I'm making a little ESP8266 project for myself, in which I want to display the Temperature and Humidity of an DHT11 sensor on a website. The code I have now works perfectly, but the timestamps at the charts are kinda weird. I want these timestamps to be the real time of Amsterdam when the data is uploaded to the charts. Is there anyone with any experience to do this? Let me know! Thanks in advance!
#include <DHT.h>
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#define DHTTYPE DHT11
#define DHTPIN 2
DHT dht(DHTPIN, DHTTYPE);
ESP8266WebServer server(80);
void setup() {
Serial.begin(115200);
WiFi.begin("SSID", "Password");
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
dht.begin();
server.on("/", []() {
String html = "<html><body>";
html = "<style> body{text-align:center; font-family:sans-serif;}#temp,#hum{color:red;}h1{font-size:75px;}.top{margin-top:50px;}</style>";
html = "<h1 class='top'>Temperatuur: <span id='temp'>Loading...</span> *C</h1>";
html = "<h1>Luchtvochtigheid <span id='hum'>Loading...</span> %</h1>";
html = "<script src='https://cdn.jsdelivr.net/npm/[email protected]/dist/Chart.min.js'></script>";
html = "<canvas id='tempChart'></canvas>";
html = "<canvas id='humChart' style='margin-top:50px;'></canvas>";
html = "<script>setInterval(function(){fetch('/temp-hum').then(res=>res.text()).then(tempHum=>{let [temp, hum] = tempHum.split(','); document.getElementById('temp').innerHTML=temp; document.getElementById('hum').innerHTML=hum});fetch('/getTempData').then(res => res.json()).then(data => {tempChart.data.labels.push(data.time); tempChart.data.datasets[0].data.push(data.temp); tempChart.update()});fetch('/getHumData').then(res => res.json()).then(data => {humChart.data.labels.push(data.time); humChart.data.datasets[0].data.push(data.hum); humChart.update()})}, 10000);</script>";
html = "<script>var tempCtx = document.getElementById('tempChart').getContext('2d');var tempChart = new Chart(tempCtx, {type: 'line',data: { labels: [],datasets: [{label: 'Temperature',data: [],borderColor: 'red',fill: false}]},options: {scales: {yAxes: [{scaleLabel: {display: true,labelString: 'Temperature (°C)'}}],xAxes: [{scaleLabel: {display: true,labelString: 'Time'}}]},title: {display: true,text: 'Temperature over time'},legend: {display: true }}});var humCtx = document.getElementById('humChart').getContext('2d');var humChart = new Chart(humCtx, {type: 'line',data:{ labels: [],datasets: [{label: 'Humidity',data: [],borderColor: 'blue',fill: false}]},options: {scales: {yAxes: [{scaleLabel: {display: true,labelString: 'Humidity (%)'}}],xAxes: [{scaleLabel: {display: true,labelString: 'Time'}}]},title: {display: true,text: 'Humidity over time'},legend: {display: true }}})</script>";
html = "</body></html>";
server.send(200, "text/html", html);
});
server.on("/temp-hum", []() {
String temp = String(dht.readTemperature());
String hum = String(dht.readHumidity());
String tempHum = temp "," hum;
server.send(200, "text/plain", tempHum);
});
server.on("/getTempData", []() {
String time = String(millis());
String temp = String(dht.readTemperature());
String data = "{\"time\":\"" time "\",\"temp\":\"" temp "\"}";
server.send(200, "application/json", data);
});
server.on("/getHumData", []() {
String time = String(millis());
String hum = String(dht.readHumidity());
String data = "{\"time\":\"" time "\",\"hum\":\"" hum "\"}";
server.send(200, "application/json", data);
});
server.begin();
Serial.println("Server started");
}
void loop() {
server.handleClient();
}
CodePudding user response:
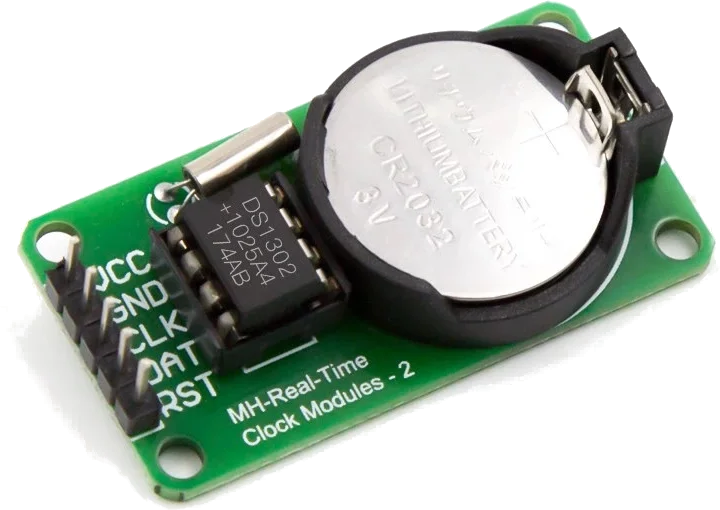