I have created a GridLayout(3,2)
in Java and inserted 6 JLabel
components. They should be inserted in order of the code written, but they are arranged in descending order. Why?
import java.awt.GridLayout;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class Summary_Graph_JPanel1 extends JPanel {
public Summary_Graph_JPanel1() {
GridLayout g=new GridLayout(3,2);
setLayout(g);
add(new JLabel("1"),JLabel.CENTER);
add(new JLabel("2"),JLabel.CENTER);
add(new JLabel("3"),JLabel.CENTER);
add(new JLabel("4"),JLabel.CENTER);
add(new JLabel("5"),JLabel.CENTER);
add(new JLabel("6"),JLabel.CENTER);
}
}
This is how it looks:
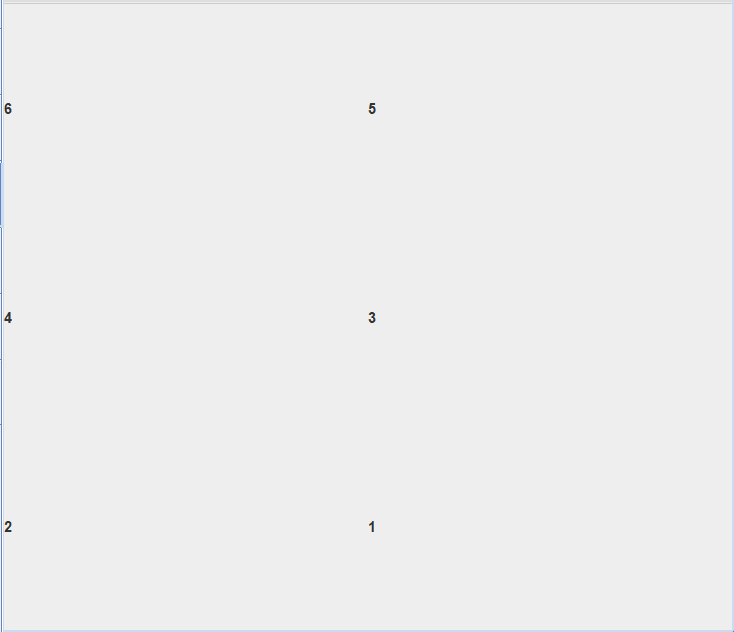
CodePudding user response:
The use of JLabel.CENTER
is in the wrong place. It should be within the JLabel
constructor rather than handed to the add(JLabel, constraint)
method. It is not an appropriate constraint of the layout, but of the label.
import java.awt.GridLayout;
import javax.swing.*;
public class Summary_Graph_JPanel1 extends JPanel {
public Summary_Graph_JPanel1() {
GridLayout g=new GridLayout(3,2);
setLayout(g);
add(new JLabel("1",JLabel.CENTER));
add(new JLabel("2",JLabel.CENTER));
add(new JLabel("3",JLabel.CENTER));
add(new JLabel("4",JLabel.CENTER));
add(new JLabel("5",JLabel.CENTER));
add(new JLabel("6",JLabel.CENTER));
}
public static void main(String[] args) {
JOptionPane.showMessageDialog(null, new Summary_Graph_JPanel1());
}
}
CodePudding user response:
The use of JLabel.CENTER
is in the wrong place. It should be within the JLabel
constructor rather than handed to the add(Component comp, int index)
method. JLabel.CENTER
is being used as an index since it is int
. The labels are therefore being inserted at the first position (index 0
), instead of being appended at the end, so they end up in the reversed order.
import java.awt.GridLayout;
import javax.swing.*;
public class Summary_Graph_JPanel1 extends JPanel {
public Summary_Graph_JPanel1() {
GridLayout g=new GridLayout(3,2);
setLayout(g);
add(new JLabel("1",JLabel.CENTER));
add(new JLabel("2",JLabel.CENTER));
add(new JLabel("3",JLabel.CENTER));
add(new JLabel("4",JLabel.CENTER));
add(new JLabel("5",JLabel.CENTER));
add(new JLabel("6",JLabel.CENTER));
}
public static void main(String[] args) {
JOptionPane.showMessageDialog(null, new Summary_Graph_JPanel1());
}
}
based on (copy) this answer as requested by author in this comment :-/