I am trying to get the date and department info stored into an array, when a user inputs a value into my HTML. When I look at the console, I see it is being saved as E.fn.init. Here is my HTML code:
<form>
<p><b>Date:</b><input id="date_out" type='date' required /></p>
<p><b>Department:</b><input id='department_out' type='text' required /></p>
<button type="submit" id='submit' >SUBMIT</button>
</form>
And here is my Javascript Code:
let count = 1;
// Handler to Submit Data
$('#submit').click(() =>{
$('form').on('submit', function(e){
e.preventDefault();
const date_out = $("#date_out").val();
const department_out = $("#department_out").val();
let data = [];
// Iterate over all rows and store data
for (let i = 1; i <= count; i ){
// Skip Row if it was Removed
if (!$(`tr[index=${i}]`).length) continue;
// Store all Info from this row
let assetInfo = {
date_out: $(`#date_out${i}`).val(date_out),
department_out: $(`#department_out${i}`).val(department_out),
}
data.push(assetInfo);
console.log(data);
}
});
});
And the console prints the array as; date_out: E.fn.init, department_out: E.fn.init. How do I get it to save whatever the user inputs in the array?
CodePudding user response:
I am not quite sure what is your HTML structure but as I understand, you have a table and you want to store each row in a data, here is how I advise you to do it: In my Table called depTable, I have each row with a unique ID like this:
date_out_1
department_out_1
so, when I want to access that, I just want to create that ID which is an easy task while I can get how many rows I have in that table, like this:
// Get ROW COUNT FROM TABLE
var count = $('#depTable tr').length;
Now, if you combine in For loop you will get all IDs
for (let i = 1; i <= count; i ){
var idDate = "#date_out_" i;
var idDep = "#department_out_" i;
}
here is my all code, hope I helped to solve your problem.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Stack21</title>
<script src="js/jquery-3.6.0.min.js"></script>
</head>
<body>
<table id="depTable">
<thead>
<tr>
<td>Date</td>
<td>Department</td>
</tr>
</thead>
<tbody>
<tr>
<td>
<input id="date_out_1" type='date' required />
</td>
<td>
<input id='department_out_1' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_2" type='date' required />
</td>
<td>
<input id='department_out_2' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_3" type='date' required />
</td>
<td>
<input id='department_out_3' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_4" type='date' required />
</td>
<td>
<input id='department_out_4' type='text' required />
</td>
</tr>
</tbody>
</table>
<Button onclick="storeUserData()">Test me</Button>
<script>
let data = [];
function storeUserData(){
// Get ROW COUNT FROM TABLE
var count = $('#depTable tr').length;
console.log("Row Count: " count)
for (let i = 1; i <= count; i ){
var idDate = "#date_out_" i;
var idDep = "#department_out_" i;
let assetInfo = {
date_out: $(idDate).val(),
department_out: $(idDep).val()
}
data.push(assetInfo);
}
console.log(data);
}
</script>
</body>
</html>
Demo code here:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Stack21</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<!-- MY OFFLINE <script src="js/jquery-3.6.0.min.js"></script> -->
</head>
<body>
<table id="depTable">
<thead>
<tr>
<td>Date</td>
<td>Department</td>
</tr>
</thead>
<tbody>
<tr>
<td>
<input id="date_out_1" type='date' required />
</td>
<td>
<input id='department_out_1' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_2" type='date' required />
</td>
<td>
<input id='department_out_2' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_3" type='date' required />
</td>
<td>
<input id='department_out_3' type='text' required />
</td>
</tr>
<tr>
<td>
<input id="date_out_4" type='date' required />
</td>
<td>
<input id='department_out_4' type='text' required />
</td>
</tr>
</tbody>
</table>
<Button onclick="storeUserData()">Test me</Button>
<script>
let data = [];
function storeUserData(){
// Get ROW COUNT FROM TABLE
var count = $('#depTable tr').length;
console.log("Row Count: " count)
for (let i = 1; i <= count; i ){
var idDate = "#date_out_" i;
var idDep = "#department_out_" i;
let assetInfo = {
date_out: $(idDate).val(),
department_out: $(idDep).val()
}
data.push(assetInfo);
}
console.log(data);
}
</script>
</body>
</html>
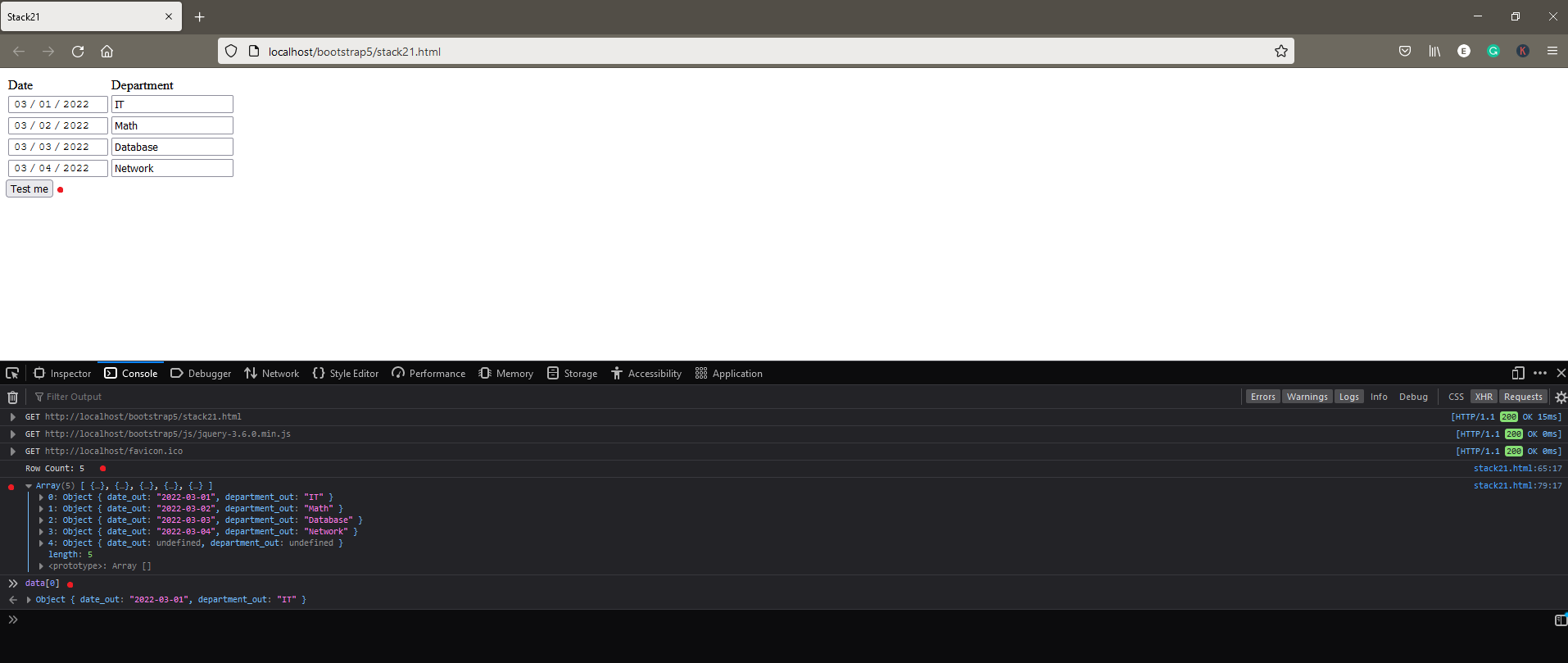