Trying to create cursor for counting the print all the country and got these errors
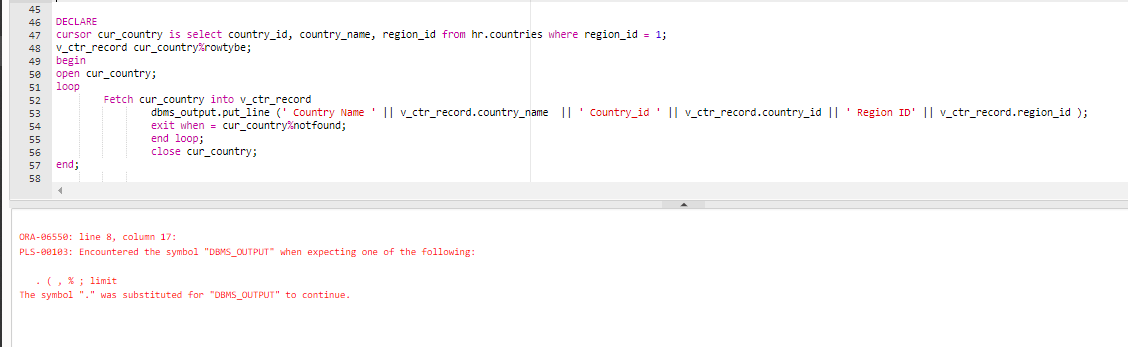
CodePudding user response:
- %rowtyBe?
fetch
should be terminated by a semi-colonwhen
clause doesn't have=
exit
clause should immediately followfetch
So, for sample data
SQL> SELECT * FROM countries;
COUNTRY_ID COUNTRY REGION_ID
---------- ------- ----------
1 Croatia 1
2 Austria 1
3 Germany 2
SQL> SET SERVEROUTPUT ON
that PL/SQL block might look like this:
SQL> DECLARE
2 CURSOR cur_country IS
3 SELECT country_id, country_name, region_id
4 FROM countries
5 WHERE region_id = 1;
6
7 v_ctr_record cur_country%ROWTYPE;
8 BEGIN
9 OPEN cur_country;
10
11 LOOP
12 FETCH cur_country INTO v_ctr_record;
13
14 EXIT WHEN cur_country%NOTFOUND;
15
16 DBMS_OUTPUT.put_line (
17 'Country name '
18 || v_ctr_record.country_name
19 || ' country_id '
20 || v_ctr_record.country_id
21 || ' Region id '
22 || v_ctr_record.region_id);
23 END LOOP;
24
25 CLOSE cur_country;
26 END;
27 /
Country name Croatia country_id 1 Region id 1
Country name Austria country_id 2 Region id 1
PL/SQL procedure successfully completed.
SQL>
Alternatively, switch to a cursor FOR
loop; it is simpler to use:
SQL> BEGIN
2 FOR v_ctr_record IN (SELECT country_id, country_name, region_id
3 FROM countries
4 WHERE region_id = 1)
5 LOOP
6 DBMS_OUTPUT.put_line (
7 'Country name '
8 || v_ctr_record.country_name
9 || ' country_id '
10 || v_ctr_record.country_id
11 || ' Region id '
12 || v_ctr_record.region_id);
13 END LOOP;
14 END;
15 /
Country name Croatia country_id 1 Region id 1
Country name Austria country_id 2 Region id 1
PL/SQL procedure successfully completed.
SQL>