I am working in textfield in jetpack compose. I want to build something like this
TextField(
value = value,
onValueChange = {
value = it
},
modifier = Modifier
.requiredWidth(56.dp)
.padding(10.dp),
colors = TextFieldDefaults.textFieldColors(
backgroundColor = OffWhite,
focusedIndicatorColor = TealLight,
cursorColor = TealLight
)
)
Scenario 1
When I am trying this
.requiredWidth(56.dp)
or
.width(56.dp)
It looks like this in the design
Scenario 2
When I try this
.widthIn(min = 56.dp)
It look like this
Scenario 3
When I try this
.fillMaxWidth()
It takes the whole screen width.
So my question is which one is used for which case? and what would be best attribute in my case?
CodePudding user response:
For your use-case requiredWidth()
is to be used.
1. fillMaxWidth
Use to fill the available width.
It takes a fraction as parameter, and defaults to 1.0F
if not provided.
2. widthIn
Use widthIn
to provide a size range.
It takes min width and max width as parameters
3. requiredWidth
Use requiredWidth
to force to a specific width.
(This is for your use-case)
4. width
Use width
to specify a preferred width. The given preferred width is used if there are no constraints overriding the given preference.
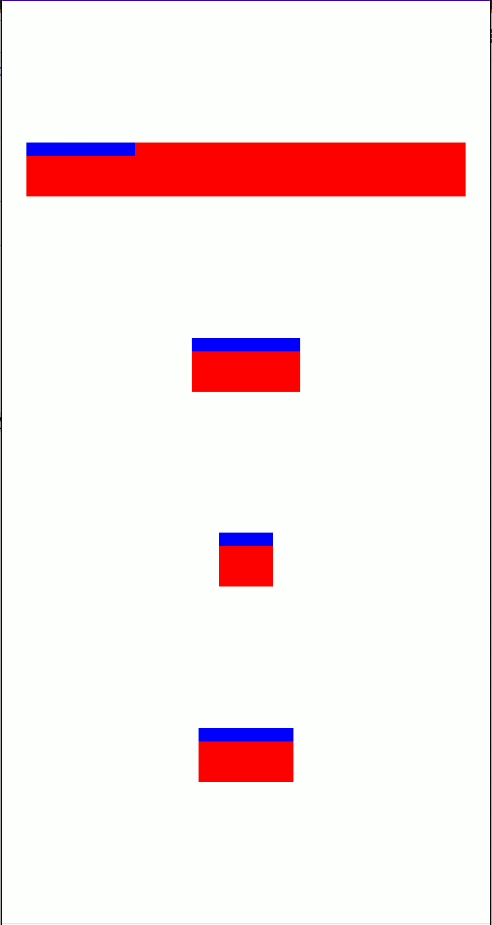
Sample code
@Composable
fun WidthTypes() {
Column(
modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.SpaceEvenly,
) {
Box(
modifier = Modifier
.background(Red)
.height(40.dp)
.fillMaxWidth(0.9F),
) {
Box(
modifier = Modifier
.background(Blue)
.height(10.dp)
.width(80.dp),
)
}
Box(
modifier = Modifier
.background(Red)
.height(40.dp)
.widthIn(40.dp, 100.dp),
) {
Box(
modifier = Modifier
.background(Blue)
.height(10.dp)
.width(80.dp),
)
}
Box(
modifier = Modifier
.background(Red)
.height(40.dp)
.requiredWidth(40.dp),
) {
Box(
modifier = Modifier
.background(Blue)
.height(10.dp)
.width(80.dp),
)
}
Box(
modifier = Modifier
.background(Red)
.height(40.dp)
.widthIn(70.dp, 100.dp)
.width(40.dp),
) {
Box(
modifier = Modifier
.background(Blue)
.height(10.dp)
.width(80.dp),
)
}
}
}
Note, how the 4th red box is 70.dp
even though width
is specified as 40.dp
. This is because the widthIn
is used which overrides the width value.
This does not happen when we use requiredWidth
.
CodePudding user response:
I don't know if i got your question right
If you were asking help to make textfield just like the reference image: the first scenario fixed the width and you want to add height adjustment too using .height
In 2nd Scenario: when you give a min value it is allowed to make the size anything more than 56.dp. it not a option for your case since you want a fixed size