I'm using a listview in a grid proportional row, and its other elements are in an auto row. Because I want my listview to take up the rest of the screen. So far everything is normal.
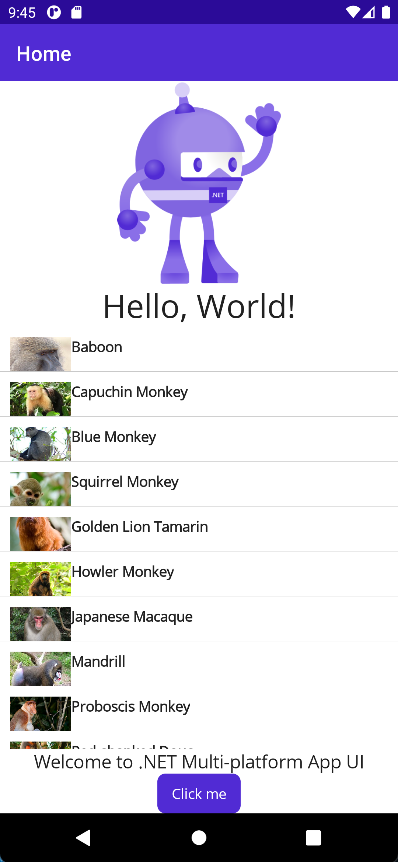
However, when I want to use a scrollview on the outside, the listview takes up the whole screen and the other elements are not visible.
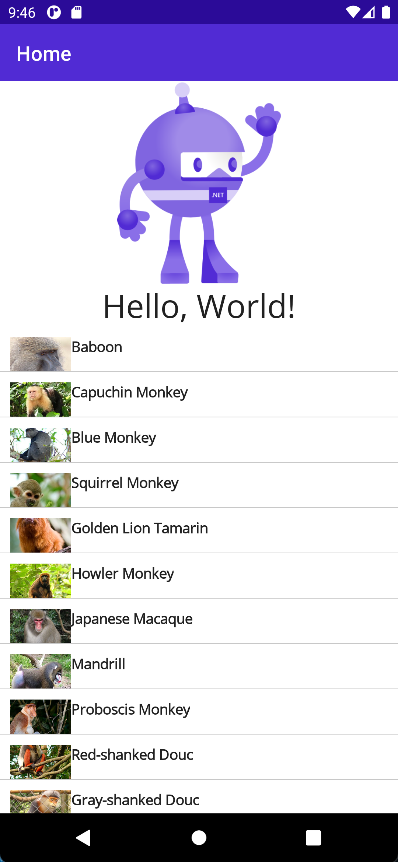
I want the listview to appear the same in the scrollview. Here I have to use scrollview because I am adding more elements (label, button, etc.) in grid dynamically. After a while, when the listview height reaches the end, the scrollview should kick in. I tried everything but failed
Many thanks to those who have helped so far.
Example code
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp4.MainPage">
<ScrollView VerticalOptions="Fill">
<Grid HorizontalOptions="Fill" VerticalOptions="Fill">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Image
Source="dotnet_bot.png"
SemanticProperties.Description="Cute dot net bot waving hi to you!"
HeightRequest="200"
HorizontalOptions="Center" />
<Label Grid.Row="1"
Text="Hello, World!"
SemanticProperties.HeadingLevel="Level1"
FontSize="32"
HorizontalOptions="Center" />
<ListView x:Name="listView"
Grid.Row="2"
VerticalOptions="Fill"
ItemsSource="{Binding Monkeys}"
MinimumHeightRequest="200">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<ViewCell.ContextActions>
<MenuItem Text="Favorite"
IconImageSource="favorite.png"
Command="{Binding Source={x:Reference listView}, Path=BindingContext.FavoriteCommand}"
CommandParameter="{Binding}" />
<MenuItem Text="Delete"
IconImageSource="delete.png"
Command="{Binding Source={x:Reference listView}, Path=BindingContext.DeleteCommand}"
CommandParameter="{Binding}" />
</ViewCell.ContextActions>
<Grid Padding="10">
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Image Grid.RowSpan="2"
Source="{Binding ImageUrl}"
Aspect="AspectFill"
HeightRequest="60"
WidthRequest="60" />
<Label Grid.Column="1"
Text="{Binding Name}"
FontAttributes="Bold" />
<Label Grid.Row="1"
Grid.Column="1"
Text="{Binding Location}"
FontAttributes="Italic"
VerticalOptions="End" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
<Label Grid.Row="3"
Text="Welcome to .NET Multi-platform App UI"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome to dot net Multi platform App U I"
FontSize="18"
HorizontalOptions="Center" />
<Button Grid.Row="4"
x:Name="CounterBtn"
Text="Click me"
SemanticProperties.Hint="Counts the number of times you click"
Clicked="OnCounterClicked"
HorizontalOptions="Center" />
</Grid>
</ScrollView>
</ContentPage>
CodePudding user response:
You want your "listview to take up the rest of the screen", and at the same time, you are wrapping this grid in a ScrollView, removing the limitation.
Do not wrap your Vertical ListView in Vertical ScrollView. Or, if you do, make sure the height of you ListView is limited.
Otherwise you will be scrolling on fully expanded ListView.
CodePudding user response:
You have the ScrollView in the wrong level and you shouldn't nest a ListView inside a ScrollView, so better leave out the ScrollView entirely since the ListView provides scrolling capabilities on its own:
<Grid RowDefinitons="auto, auto, *, auto, auto">
<!-- leaving out irrelevant bits -->
<ListView Grid.Row="2">
<!-- leaving out irrelevant bits -->
</ListView>
<Label />
<Button />
</Grid>
https://learn.microsoft.com/en-us/xamarin/xamarin-forms/user-interface/layouts/scrollview