I'm new to android development and I'm trying to make a screen (a layout) that resembles this: a spinner, and two text fields on top, a thumbnail (ImageView) just below on the left side, and a fragment container view below that I will use to show a different page. Right now I'm not looking for beautiful design, I just want to make android display it like this.
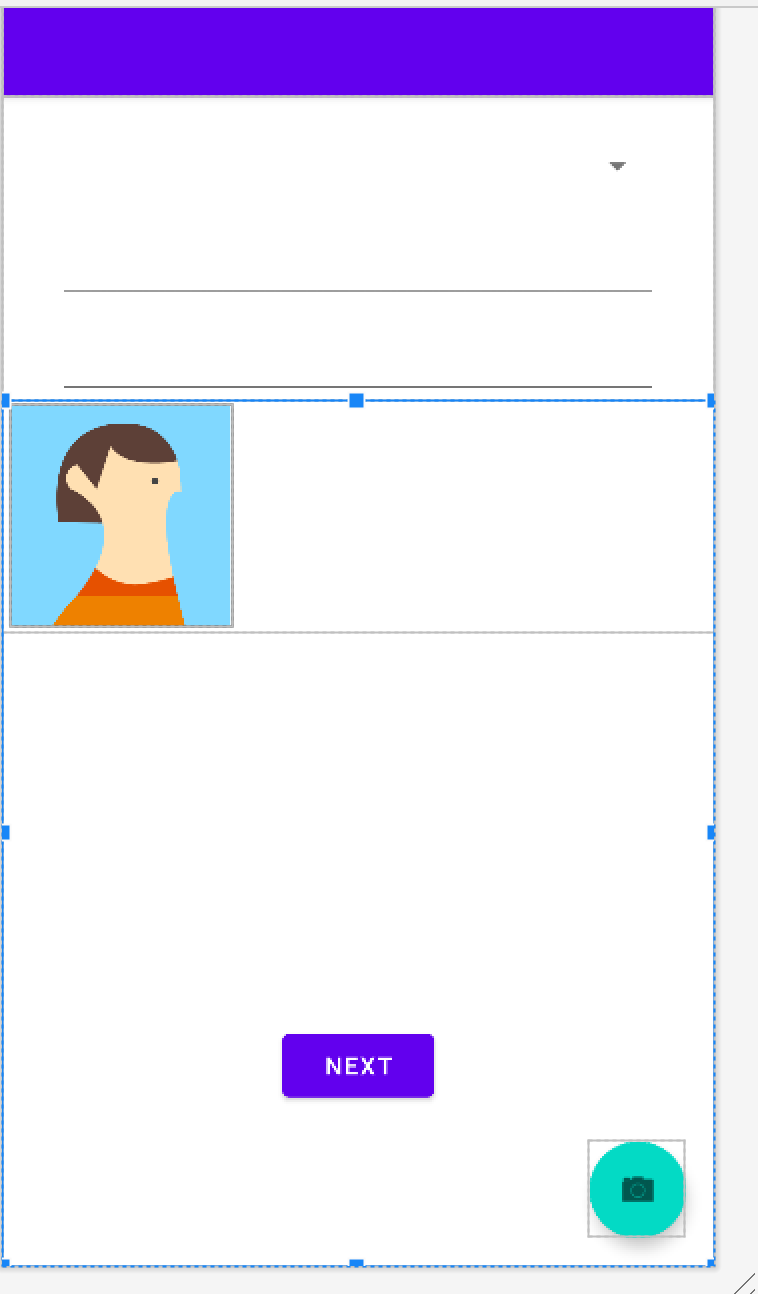
The problem is that, even though on the design tab of android studio the thumbnail is shown in the correct place, on runtime is completely wrong:
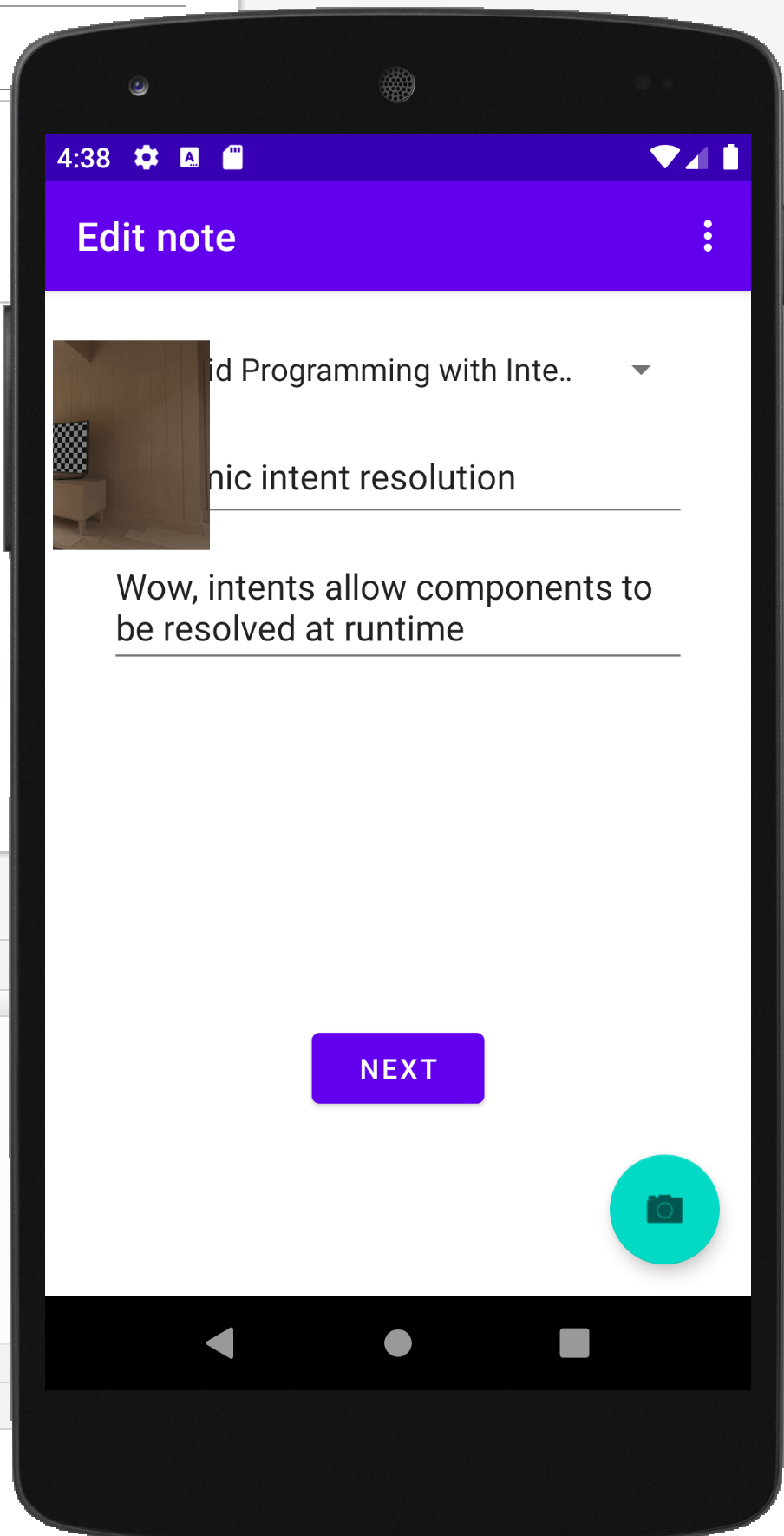
Here is the Component Tree and the layout's xml in case it's useful:
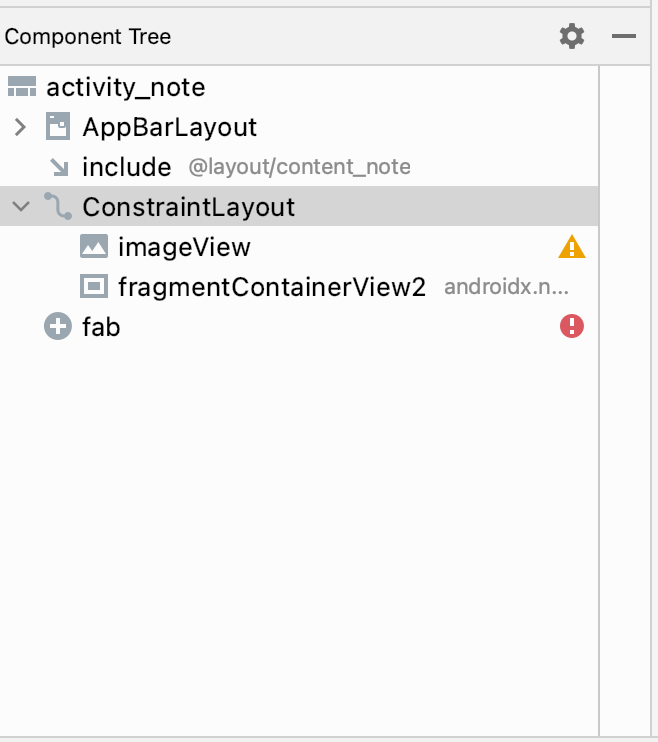
<androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@ id/activity_note"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".NoteActivity">
<com.google.android.material.appbar.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:theme="@style/Theme.NoteKeeper.AppBarOverlay">
<androidx.appcompat.widget.Toolbar
android:id="@ id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/Theme.NoteKeeper.PopupOverlay" />
</com.google.android.material.appbar.AppBarLayout>
<include
android:id="@ id/include"
layout="@layout/content_note" />
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="500dp"
android:layout_gravity="bottom|center">
