I changed my method in the way when the thread interrupt himself:
public static void main(String[] args) throws InterruptedException {
System.out.println(Thread.currentThread().isInterrupted());
Runnable target = new Runnable() {
@Override
public void run() {
System.out.println("From thread-run(): " Thread.currentThread().isInterrupted());
Thread.currentThread().interrupt();
}
};
Thread someThread = new Thread(target);
System.out.println("Before start(): " someThread.isInterrupted());
someThread.start();
Thread.sleep(1000);
System.out.println("After start(): " someThread.isInterrupted());
Thread.sleep(1000);
System.out.println("After interrupt: " someThread.isInterrupted());
}
But the result is the same:
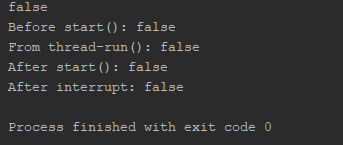
I supposed, the From thread-catch():
and After interrupt:
lines should have returned true instead of false - why isInterrupted() == false if the thread was interrupted?
the answer from
CodePudding user response:
The spec works precisely as it says it does. When InterruptedException
is thrown, the flag is also lowered as it does that. Programming goes by spec. It doesn't really care that you find an answer 'unacceptable'.
The second case gives you a false
because the thread is already dead. You interrupt your own thread, which does absolutely nothing except raise that flag. That raised flag will then have an effect only on methods that are explicitly marked as looking at it, such as Thread.sleep
(the next Thread.sleep
you would execute in that thread will end immediately, waiting no time at all, and end by throwing InterruptedException (which will, of course, also lower the flag).
At any rate, okay, so, the flag is up, you then call no methods that look at it, and the thread ends because it ran to the end of the run()
method. At which point, things like 'interrupted' status are irrelevant, and no longer tracked.
If you're calling isInterrupted()
, you're probably doing it wrong, but this question does not indicate why you (think you) need this, so it is not possible to name some good alternatives.
At any rate, if you interrupt a thread, then isInterrupted()
will return true until [A] that thread lowers the flag by calling Thread.interrupted()
, [B] same as A but be aware that other methods and notably a bunch of methods in java.*
do this for you. Notably, Thread.sleep
; after a sleep() call exits, the interrupt flag cannot possibly be raised anymore. If it was raised at any time before or during the sleep, the sleep method lowers it and exits by way of throwing InterruptedException. It does this. By design. You can't make it no do this, or [C] the thread ends entirely.
CodePudding user response:
Take a look at the javadoc of someThread.isInterrupted()
method: 'A thread interruption ignored because a thread was not alive at the time of the interrupt will be reflected by this method returning false.' - I think it is the reason.
Also you should know, that you must listen for 'interrupted status' on yourself. By checking Thread.currentThread().isInterrupted()
. And then may be throw new InterruptedException()
on yourself.
Try the code below. The loop logic just for 'waiting' - you cannot use Thread.sleep(10_000)
after thread interrupted.
public static void main(String[] args) throws InterruptedException {
System.out.println(Thread.currentThread().isInterrupted());
Runnable target = new Runnable() {
@Override
public void run() {
System.out.println("From thread-run(): " Thread.currentThread().isInterrupted());
Thread.currentThread().interrupt();
System.out.println("After interruption");
long sum = 0;
Random r = new Random();
for (int i = 0; i < Integer.MAX_VALUE; i ) {
sum = r.nextInt(3);
}
System.out.println(sum);
try {
Thread.sleep(10_000);
} catch (InterruptedException e) {
e.printStackTrace();
System.out.println("InterruptedException");
}
}
};
Thread someThread = new Thread(target);
System.out.println("Before start(): " someThread.isInterrupted());
someThread.start();
Thread.sleep(1000);
System.out.println("After start(): " someThread.isInterrupted());
}
Outputs:
false
Before start(): false
From thread-run(): false
After interruption
After start(): true
2147470801
java.lang.InterruptedException: sleep interrupted
at java.base/java.lang.Thread.sleep(Native Method)
at ru.eta2.Test$1.run(Test.java:20)
at java.base/java.lang.Thread.run(Thread.java:834)
InterruptedException
Process finished with exit code 0