I'm trying to load an image tag into my HTML. The problem is that the generated tag appears as text and the image is not loaded. How can I solve this? In the end, it should look like the example.
This is the XML DATA info2.xml
<BILD ID="8">
<ID>8</ID>
<PIC><IMG SRC="https://d1pgrp37iul3tg.cloudfront.net/zimmer_pics/zim_238461_008.jpg" width="586" height="480" BORDER=0></PIC>
</BILD><BILD ID="6">
<ID>6</ID>
<PIC><IMG SRC="https://d1pgrp37iul3tg.cloudfront.net/zimmer_pics/zim_238461_006.jpg" width="640" height="480" BORDER=0></PIC>
</BILD><BILD ID="1">
<ID>1</ID>
<PIC><IMG SRC="https://d1pgrp37iul3tg.cloudfront.net/zimmer_pics/zim_238461_001.jpg" width="640" height="480" BORDER=0></PIC>
</BILD><BILD ID="4">
<ID>4</ID>
<PIC><IMG SRC="https://d1pgrp37iul3tg.cloudfront.net/zimmer_pics/zim_238461_004.jpg" width="638" height="480" BORDER=0></PIC>
</BILD>
<script>
let xmlContent = '';
let tableGallery = document.getElementById('image-gallery');
fetch('info2.xml').then((response)=> {
response.text().then((xml)=>{
xmlContent = xml;
let parser = new DOMParser();
let xmlDOM = parser.parseFromString(xmlContent, 'application/xml');
let gallery = xmlDOM.querySelectorAll('BILD');
gallery.forEach(galleryXmlNode => {
let row = document.createElement('li');
//Picture
let img = document.createElement('');
img.innerHTML = galleryXmlNode.children[1].innerHTML;
row.appendChild(img);
tableGallery.children[1].appendChild(row);
});
});
});
</script>
<!--gallery-->
<div class="clearfix" style="max-width:540px;">
<ul id="image-gallery" class="gallery list-unstyled cS-hidden">
<li data-thumb="images/knurrhahn/thumbs/zim_s_238842_007.jpg">
<img src="images/knurrhahn/site/obj_full_91963_003.jpg" />
</li>
<li data-thumb="images/knurrhahn/thumbs/zim_s_238842_015.jpg">
<img src="images/knurrhahn/site/zim_full_238842_015.jpg" />
</li>
</ul>
</div>
<!--gallery end-->
<iframe name="sif1" sandbox="allow-forms allow-modals allow-scripts" frameborder="0"></iframe>
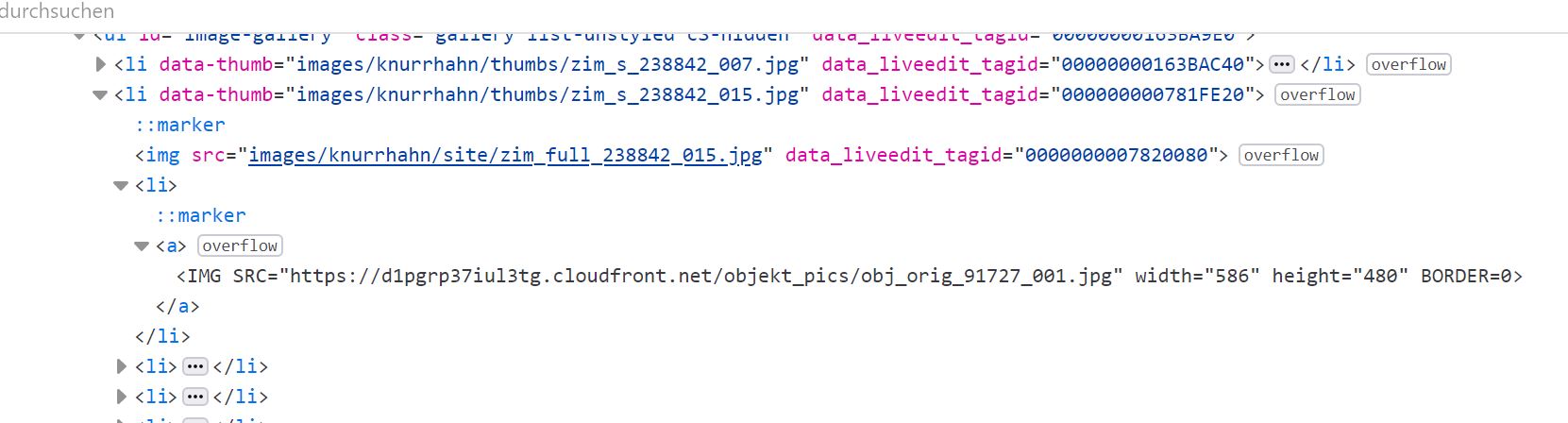
CodePudding user response:
As mentioned in the comments, your xml is indeed not well formed. If you really can't get the source to generate well formed xml, you can try to treat it as html and unescape the html entities. Long term, however, it's not the best way to proceed.
The following will work, at least in the case of your example xml:
let parser = new DOMParser();
let xmlDOM = parser.parseFromString(xmlContent, "text/html");
let gallery = xmlDOM.evaluate(
"//BILD//PIC",
xmlDOM,
null,
XPathResult.ORDERED_NODE_SNAPSHOT_TYPE,
null
);
for (let i = 0; i < gallery.snapshotLength; i ) {
let galleryXmlNode = gallery.snapshotItem(i);
row = document.createElement("li");
row.innerHTML = galleryXmlNode.textContent
tableGallery.appendChild(row);
}