When the app starts up, I want to display a 10x10 grid based on a ScrollView. When the app loads, I get this:
... when what I really want to see is
Seems like it should be easy but I can't seem to Google the correct solution. Any suggestions?
Edit: I am using SwiftUI. Here's what my code looks like ATM:
import SwiftUI
struct TableView: View {
var body: some View {
let width = 0...10
let height = 0...10
NavigationView {
ScrollView ([.horizontal, .vertical]) {
VStack (alignment: .center, spacing: 0) {
ForEach (height, id: \.self) { h in
HStack (alignment: .center, spacing: 0){
ForEach (width, id: \.self) { w in
if (h == 5 && w == 5) {
Image(uiImage: UIImage(named: "X.png")!)
.resizable()
.frame(width: 40, height: 40, alignment: .center)
} else {
Image(uiImage: UIImage(named: "Blank.png")!)
.resizable()
.frame(width: 40, height: 40, alignment: .center)
}
}
}
}
}
.frame(width: 1200, height: 1200, alignment: .center )
} // end ScrollView
}
}
}
struct TableView_Previews: PreviewProvider {
static var previews: some View {
TableView()
}
}
CodePudding user response:
ScrollView won't center itself by default — you'll need to add a ScrollViewReader
.
ScrollView ([.horizontal, .vertical]) {
ScrollViewReader { proxy in /// here!
VStack (alignment: .center, spacing: 0) {
ForEach (height, id: \.self) { h in
HStack (alignment: .center, spacing: 0){
ForEach (width, id: \.self) { w in
if (h == 5 && w == 5) {
Image(uiImage: UIImage(named: "X.png")!)
.resizable()
.frame(width: 40, height: 40, alignment: .center)
} else {
Image(uiImage: UIImage(named: "Blank.png")!)
.resizable()
.frame(width: 40, height: 40, alignment: .center)
}
}
}
}
}
.onAppear {
proxy.scrollTo(5) /// scroll to center
}
}
}
.frame(width: 1200, height: 1200, alignment: .center)
Result:
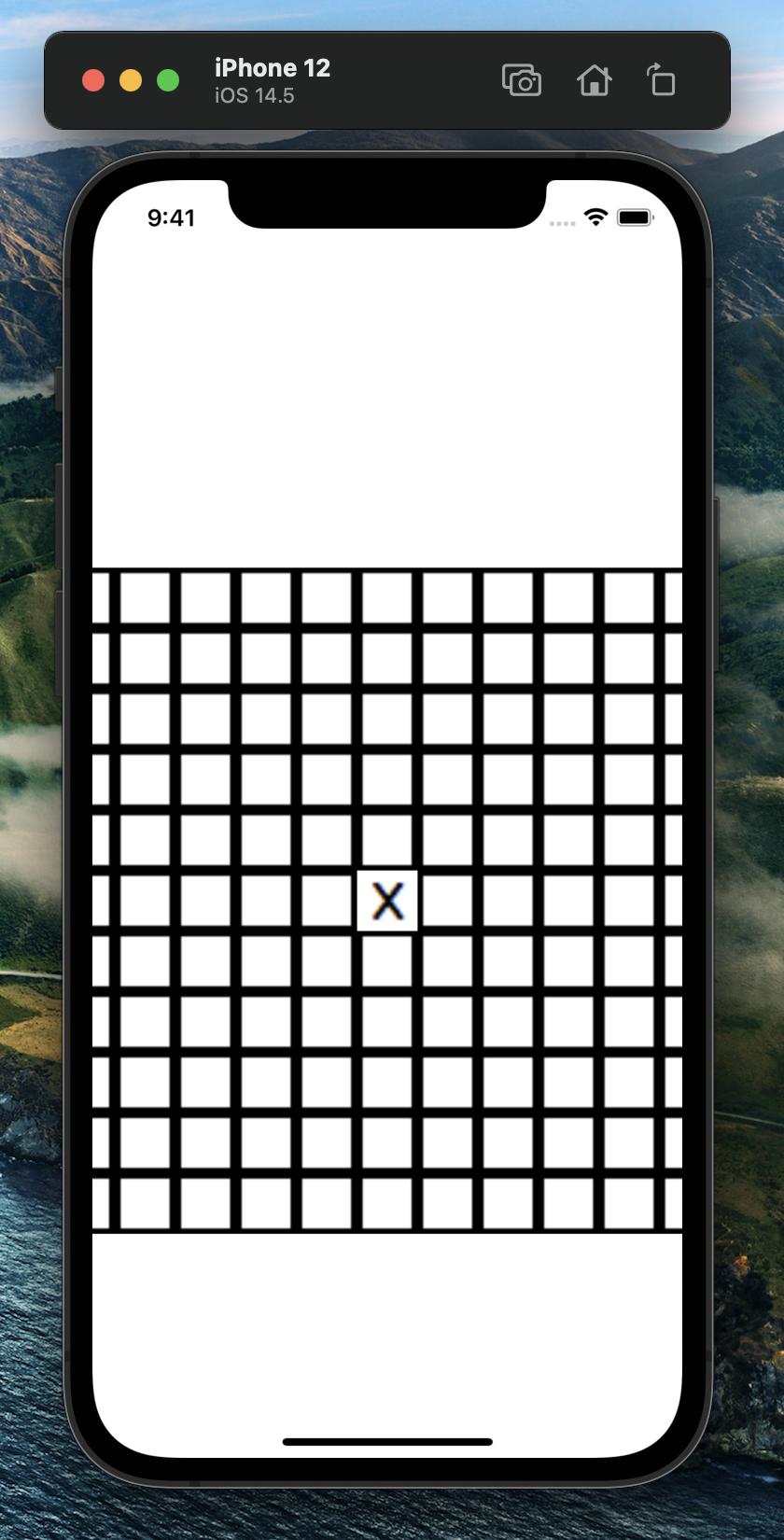