {
render() {
return (
<BrowserRouter>
<CustomLayout {...this.props}>
<Switch>
<Route exact path="/" component={mainpage} />
<Route exact path="/signup" component={Signup} />
<Route exact path="/Add-Item" component={AddItem} />
<Route exact path="/admin" component={admin} />
<Route exact path="/post/:id" component={Details} />
</Switch >
</CustomLayout>
</BrowserRouter>
);
}
}
CodePudding user response:
Here is an example about what i mean in the comment, just add Switch wrapping the routes
<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/users">
<Users />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
CodePudding user response:
Add the Switch component like everyone is suggesting, get the components you're mounting to the Route are named and imported in Pascal case(Eg: MainPage). If these doesn't work can you paste the code dump of App.js file? (including imports)
Update after code inspection: I changed one route URL to reproduce the issue, "/signup" to "/signupnew". You are right, after changing the URL, if you visit old one(which doesn't exist anymore) you will just see Layout wrapper(which is navbar and footer). This behavior is understandable as no matching route was found pointing to that path and no fallback route was specified to mount 404 component. this explains component not rendering on old path. On new URL/path I was able to render the component assigned to it.
Change I Made in the code
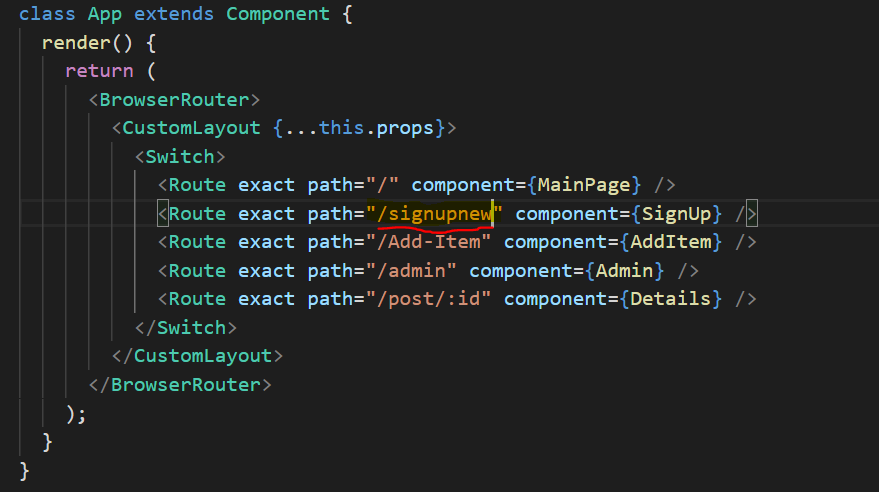
Old Path
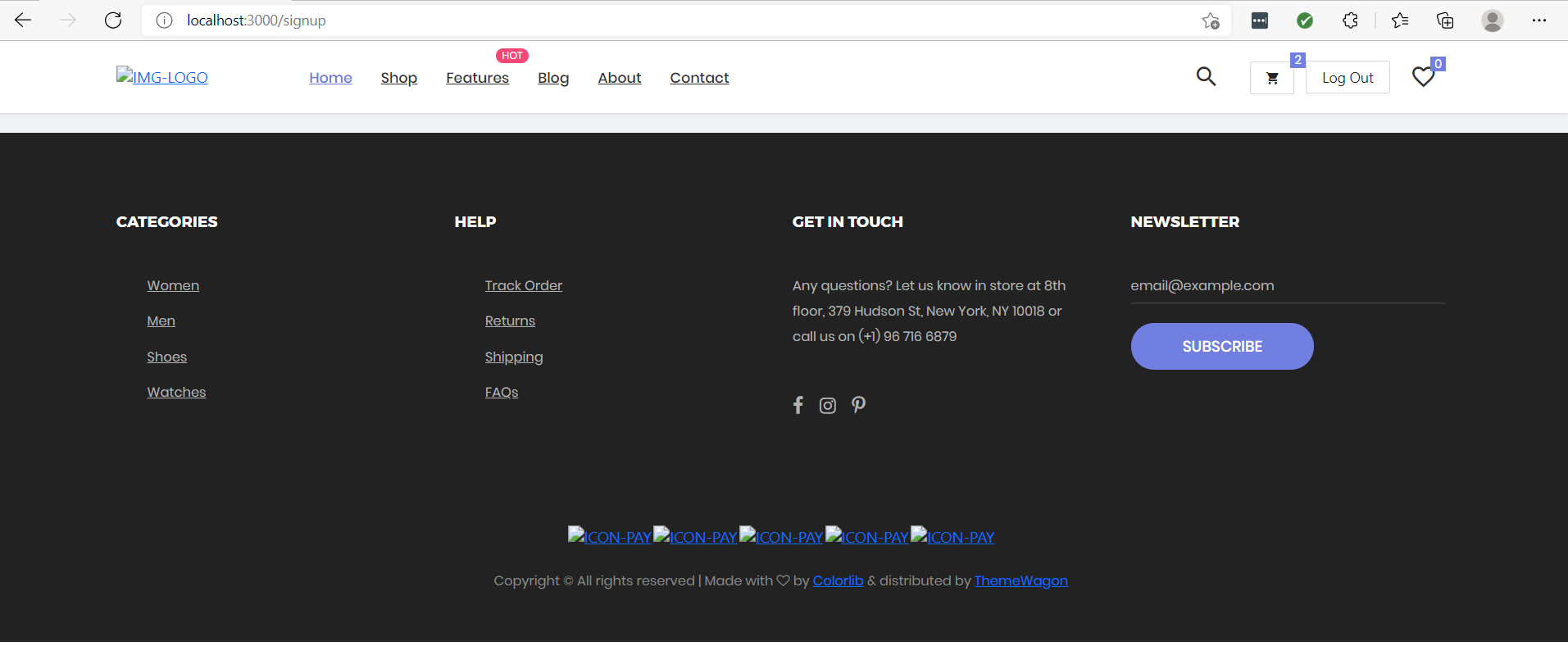
New Path
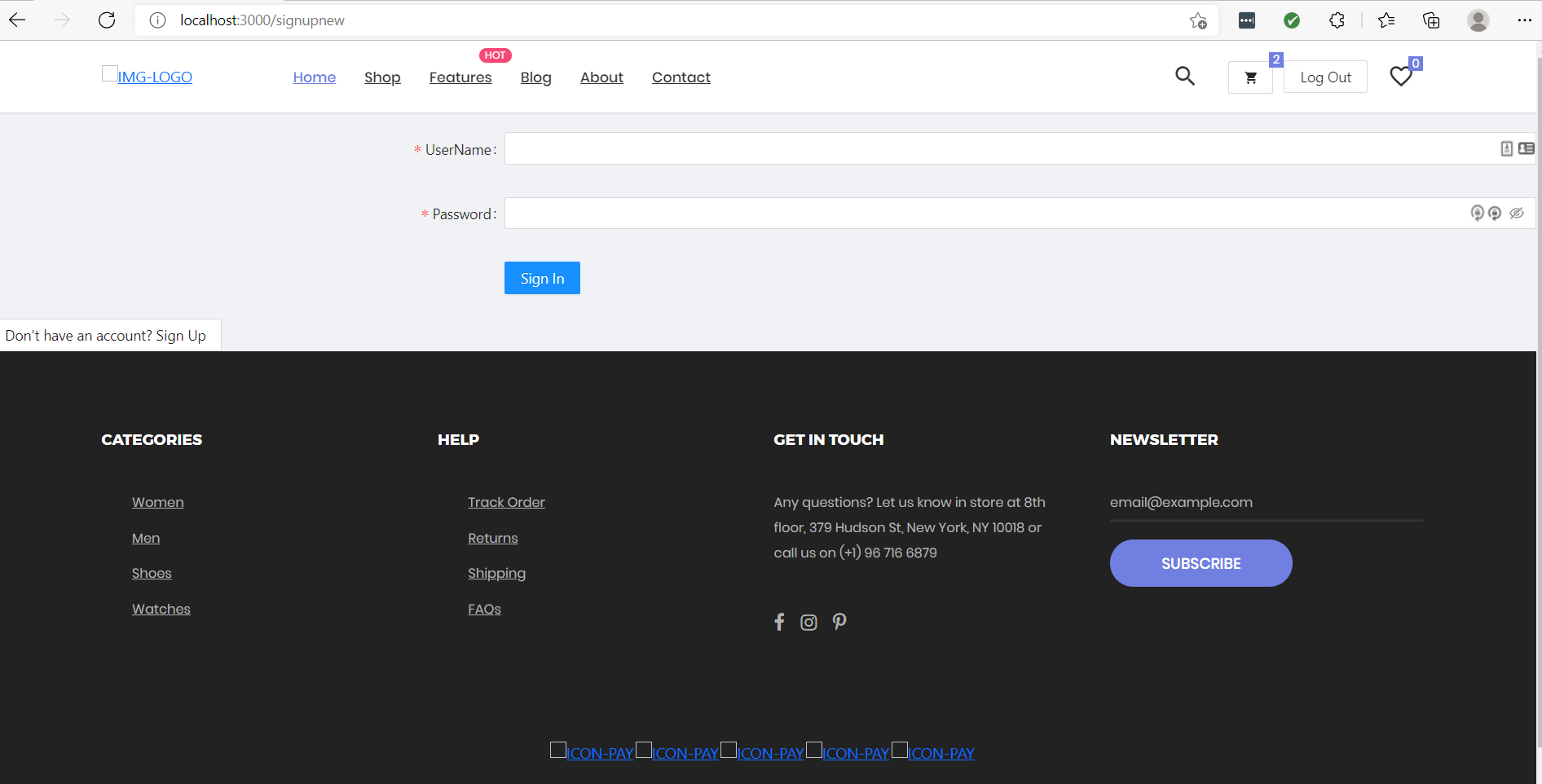
Try to restart the react server and access new URL you changed in app.js file. Or if you changed the URL differently compared to what I did, Let me know.
CodePudding user response:
Use Switch and change BrowserRouter to Router.
class App extends Component {
render() {
return (
<Router>
<CustomLayout {...this.props}>
<Switch>
<Route exact path="/" component={mainpage} />
<Route exact path="/signup" component={Signup} />
<Route exact path="/Add-Item" component={AddItem} />
<Route exact path="/admin" component={admin} />
<Route exact path="/post/:id" component={Details} />
</Switch >
</CustomLayout>
</Router>
);
}
}
Also, you can try adding History; history.createBrowserHistory . . . to the router to see if it's working.
And then you can just to clickEvents to change path, so import history: the history.push('/path')