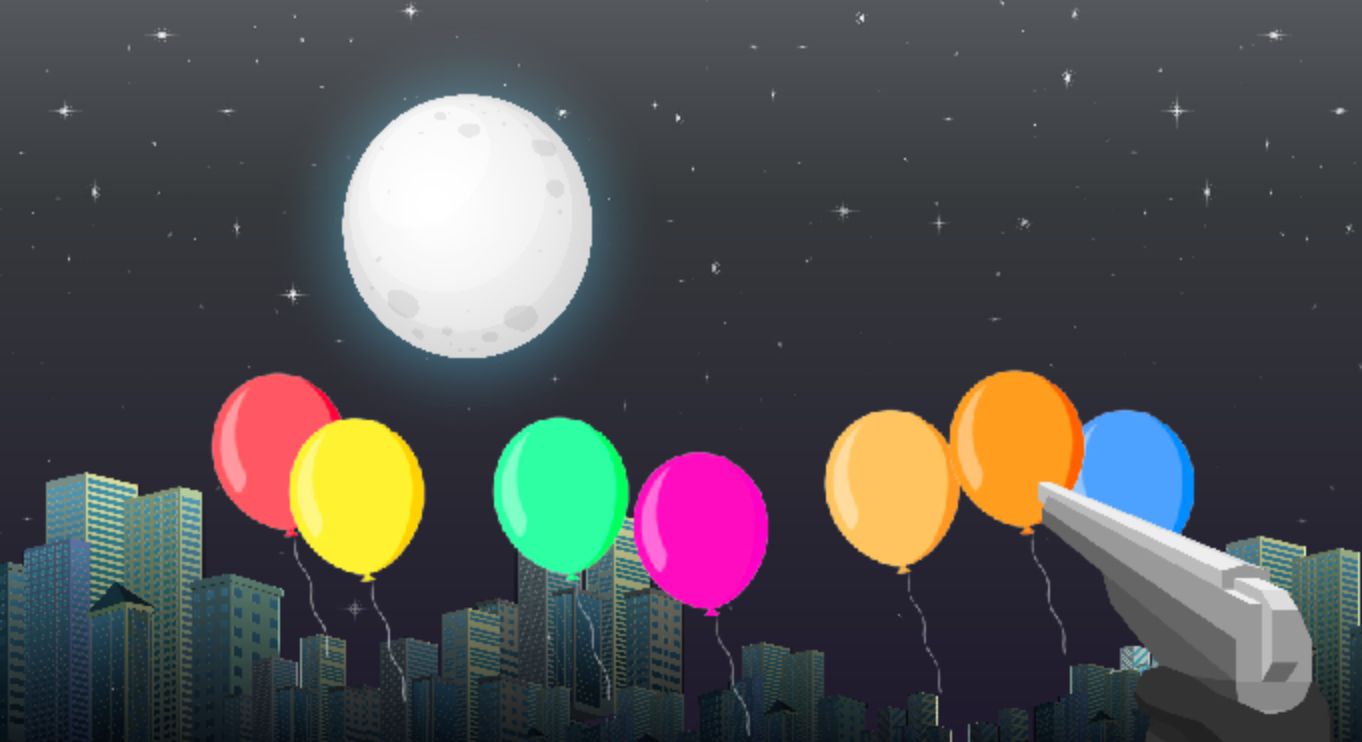
(When the gun is in the same position as the balloon, it should print out which balloon it hit.)
How can I check the collisions between these images properly, and print out which balloon was hit?
CodePudding user response:
pygame.Surface.get_rect.get_rect()
returns a rectangle with the size of the Surface object, that always starts at (0, 0) since a Surface object has no position. A Surface is blit
at a position on the screen. The position of the rectangle can be specified by a keyword argument. For example, the top left of the rectangle can be specified with the keyword argument topleft
. e.g.:
gun_rect = gun.get_rect(topleft = (x, y))
Read How do I detect collision in pygame? and use pygame.Rect.collidepoint
to detect the collision of the gun tip (gun_rect.topleft
) with the enclosing rectangle of a balloon (ballon_rect
):
def check_collisions(x, y):
for i in range(num_balloons):
gun_rect = gun.get_rect(topleft = (x,y))
ballon_rect = colors[i].get_rect(topleft = (balloon_list[i] - 100, y-90))
if ballon_rect.collidepoint(gun_rect.topleft):
print(f'collision with {colors[i]}')
while running:
check_collisions(x, y)
Note, that the position of the balloon is (balloon_list[i] - 100, y-90)
, since you are drawing it at that position:
def draw_balloons(y):
for i in range(num_balloons):
screen.blit(colors[i], (balloon_list[i] - 100, y-90))