I'm using a nested MaterialApp such that, FirstMaterialApp
has SecondMaterialApp
as its child. I'm facing an issue when calling showDialog
from SecondMaterialApp
, that is it appears on the entire screen as if it is opened from the FirstMaterialApp
.
I want that the dialog remains confined to the boundaries of the SecondMaterialApp
.
In the image, I have intentionally stretched the Dialog across the width so that it is apparent that it covers the FirstMaterialApp
.
First MaterialApp
class FirstMaterialApp extends StatelessWidget {
const FirstMaterialApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'First Material App',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('First App Scaffold'),
),
body: Center(
child: DeviceFrame(
device: Devices.ios.iPhone12, screen: const SecondMaterialApp()),
));
}
}
Second MateriaApp
class SecondMaterialApp extends StatelessWidget {
const SecondMaterialApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Second Materia App', home: SecondScaffold());
}
}
class SecondScaffold extends StatelessWidget {
const SecondScaffold({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
margin: const EdgeInsets.only(top: 40.0),
child: Scaffold(
appBar: AppBar(
title: const Text('Second App Home'),
),
body: Center(
child: TextButton(
child: const Text('Open Dialog'),
onPressed: () async {
await showDialog(
context: context,
builder: (buildContext) => CustomDialog());
},
),
),
),
);
}
}
class CustomDialog extends StatelessWidget {
const CustomDialog({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Dialog(
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: const [
Text(
'Dialog',
style: TextStyle(fontSize: 20.0),
),
Text(
'Message Text',
),
],
),
);
}
}
CodePudding user response:
Found the solution by using a showDialog
parameter named useRootNavigator
. Setting it to false
provided the required results.
Now the dialog is confined to the boundaries of child MaterialApp and the backgroundOverly
from showDialog
covers only the second material app.
CodePudding user response:
-> use Your Second File code
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class SecondMaterialApp extends StatelessWidget {
const SecondMaterialApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Second Materia App', home: SecondScaffold());
}
}
class SecondScaffold extends StatelessWidget {
const SecondScaffold({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
margin: const EdgeInsets.only(top: 40.0),
child: Scaffold(
appBar: AppBar(
title: const Text('Second App Home'),
),
body: Center(
child: TextButton(
child: const Text('Open Dialog'),
onPressed: () async {
await showDialog(
context: context,
builder: (buildContext) => const CustomDialog());
},
),
),
),
);
}
}
class CustomDialog extends StatelessWidget {
const CustomDialog({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return CupertinoAlertDialog(
content: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: const \[
Text(
'Dialog',
style: TextStyle(fontSize: 20.0),
),
Text(
'Message Text',
),
\],
),
);
}
}
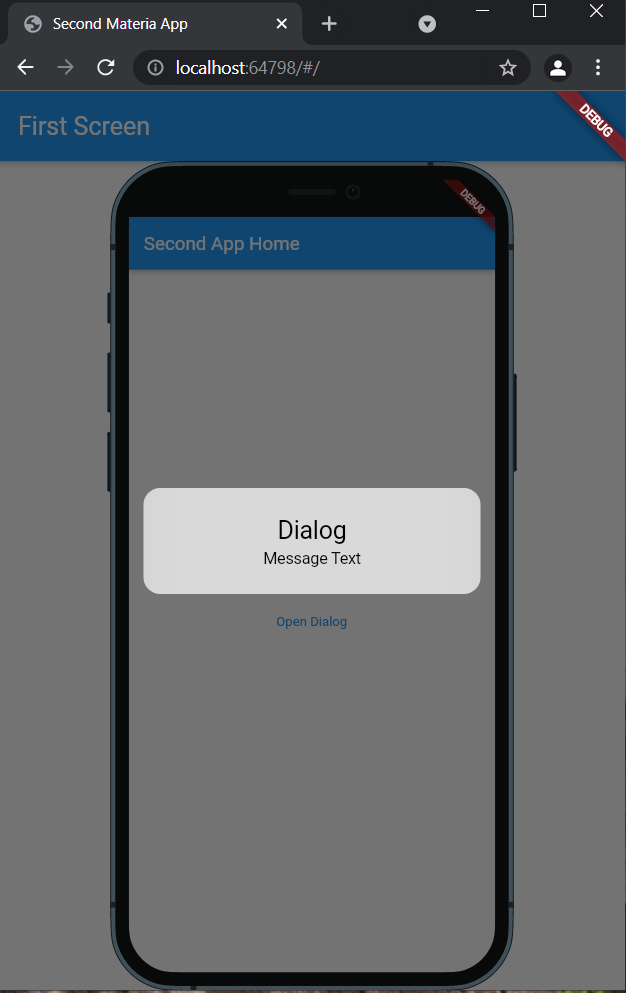
CodePudding user response:
create material before dialog
return Material(child: Dialogue();