I have a custom keyboard view.
When user presses one of the UIButtons
I change its backgroundColor to imitate fade in\out effect.
Here is the code I use:
class NumpadButton: UIButton {
override var isHighlighted: Bool {
didSet {
if isHighlighted {
backgroundColor = UIColor(named: "FadeGrayColor")
} else {
UIView.animate(withDuration: 0.2) {
default: self.backgroundColor = UIColor(named: "NumpadGrayColor")
}
}
}
}
}
Here is how it looks:
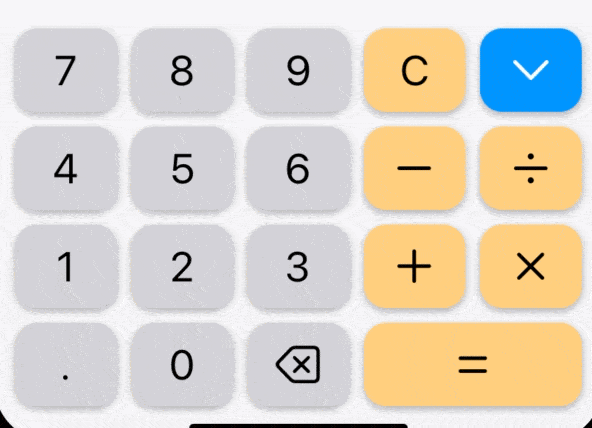
The problem:
If you tap on the same button very-very fast you won't see a fade in\out background color
change for taps after the first one, because UIView.animate(withDuration: 0.2)
should be ended and then the code can be run again. The second tap can be faster that animate part will end.
If you open standard iPhone Calculator app and try to do the same you'll notice it will fade in\out on every tap, no matter how fast it is.
If I remove UIView.animate
part then fade out will be straight, which I would like to avoid.
I tied to use also touchedBegan()
and touchedEnded()
methods but it has the same effect...
How can I replicate behaviour from Calculator app and run above code on every tap?
CodePudding user response:
See below:
class NumpadButton: UIButton {
override var isHighlighted: Bool {
didSet {
if isHighlighted {
backgroundColor = UIColor.systemYellow
} else {
// You can add options to adjust the animation
// The option 'beginFromCurrentState' fixes this
UIView.animate(withDuration: 0.2, delay: 0, options: [.curveEaseInOut, .beginFromCurrentState, .allowUserInteraction]) { [unowned self] in
backgroundColor = UIColor.systemGreen
}
}
}
}
}