In a Row
, how can I ensure that the Text
with "Hello" in it is perfectly centered and not a bit to the left when the right most inner Row
widget has more items than the left most inner Row
widget?
Notice in the screenshot that the "Hello" is slight to the left.
I tried using a Stack
but that seems to not work well if the text is longer than the available space as it causes the text to then overlap the side-colored widgets.
I am using the following code:
Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.red,
),
],
),
Text(
"Hello",
textAlign: TextAlign.center,
),
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.purple,
),
Container(
width: 45,
height: 45,
color: Colors.green,
),
],
)
],
),
)
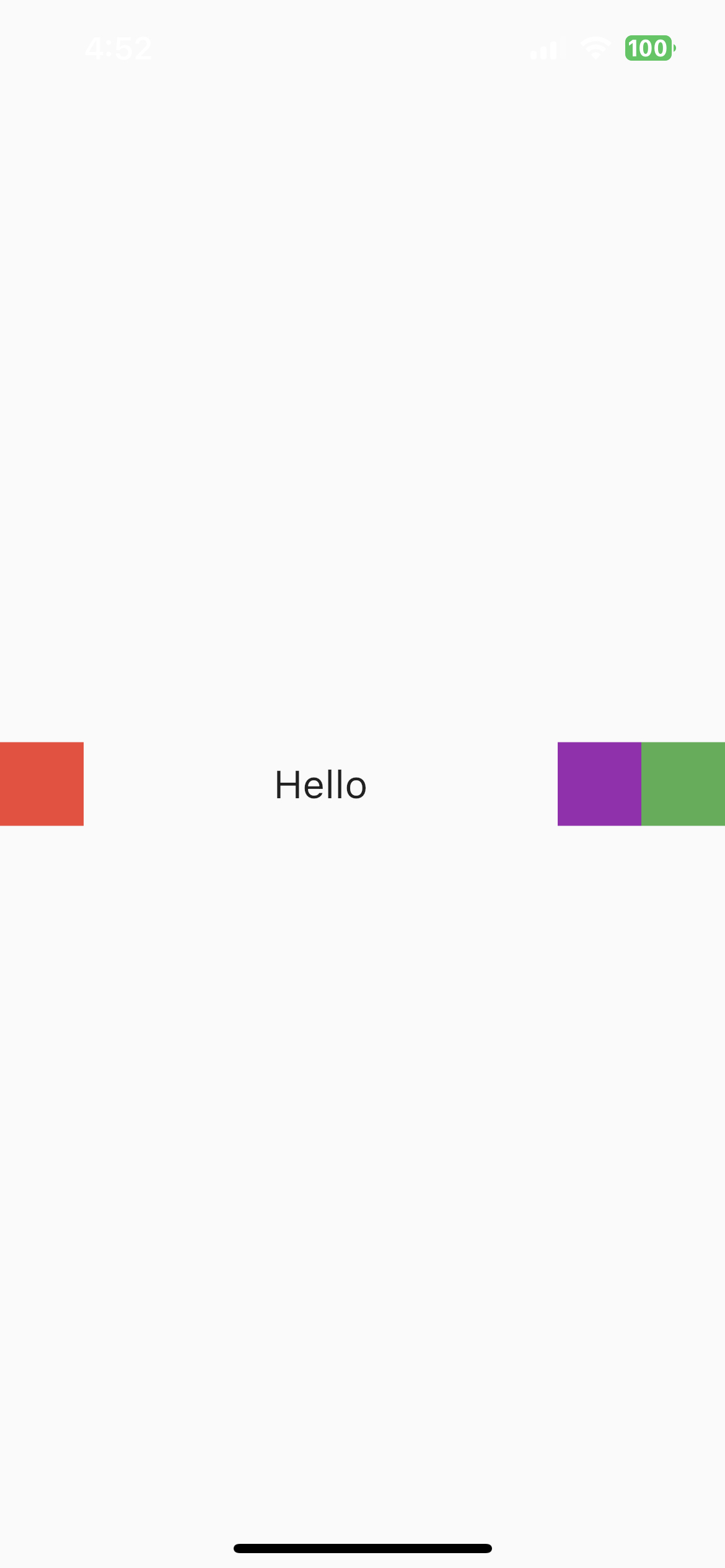
CodePudding user response:
Wrap both the Row
s with the Expanded
widget and set the mainAxisAlignmnet
of the right Row
to MainAxisAlignment.end
.
Also, if your text is long then you can wrap the Text widget with the Expanded too. Expanded(child: Text('...'))
.
Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Expanded(
child: Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.red,
),
],
),
),
const Text(
"Hello",
textAlign: TextAlign.center,
),
Expanded(
child: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Container(
width: 45,
height: 45,
color: Colors.purple,
),
Container(
width: 45,
height: 45,
color: Colors.green,
),
],
),
),
],
),
)
Output:
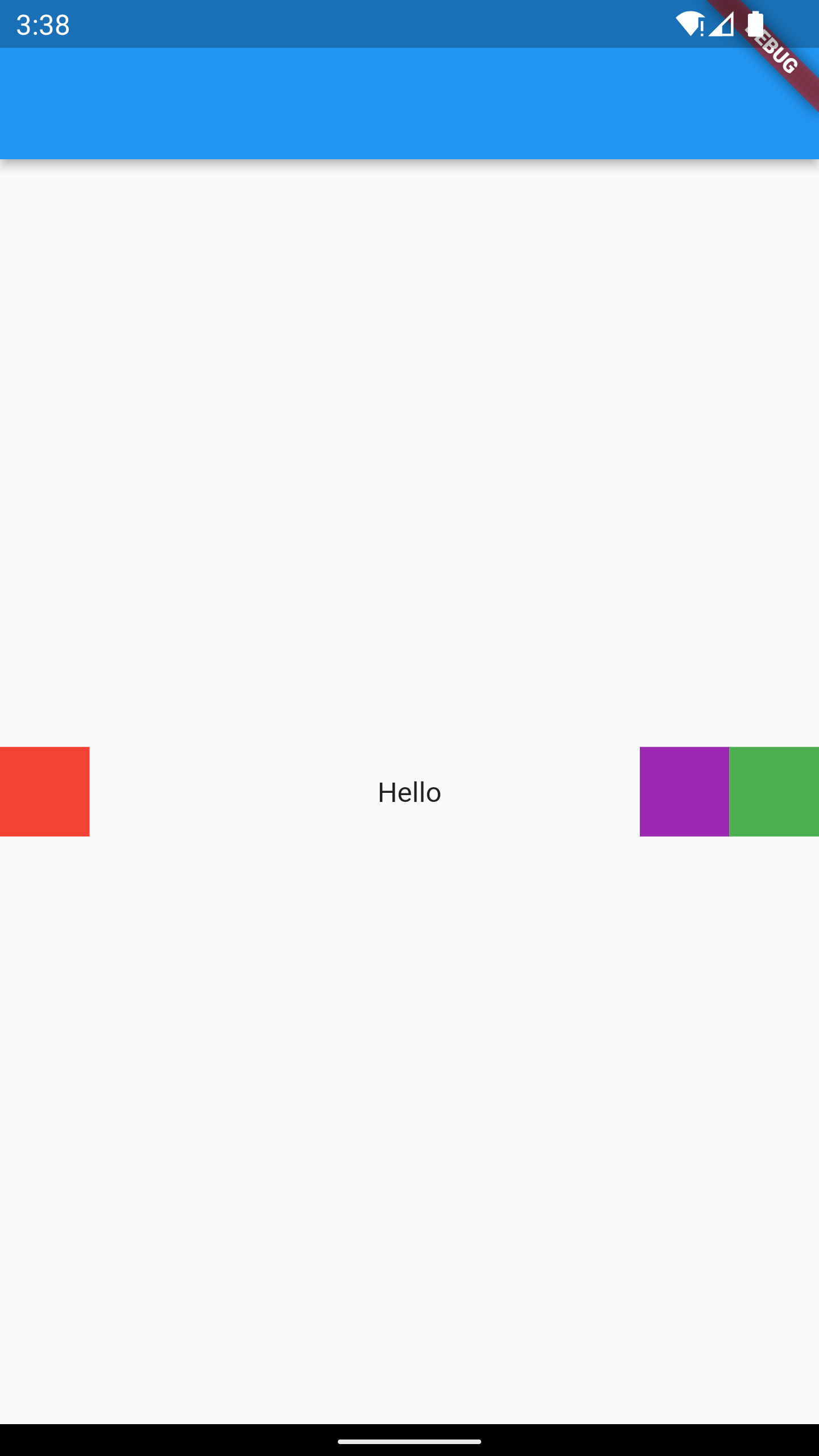
CodePudding user response:
If you don't have other widget width you can use stack
widget:
Stack(
alignment: Alignment.center,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.red,
),
],
),
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.purple,
),
Container(
width: 45,
height: 45,
color: Colors.green,
),
],
)
],
),
Text(
"Hello",
textAlign: TextAlign.center,
),
],
),
If your text is long, I recommend @OMiShah answer and wrap your text with expanded
widget.
CodePudding user response:
try the following, I made it with the Stack
widget:
Center(
child: Stack(
alignment: Alignment.center,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.red,
),
],
),
Row(
children: [
Container(
width: 45,
height: 45,
color: Colors.purple,
),
Container(
width: 45,
height: 45,
color: Colors.green,
),
],
)
],
),
Text(
"Hello",
textAlign: TextAlign.center,
),
],
),
);