I have angular material date picker control in my web app. By default it shows that the date field is mandatory by putting the 'asterisk' following the mat-label. The below code ilustrates that case:
<div >
<div >
<mat-form-field appearance="outline" enabled>
<mat-label enabled>DOB</mat-label>
<input formControlName="dob" #ref matInput [ngxMatDatetimePicker]="picker"
placeholder="Choose a date" required />
<mat-datepicker-toggle matSuffix [for]="$any(picker)"></mat-datepicker-toggle>
<mat-hint>YYYY-MM-DD</mat-hint>
<ngx-mat-datetime-picker #picker [showSpinners]="true" [showSeconds]="false" [stepHour]="1"
[stepMinute]="1" [stepSecond]="1" [touchUi]="false" [color]="undefined" [enableMeridian]="false"
[disableMinute]="false" [hideTime]="true">
</ngx-mat-datetime-picker>
<mat-error *ngIf="f['dob'].errors">Invalid DOB</mat-error>
</mat-form-field>
</div>
</div>
... so it looks on the screen like this:
I would like the star (asterisk) to be displayed in red
colour.
I have tried this css:
span.mdc-text-field:not(.mdc-text-field--disabled) .mdc-floating-label {
color: red;
font-size: 18px;
}
... but it doesn't work.
Is there any way to force it to be red
.
CodePudding user response:
I figured out you have to use :host ::ng-deep
in the local stylesheet of your component, otherwise your css-selector won't be applied. Additionally, to specifically target the asterisk rather than the entire label text, you have to include .mat-mdc-form-field-required-marker
in your selector.
In case you use CSS
, add the following to you local component's stylesheet:
:host ::ng-deep .mdc-text-field:not(.mdc-text-field--disabled) .mdc-floating-label .mat-mdc-form-field-required-marker {
color: red;
}
In case you use SCSS
, add the following selector instead:
:host ::ng-deep .mdc-text-field:not(.mdc-text-field--disabled) {
.mdc-floating-label {
.mat-mdc-form-field-required-marker {
color: red;
}
}
}
CodePudding user response:
You can use .mat-mdc-form-field-required-marker
to style the asterisk and change color
to what you want. Here's an example:
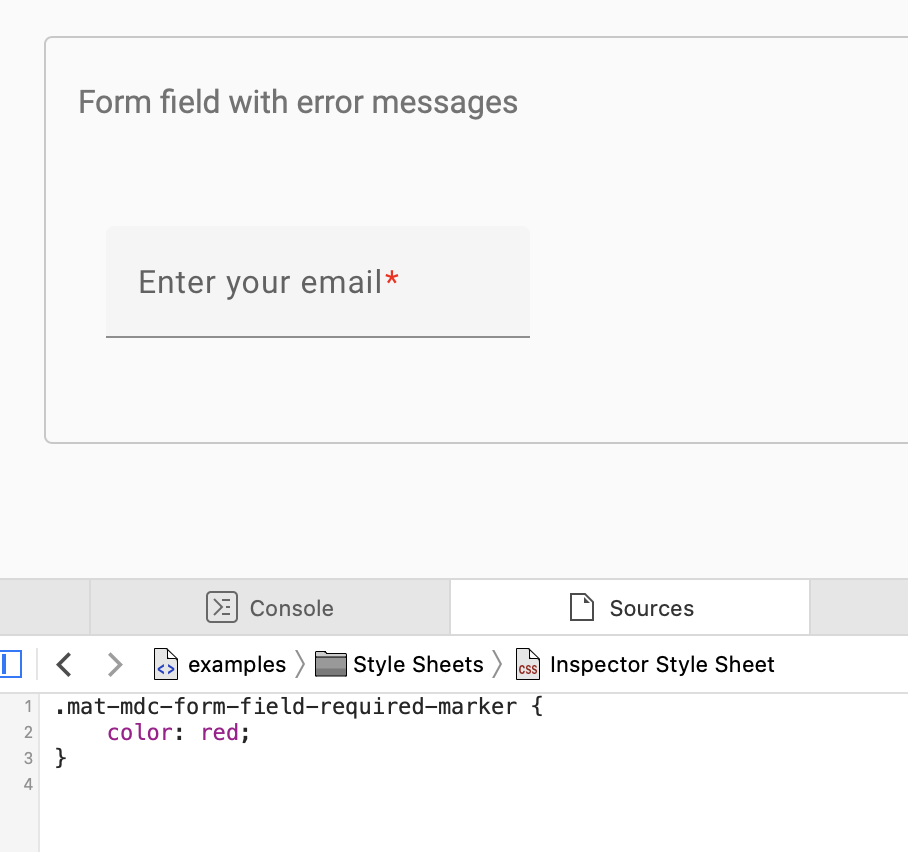
Encapsulation
But if you're NOT writing this style in a global stylesheet, it won't work. This is due to the way that Angular components encapsulates every style rule in your component-level CSS and so that your styles don't go out of their scope, i.e. Material Design components.
But here you actually want to work around this encapsulation and one way is to set ViewEncapsulation.None
in your @Component
's encapsulation
metadata:
@Component({
...
encapsulation: ViewEncapsulation.None
})
or to use ::ng-deep
before your CSS selector. ::ng-deep
simply disables view-encapsulation for that particular CSS rule, meaning you can access deeper levels of your components, i.e:
::ng-deep .mat-mdc-form-field-required-marker { /* your code here */ }