I'm trying to follow the Cloudinary documentation to get started with the React SDK. I've already uploaded an image and now I want to retrieve and edit it. I'm stuck on the retrieval bit. I've replicated the tutorial as per:
// Create a Cloudinary instance and set your cloud name.
const cld = new Cloudinary({
cloud: {
cloudName: myCloudName,
},
});
// Use the image with public ID, 'front_face'.
const myImage = cld.image("samples/cld-sample-5");
I've changed the cloudName
as per the cloudName
in my dashboard. I've then changed the public ID
to a sample image in my Media Library:
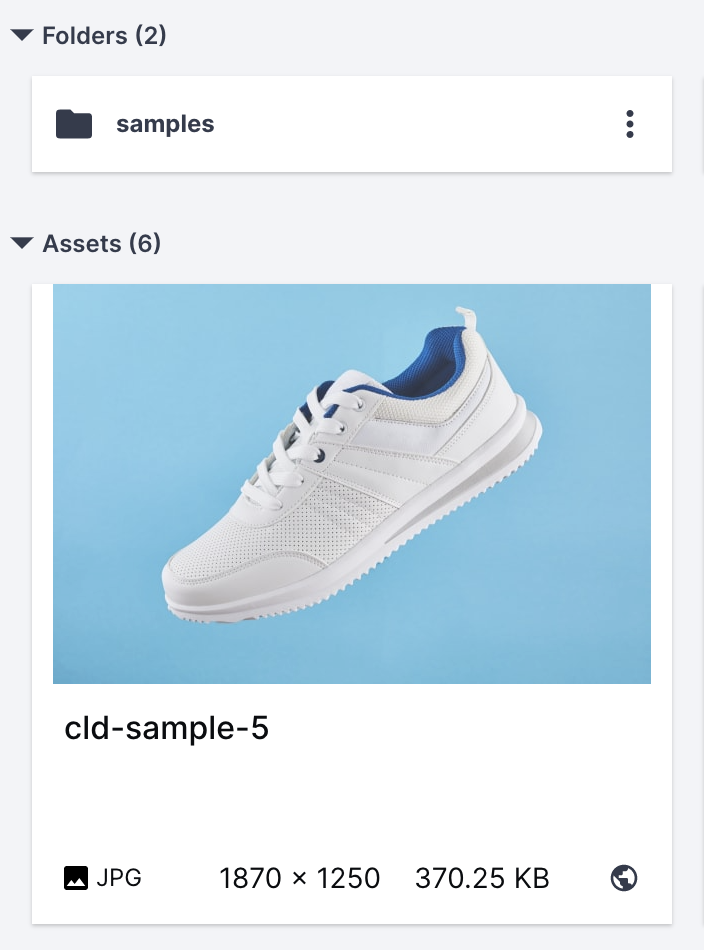
Unfortunately I keep getting a 404 error. What am I doing wrong?
CodePudding user response:
The Public ID on Cloudinary is the asset's identifier and it'll contain a filename (could be a randomly generated string of characters or the actual filename of the asset) any containing folder(s). In this case, and based on your screenshot, the cld-sample-5
asset is in the root folder but you're requesting it by passing the public_id set to samples/cld-sample-5
so unless you have an asset in the samples
folder called cld-sample-5
, the expected response is 404 as no asset exists for that public_id. If you want to target the cld-sample-5
asset then your public_id (that is passed to the image()
method) should just be cld-sample-5
.
In addition, in Cloudinary, an asset is unique/identified by not only the 'public_id' but only when that is in combination with the 'resource_type' ('image', 'video' or 'raw') and 'type' (e.g. 'upload', 'private', 'authenticated', 'fetch' etc). Therefore the below assets with the same public_id (sample) are actually different entities:
image/upload/cld-sample-5
image/private/cld-sample-5
video/upload/cld-sample-5
video/authenticated/cld-sample-5
The public_id is returned in the response for each successful upload and you can store the 'public_id', 'resource_type' and 'type' parameters in your database and then you can reference any asset on Cloudinary both for delivery and via the API.
Finally, API methods, such as upload()
and others, default the value of certain optional parameters if they are not provided. This includes the 'resource_type' (defaulted to "image") and also 'type' (defaulted to "upload"). This is why if you're uploading a "video" rather than an image, you will either need to pass resource_type: "video"
or resource_type: "auto"
to the upload() method - otherwise it'll default to "image" resource_type and you'll get an error back.