I'm trying to add "quick search buttons" to my flutter app like (in red):
I'm not sure if this is a material design component - if it is, I was hoping to identify the name so that I can e.g. search https://pub.dev/ for an existing widget.
- Does anyone know what this material design component is called (if applicable)?
- How can I implement it/does an existing widget exist on e.g. https://pub.dev/?
Many thanks! :)
CodePudding user response:
Those are called chips, refer to this page https://material.io/components/chips. There is also a section of how to implement these in flutter.
CodePudding user response:
you can use FilterChip
widget for implementing "quick search buttons"
https://api.flutter.dev/flutter/material/FilterChip-class.html
or you can also create your custom widget for achieving this UI
import 'package:flutter/material.dart';
class MyFilterChip extends StatefulWidget {
const MyFilterChip({Key? key}) : super(key: key);
@override
State<MyFilterChip> createState() => _MyFilterChipState();
}
class _MyFilterChipState extends State<MyFilterChip> {
List fruits = ['apple', 'banana', 'mango', 'papaya', 'orange', 'guava'];
int selectedIndex = -1;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Column(
children: [
Padding(
padding: const EdgeInsets.all(15),
child: SizedBox(
height: 30,
child: ListView.separated(
itemCount: fruits.length,
scrollDirection: Axis.horizontal,
itemBuilder: (context, index) => FilterChip(
label: Text(fruits[index]),
selected: index == selectedIndex,
onSelected: (value) {
setState(() {
selectedIndex = index;
});
},
),
separatorBuilder: (BuildContext context, int index) =>
const SizedBox(
width: 10,
),
),
),
),
],
),
);
}
}
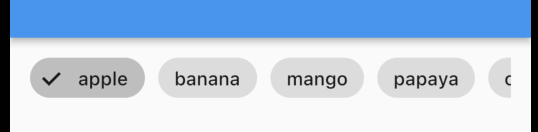