My card's text part has multiple text widgets that I have to put under column
. I want to add this whole part under a row
widget, so that I can add the image part to the right of this column widget.
This is what I've done:
class ContactMe extends StatelessWidget {
static const String route = '/contact_me';
const ContactMe({Key? key}) : super(key: key);
Widget build(BuildContext context) {
double width = MediaQuery.of(context).size.width;
double height = MediaQuery.of(context).size.height;
return Scaffold(
body: Column(
children: [
CommonNavBar(height: height),
Expanded(
child: FutureBuilder(
future: contactMe(),
builder: (context, snapshot) {
if (snapshot.data == null)
return Center(child: CircularProgressIndicator());
else {
var data = snapshot.data as List<String>;
return LayoutBuilder(builder: (context, constraints) {
if (constraints.maxWidth < 1000) {
return Center();
} else {
return Row( //this is parent row
children: [
Container(
child: Column( //the column that contains multiple other widgets
children: [
text('Reach Out to me!', 25,
Theme.of(context).primaryColorLight),
Text('anything'),
],
),
),
Image.asset('assets/contact_me/' data[2], scale: 2), //the image widget
],
);
}
});
}
}),
),
],
),
);
}
}
But instead of a card, the layout looks like this:
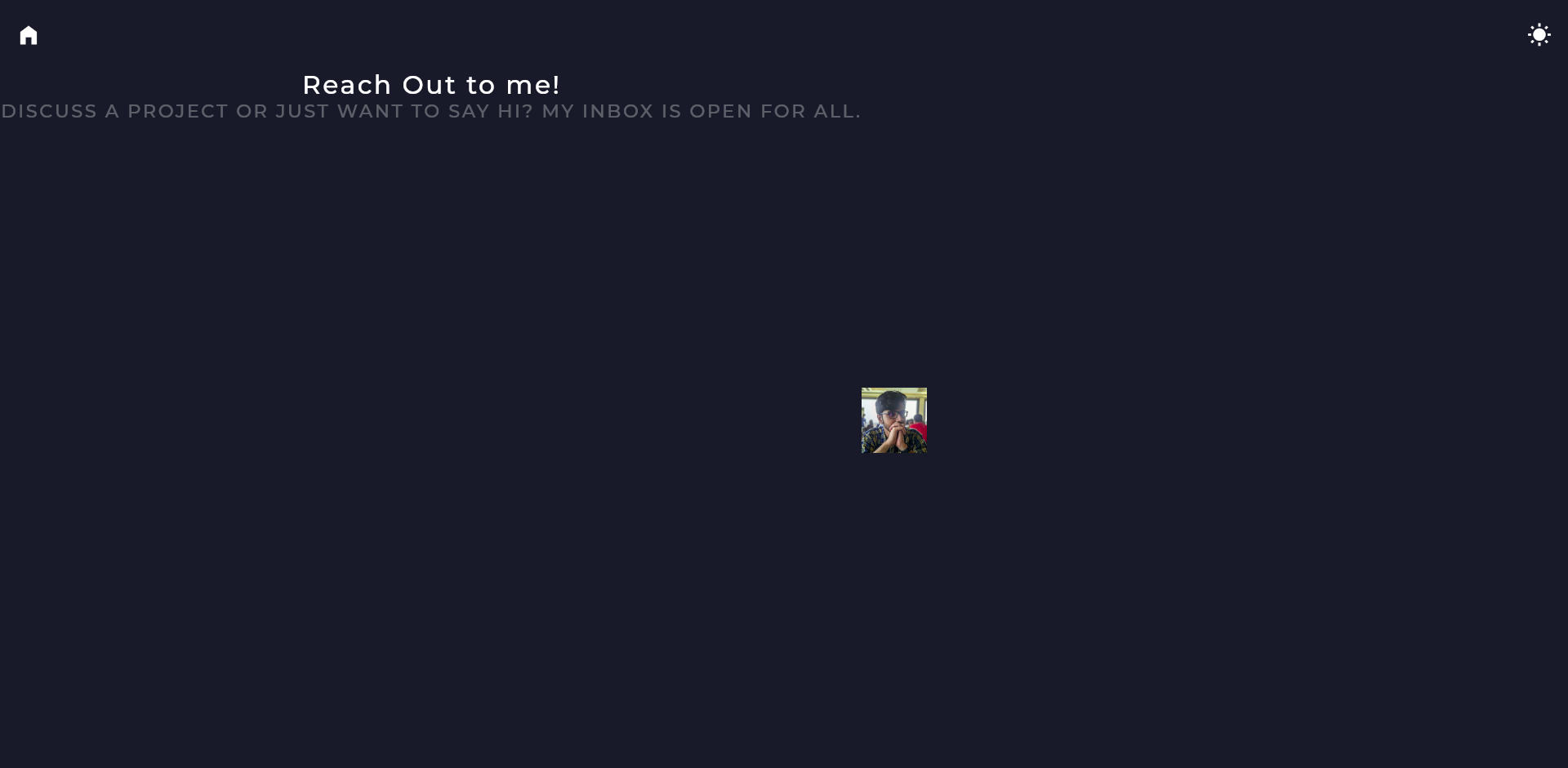
As visible in the picture, the image for some reason is not in line with the column widget. What's the mistake I'm making here?
