I just start learning React Native, and I added my bottom nav using Material Bottom Tabs.
My only issue is this purple circle around my icon when selected. I checked the docs looking for this default setting but couldn't find it.
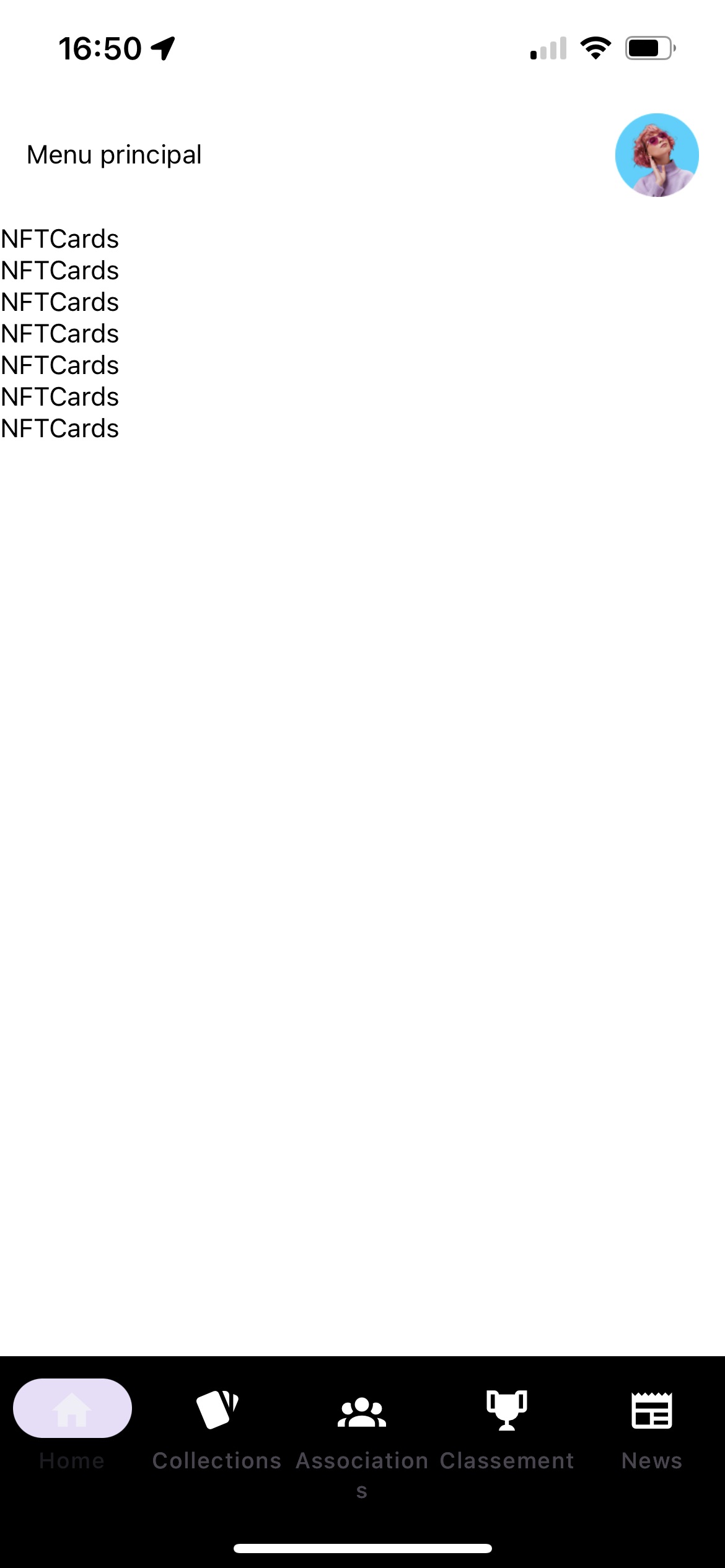
Here is my code:
const Tab = createMaterialBottomTabNavigator();
const App = () => {
const [loaded] = useFonts({
InterBold: require("./assets/fonts/Inter-Bold.ttf"),
InterSemiBold: require("./assets/fonts/Inter-SemiBold.ttf"),
InterMedium: require("./assets/fonts/Inter-Medium.ttf"),
InterRegular: require("./assets/fonts/Inter-Regular.ttf"),
InterLight: require("./assets/fonts/Inter-Light.ttf"),
});
if (!loaded) return null;
return (
<NavigationContainer theme={theme}>
<Tab.Navigator initialRouteName="Menu principal"
activeColor="#f0edf6"
inactiveColor="white"
barStyle={{ backgroundColor: 'black' }}
>
<Tab.Screen name="Menu principal" component={Home}
options={{
tabBarLabel: 'Home',
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="home" color={color} size={26} />
),
}}
/>
<Tab.Screen name="Collections" component={Collections}
options={{
tabBarLabel: 'Collections',
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="cards" color={color} size={26} />
),
}}
/>
<Tab.Screen name="Associations" component={Associations}
options={{
tabBarLabel: 'Associations',
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="account-group" color={color} size={26} />
),
}}
/>
<Tab.Screen name="Classement" component={Classement}
options={{
tabBarLabel: 'Classement',
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="trophy" color={color} size={26} />
),
}}
/>
<Tab.Screen name="News" component={News}
options={{
tabBarLabel: 'News',
tabBarIcon: ({ color }) => (
<MaterialCommunityIcons name="newspaper" color={color} size={26} />
),
}}
/>
</Tab.Navigator>
CodePudding user response:
Here is the solution I found. You have to npm install react-native-paper
, import, and use it.
To use react-native-paper to change the little circle tab buttons, wrap <Tab.Navigator>...</Tab.Navigator>
with <PaperProvider theme={theme}>
as shown below.
Finally, create a const theme
like I did, and change the secondaryContainer to whatever color you want.
P.S. make sure to change your import names as shown to not conflict with the Provider for redux.
import {
MD3LightTheme as DefaultTheme,
Provider as PaperProvider,
} from "react-native-paper";
const Tab = createMaterialBottomTabNavigator();
const theme = {
...DefaultTheme,
colors: {
...DefaultTheme.colors,
secondaryContainer: "red",
},
};
const App = () => {
return (
<NavigationContainer>
<PaperProvider theme={theme}>
<Tab.Navigator initialRouteName="Menu principal"
activeColor="#f0edf6"
inactiveColor="white"
barStyle={{ backgroundColor: 'black' }}
>
// rest of your code
</Tab.Navigator>
</PaperProvider>
</NavigationContainer>
)
}