I want to make several changes to UILabel
text style by using NSAttributedString
:
- Part of the string must have a solid
backgroundColor
; - Some parts of the string must have a different
font
; - Some parts of the string must have a different
foregroundColor
;
But when i made these changes, i ran into the problem that backgroundColor
has some spacing (vertical lines in the image) between (i think) other attributes (font
/foregroundColor
).
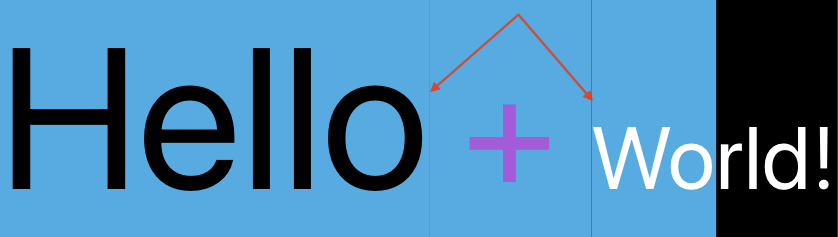
(See the full size image)
How can i fixed that?
Swift Playground code:
import PlaygroundSupport
import UIKit
let label = UILabel()
label.backgroundColor = .black
let text = "Hello World!"
let nsText = NSString(string: text)
let attributedString = NSMutableAttributedString(string: text)
attributedString.addAttribute(.backgroundColor, value: UIColor.systemCyan, range: nsText.range(of: "Hello Wo"))
attributedString.addAttribute(.font, value: UIFont.systemFont(ofSize: 100.0), range: nsText.range(of: "Hello"))
attributedString.addAttribute(.foregroundColor, value: UIColor.systemPurple, range: nsText.range(of: " "))
attributedString.addAttribute(.font, value: UIFont.systemFont(ofSize: 80.0), range: nsText.range(of: " "))
attributedString.addAttribute(.foregroundColor, value: UIColor.white, range: nsText.range(of: "World!"))
attributedString.addAttribute(.font, value: UIFont.systemFont(ofSize: 44.0), range: nsText.range(of: "World!"))
label.attributedText = attributedString
label.sizeToFit()
PlaygroundPage.current.liveView = label
CodePudding user response:
There is an attribute called expansion
that adjusts the font's expansion factor using a float value. The problem only occurs where two ranges with different font sizes meet, and this is a graphical rendering issue that cannot be overridden. But if you explicitly zero out the expansion factor, or even apply a small negative value, the gaps will not be noticeable.
attributedString.addAttribute(.expansion, value: NSNumber(value: 0), range: nsText.range(of: "Hello World!"))