developers; I have three arrays
: Days (columns), Subject (rows), Data(cells)
let Days = ["Monday", "Tuesday", "Wednesday"];
let Subject = [
"Economics",
"geography",
"theatre",
"music",
"mathematics",
"psychology",
"marketing",
"business",
"journalism",
"languages",
];
const Data = [
["10:00", "12:10", "13:30"],
["08:30", "12:10", "14:30"],
["14:10", "15:15", "19:10"],
["20:20", "20:50", "23:00"],
["09:10", "10:00", "19:00"],
["10:12", "16:40", "18:10"],
["08:30", "12:10", "14:30"],
["14:10", "15:15", "19:10"],
["20:20", "20:50", "23:00"],
["08:10", "14:20", "20:20"],
];
I wanna make a relation between those arrays:
I've tried something like playing with modulo and looping over product of rows and cells:
const cols = Days.length;
const rows = Subject.length;
const len = Subject.length * Days.length;
const table = [];
for (let i = 0, j = 0; i < len; i ) {
table.push([
Subject[i % rows],
Days[j % cols],
Data[i % rows][j % cols],
]);
j ;
}
console.table(table);
But it gives results not expected in order:
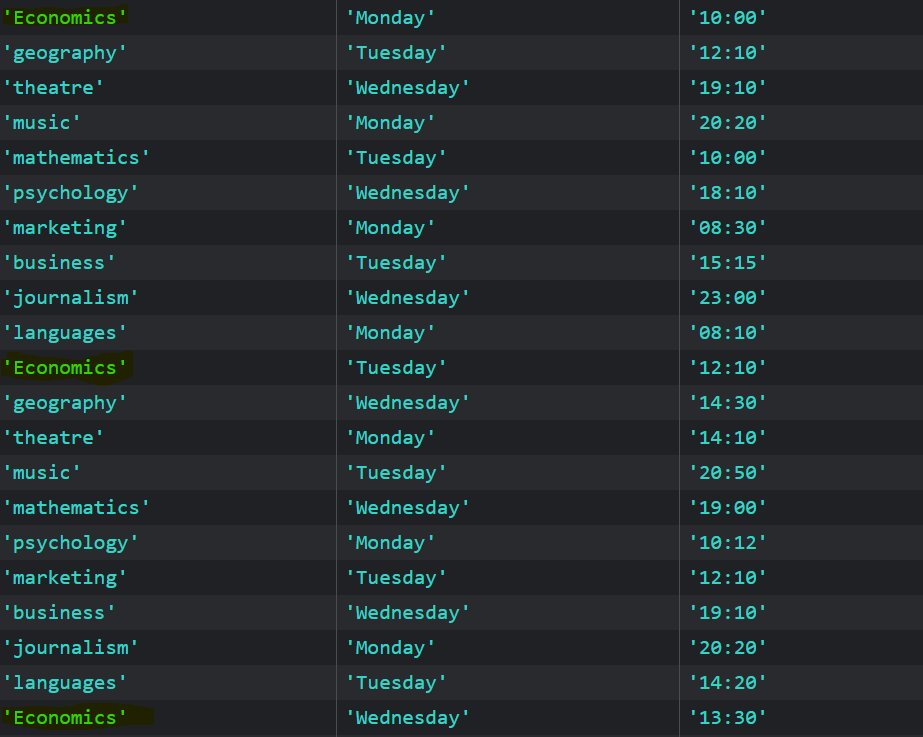
I still trying to sorting it but does not work since sorting would sort in alphabet order which doesn't keep the order?
console.table(table.sort((a, b) => a[0].localeCompare(b[0])));
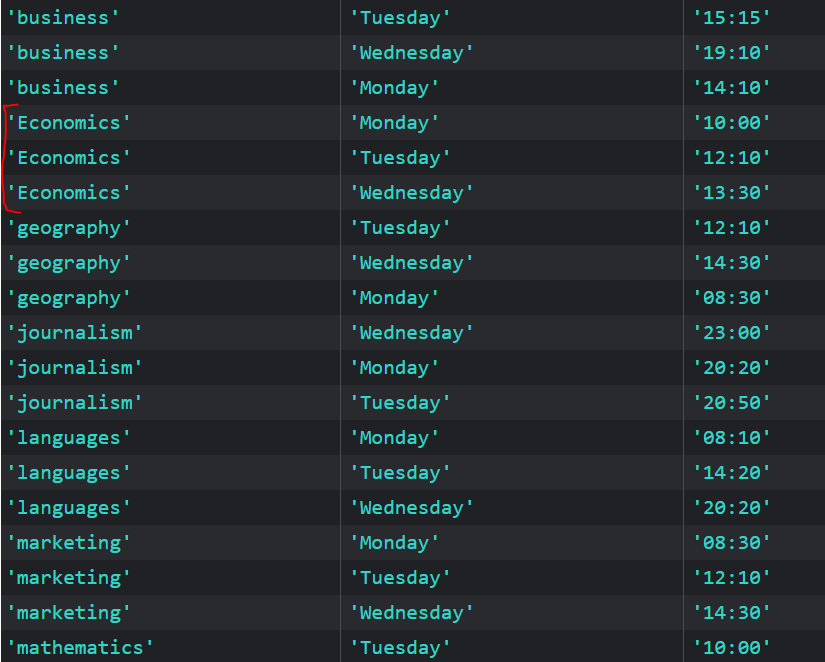
Thanks in advance, any suggestions are welcome and appreciated
CodePudding user response:
Simply by doing the sort while doing the product process, as follows:
for (let i = 0; i < rows; i ) {
for (let j = 0; j < cols; j ) {
table.push([
Subject[I],
Days[j],
Data[i][j],
]);
}
}
In this case, you will be iterating each row from Subject with all the corresponding values from Days, before moving to the next row of Subject, ending with the desired order of elements.
For example:
the first iteration will be as follows (representing the first three row of the resulting array)
i = 0 (Subject[i] = "Economics")
j = 0 (Days[j] = "Monday") ---> Data[i][j] = "10:00"
j = 1 (Days[j] = "Tuesday") ---> Data[i][j] = "12:10"
j = 2 (Days[j] = "Wednesday") ---> Data[i][j] = "13:30"
CodePudding user response:
you can do it easily by just lop twice, rows and cols.
let Days = ["Monday", "Tuesday", "Wednesday"];
let Subject = [
"Economics",
"geography",
"theatre",
"music",
"mathematics",
"psychology",
"marketing",
"business",
"journalism",
"languages",
];
const Data = [
["10:00", "12:10", "13:30"],
["08:30", "12:10", "14:30"],
["14:10", "15:15", "19:10"],
["20:20", "20:50", "23:00"],
["09:10", "10:00", "19:00"],
["10:12", "16:40", "18:10"],
["08:30", "12:10", "14:30"],
["14:10", "15:15", "19:10"],
["20:20", "20:50", "23:00"],
["08:10", "14:20", "20:20"],
];
const cols = Days.length;
const rows = Subject.length;
const len = Subject.length * Days.length;
const table = [];
for (let i = 0; i < rows; i ) {
for (let j = 0; j < cols; j ) {
table.push([
Subject[i],
Days[j],
Data[i][j],
]);
}
}
console.table(table);