What I am trying to achieve is like how we see text messages like the image below. But when I put a long text I am getting the screen overflow error. I tried using softwrap: true
with all the overflow: TextOverflow.xxxx
options, but doesn't seem to do what I am looking for. For reference I put my code here.
Container(
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(10)),
child: Container(
padding:
EdgeInsets.all(5),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.end,
children: [
Text(
'Add a very long text the goes a few lines',
style: TextStyle(fontSize: 16, color: Colors.black),
softWrap: true,
overflow: TextOverflow.visible,
),
Text(
'3:08',
style:
TextStyle(fontSize: 12, color: Colors.black38),
),
],
),
),
),
CodePudding user response:
You can wrap Text
with Flexible
widget to fix the overflow.
Container(
decoration: BoxDecoration(
color: Colors.white, borderRadius: BorderRadius.circular(10)),
child: Container(
padding: EdgeInsets.all(5),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.end,
children: [
Flexible(
child: Text(
'Add a very long text the goes a few lines',
style: TextStyle(fontSize: 16, color: Colors.black),
softWrap: true,
overflow: TextOverflow.visible,
),
),
Text(
'3:08',
style: TextStyle(fontSize: 12, color: Colors.black38),
),
],
),
),
),
CodePudding user response:
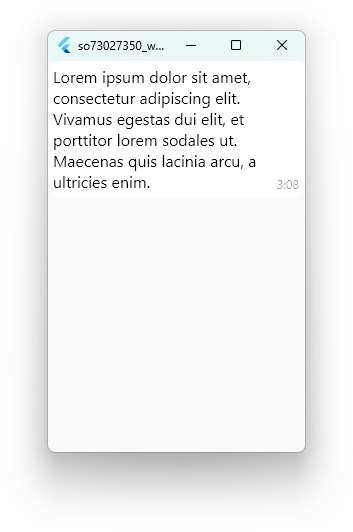
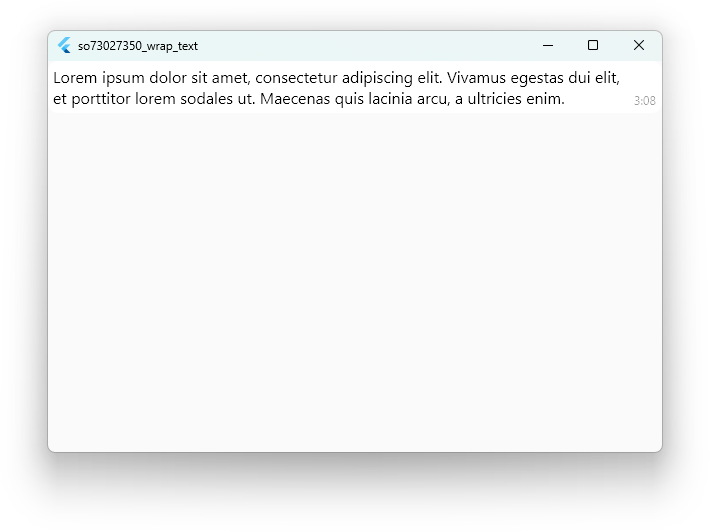
Here's the code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
debugShowCheckedModeBanner: false,
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
decoration: BoxDecoration(
color: Colors.white, borderRadius: BorderRadius.circular(10)),
child: Container(
padding: const EdgeInsets.all(5),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.end,
children: const [
Expanded(
child: Text(
'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vivamus egestas dui elit, et porttitor lorem sodales ut. Maecenas quis lacinia arcu, a ultricies enim.',
style: TextStyle(fontSize: 16, color: Colors.black),
softWrap: true,
overflow: TextOverflow.visible,
),
),
Text(
'3:08',
style: TextStyle(fontSize: 12, color: Colors.black38),
),
],
),
),
),
);
}
}