I want to find the pink wood in the picture.
The code does this correctly, but in some images, such as the image below, it finds the pink stick correctly but finds several other boxes incorrectly.
In fact, instead of finding one coordinate (x,y,w,h)
, it misdiagnoses several coordinates.
The image below is quite clear
import numpy as np
imagePath = "core4.jpg"
import cv2
from cv2 import *
im = cv2.imread(imagePath)
im = cv2.bilateralFilter(im,9,75,75)
im = cv2.fastNlMeansDenoisingColored(im,None,10,10,7,21)
hsv_img = cv2.cvtColor(im, cv2.COLOR_BGR2HSV) # HSV image
COLOR_MIN = np.array([130,0,220],np.uint8)
COLOR_MAX = np.array([170,255,255],np.uint8)
frame_threshed = cv2.inRange(hsv_img, COLOR_MIN, COLOR_MAX) # Thresholding image
imgray = frame_threshed
ret,thresh = cv2.threshold(frame_threshed,127,255,0)
contours, hierarchy = cv2.findContours(thresh,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
print(x,y,w,h)
cv2.rectangle(im,(x,y),(x w,y h),(0,255,0),2)
cv2.imwrite("core4_cropped.jpg", im)
input

output (incorrect several boxes detected)
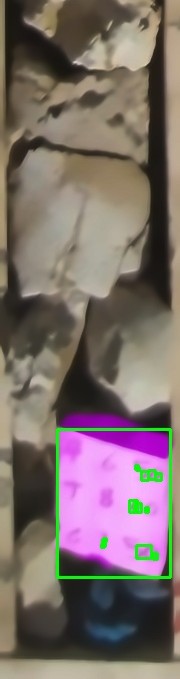
instead of finding one coordinate (x,y,w,h), it misdiagnoses several coordinates bounding boxes.
57 2410 113 148
153 2534 4 6
136 2526 15 13
101 2525 3 3
102 2520 3 7
103 2519 3 3
145 2488 3 5
134 2484 7 9
129 2481 8 12
155 2454 6 7
141 2452 7 9
148 2451 7 9
136 2448 3 3
135 2446 3 4
109 1416 4 3
106 1414 1 1
What could be the reason for this error?
notice: Numbers are written on pink wood.
CodePudding user response:
I would lower the threshold of the pink color or apply a blur to the img to destroy all small details like in this example the numbers. Also I would recommend for something like this to use "Rotated Rectangle". It will display the exact rectangle and rotate it. In your case you are using "Straight Bounding Rectangle". You can find out more about it here. Scroll to 7.b.