CodePudding user response:
I think your problem is related to different alignments. The default crossAxisAlignment
for a Row is CrossAxisAlignment.center
, thus your image and column are located in the vertical middle of the row. The default mainAxisAlignment
for a Column is MainAxisAlignment.start
, so the content inside your column is located at its top.
I can see two possible ways to put your content on the same horizontal line:
- Set
mainAxisAlignment
for the Column toMainAxisAlignment.center
- Set
crossAxisAlignment
for the Row toCrossAxisAlignment.start
For the future, you might find this resource helpful when dealing with different layouts: https://docs.flutter.dev/development/ui/layout
CodePudding user response:
In continuation to @OlegBezr's answer, I fixed it by using mainAxisAlignment
and crossAxisAlignment
for both the widgets.
return Row(
crossAxisAlignment: CrossAxisAlignment.start, //takes the row to the top
mainAxisAlignment: MainAxisAlignment.spaceAround, //Used this for spacing between the children
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.start, //used for aligning the children vertically
children: [
text('Reach Out to me!', 25,
Theme.of(context).primaryColorLight),
text(
'DISCUSS A PROJECT OR JUST WANT TO SAY HI? MY INBOX IS OPEN FOR ALL.',
18,
Theme.of(context)
.primaryColorLight
.withOpacity(0.3)),
],
),
Image.asset('assets/contact_me/' data[2], scale: 2),
],
);
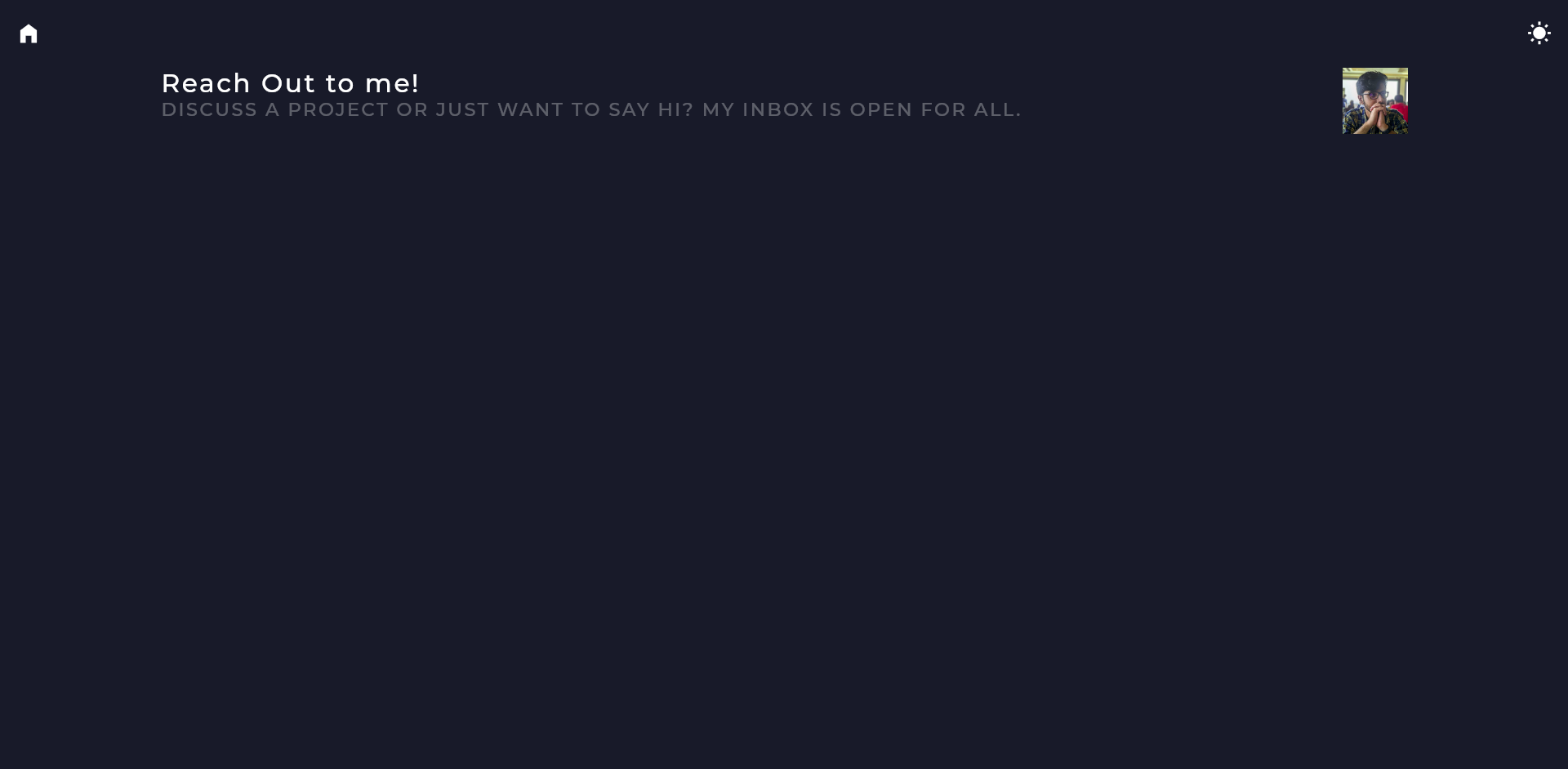