Currently i am implementing the DatePicker where i am selecting the initial date and then passing that date to another date picker which will give me the only dates that are weekly(day after 7 days) or monthly(Day after 30 days) based on the selected date. But it seems I am not getting it I Messing it some where.
Adding a sample code for the reference.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
DateTime selectedDate = DateTime(2020, 1, 14);
// DatePicker will call this function on every day and expect
// a bool output. If it's true, it will draw that day as "enabled"
// and that day will be selectable and vice versa.
bool _predicate(DateTime day) {
print(day);
if ((day.isAfter(selectedDate.subtract(Duration(days: 1))) &&
day.isBefore(selectedDate.add(Duration(days: 7))))) {
return true;
}
return false;
}
Future<void> _selectDate(BuildContext context) async {
final DateTime? picked = await showDatePicker(
context: context,
initialDate: selectedDate,
selectableDayPredicate: _predicate,
firstDate: DateTime(2019),
lastDate: DateTime(2021),
builder: (context, child) {
return Theme(
data: ThemeData(
primaryColor: Colors.orangeAccent,
disabledColor: Colors.brown,
textTheme: TextTheme(bodyText1: TextStyle(color: Colors.blueAccent)),
accentColor: Colors.yellow),
child: child!,
);
});
if (picked != null && picked != selectedDate)
setState(() {
selectedDate = picked;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text("${selectedDate.toLocal()}".split(' ')[0]),
SizedBox(
height: 20.0,
),
RaisedButton(
onPressed: () => _selectDate(context),
child: Text('Select date'),
),
],
),
),
);
}
}
Current Result :
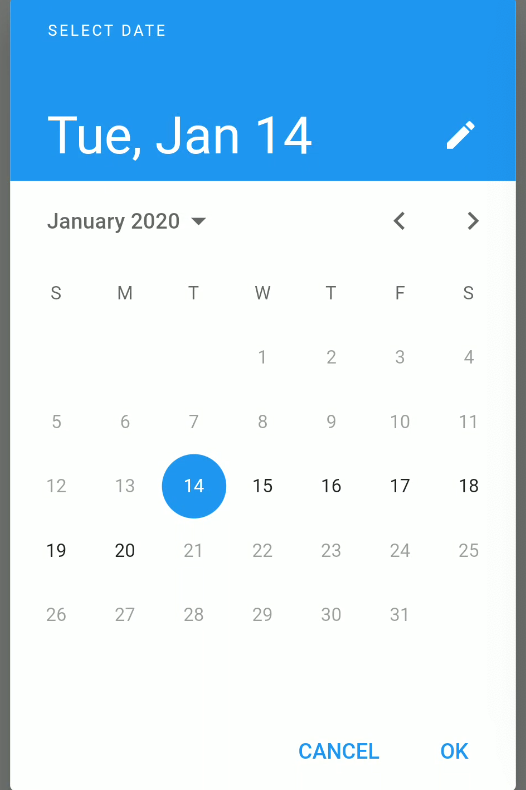
Can any one guide any better resource for this one or suggest if any one has worked on this one.
CodePudding user response:
void _datePicker() {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2019),
lastDate: DateTime.now(),
).then((value) {
if (value == null) {
return;
}
setState(() {
_selectedDate = value;
});
});
}
And Where ever you want to use this
Container(
height: 70,
child: Row(
children: <Widget>[
FlatButton(
textColor: Theme.of(context).primaryColor,
onPressed: _datePicker,
child: Text(
'Choose Date',
style: TextStyle(fontWeight: FontWeight.bold),
),
),
],
),
),
this should solve your issue SO you just need to add initial and last date.
CodePudding user response:
From my understanding, what you want is to only enable dates in interval of weekly or monthly(?) For example, your current initialDate is 14/01/2020, so it should only enable
- weekly: 21/01/2020, 28/01/2020 and so on.
- Monthly: 13/02/2020 (because 31 days for January).
bool _predicate(DateTime day) {
print(day);
if (selectedDate.difference(day).inDays == 7 ||
selectedDate.difference(day).inDays == 30) {
return true;
}
return false;
}
Please recheck if any errors cause I did not run this directly.