qr_code_scanner cannot scan after first time works correctly. It shows black screen as shown in screenshot. I used flutter version 3.3.0 and qr_code_scanner: ^1.0.1 . I test on android 13 and android 10. It does not work. After hot restart , it works first time. What should I do and what package do you recommend for QR scanner. I will show you code details.
when i try to scan second time, it shows as in : -
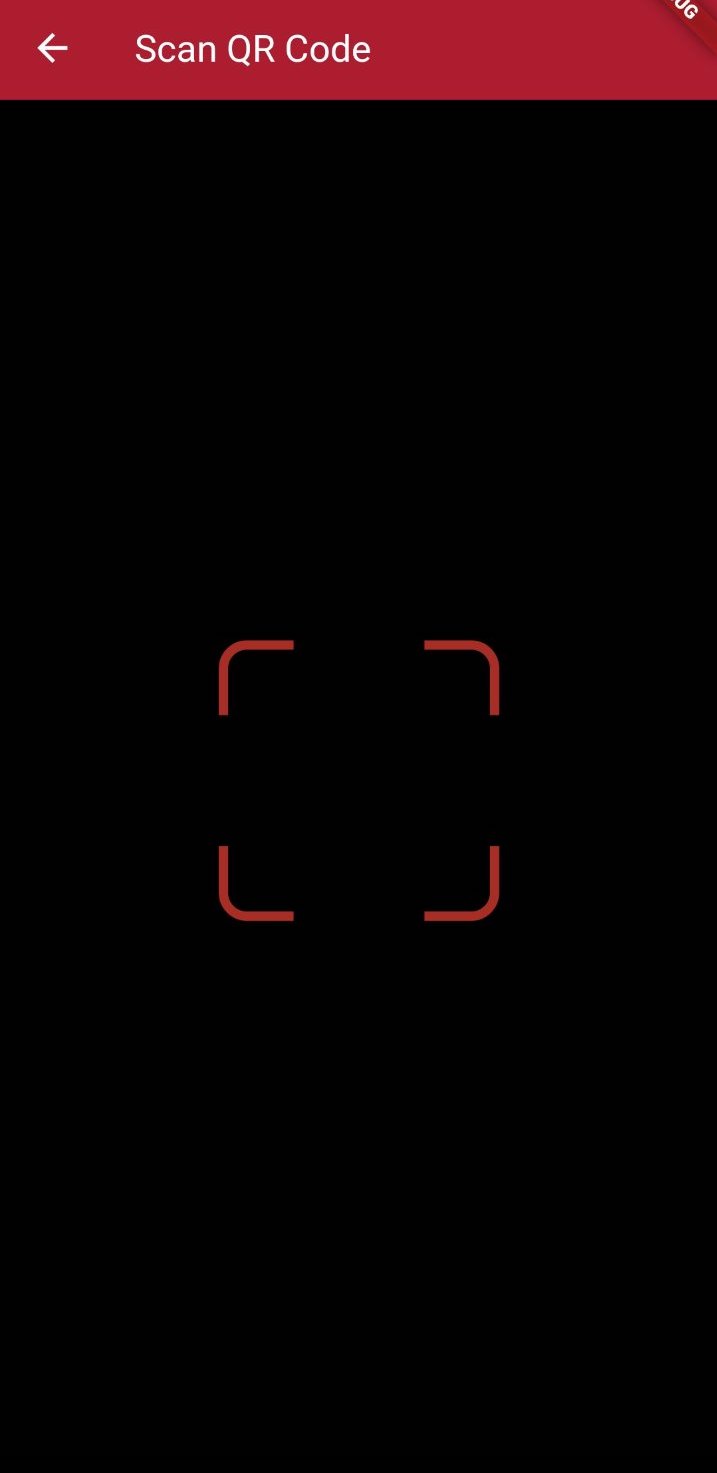
class QRScanScreen extends StatefulWidget {
const QRScanScreen({Key? key}) : super(key: key);
@override
State<QRScanScreen> createState() => _QRScanScreenState();
}
class _QRScanScreenState extends State<QRScanScreen> {
final GlobalKey qrKey = GlobalKey(debugLabel: 'QR');
Barcode? result;
QRViewController? controller;
@override
void initState() {
super.initState();
controller?.resumeCamera();
}
@override
void reassemble() {
super.reassemble();
if (Platform.isAndroid) {
controller!.pauseCamera();
} else if (Platform.isIOS) {
controller!.resumeCamera();
}
}
@override
void dispose() {
controller?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
controller?.resumeCamera();
final cashInBloc = CashInModuleProvider.of(context);
var scanArea = (MediaQuery.of(context).size.width < 400 ||
MediaQuery.of(context).size.height < 400)
? 150.0
: 300.0;
return Scaffold(
appBar: AppBar(
title: const Text(TITLE_SCAN_QR_CODE),
),
body: QRView(
key: qrKey,
onQRViewCreated: (controller) {
this.controller = controller;
controller.scannedDataStream.listen((scanData) {
setState((){
result = scanData.code;
});
Navigator.pop(context);
});
},
overlay: QrScannerOverlayShape(
borderColor: Colors.red,
borderRadius: 10,
borderLength: 30,
borderWidth: 10,
cutOutSize: scanArea,
),
onPermissionSet: (ctrl, p) => _onPermissionSet(context, ctrl, p),
),
);
}
void _onPermissionSet(BuildContext context, QRViewController ctrl, bool p) {
log('${DateTime.now().toIso8601String()}_onPermissionSet $p');
if (!p) {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('no Permission')),
);
}
}
}
CodePudding user response:
You did not implement it properly. You resumed camera onInit() method, before even controller was initialised. So, hot reloading was making it work. Next, why are you popping navigation everytime you scan an item? Is this your logic, to remove the current screen and go back to previous screen once the item has been successfully scanned? Anyway modify the below code to your logic. I have modified your code below and it works now:
class QRScanScreen extends StatefulWidget {
const QRScanScreen({Key? key}) : super(key: key);
@override
State<QRScanScreen> createState() => _QRScanScreenState();
}
class _QRScanScreenState extends State<QRScanScreen> {
final GlobalKey qrKey = GlobalKey(debugLabel: 'QR');
Barcode? result;
QRViewController? controller;
@override
void initState() {
super.initState();
// controller?.resumeCamera();
}
void resumeCamera() {
if (Platform.isAndroid) {
controller?.pauseCamera();
}
controller?.resumeCamera();
}
@override
void dispose() {
controller?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
var scanArea = (MediaQuery.of(context).size.width < 400 ||
MediaQuery.of(context).size.height < 400)
? 150.0
: 300.0;
return Scaffold(
appBar: AppBar(
),
body: QRView(
key: qrKey,
onQRViewCreated: (controller) {
setState(() {
this.controller = controller;
});
resumeCamera();
controller.scannedDataStream.listen((scanData) {
setState((){
result = scanData;
});
// Navigator.pop(context);
});
},
overlay: QrScannerOverlayShape(
borderColor: Colors.red,
borderRadius: 10,
borderLength: 30,
borderWidth: 10,
cutOutSize: scanArea,
),
onPermissionSet: (ctrl, p) => _onPermissionSet(context, ctrl, p),
),
);
}
void _onPermissionSet(BuildContext context, QRViewController ctrl, bool p) {
if (!p) {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('no Permission')),
);
}
}
}