<ImageView
android:id="@ id/imageView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="center|left"
android:layout_marginStart="4dp"
app:layout_constraintBottom_toTopOf="@ id/fragmentContainerView2"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@tools:sample/avatars" />
<androidx.fragment.app.FragmentContainerView
android:id="@ id/fragmentContainerView2"
android:name="androidx.navigation.fragment.NavHostFragment"
android:layout_width="match_parent"
android:layout_height="367dp"
android:layout_gravity="bottom|center"
app:defaultNavHost="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:navGraph="@navigation/nav_graph" />
</androidx.constraintlayout.widget.ConstraintLayout>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@ id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_marginEnd="@dimen/fab_margin"
android:layout_marginBottom="16dp"
app:srcCompat="@android:drawable/ic_menu_camera" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
EDIT:
In the end, I had to use constraint layout like this:
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@ id/activity_note"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".NoteActivity">
<com.google.android.material.appbar.AppBarLayout
android:id="@ id/appBarContentNoteLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:theme="@style/Theme.NoteKeeper.AppBarOverlay"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<androidx.appcompat.widget.Toolbar
android:id="@ id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/Theme.NoteKeeper.PopupOverlay" />
</com.google.android.material.appbar.AppBarLayout>
<include
android:id="@ id/include"
layout="@layout/content_note"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@ id/appBarContentNoteLayout" />
<ImageView
android:id="@ id/imageView"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@ id/include"
tools:srcCompat="@tools:sample/avatars" />
<androidx.fragment.app.FragmentContainerView
android:id="@ id/fragmentContainerView"
android:name="androidx.navigation.fragment.NavHostFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:background="#AC1A1A"
android:backgroundTint="#C51111"
app:defaultNavHost="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintTop_toBottomOf="@ id/imageView"
app:navGraph="@navigation/nav_graph" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@ id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_marginEnd="32dp"
android:layout_marginBottom="32dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:srcCompat="@android:drawable/ic_menu_camera" />
</androidx.constraintlayout.widget.ConstraintLayout>
Added tint so that the fragment view would show the borders
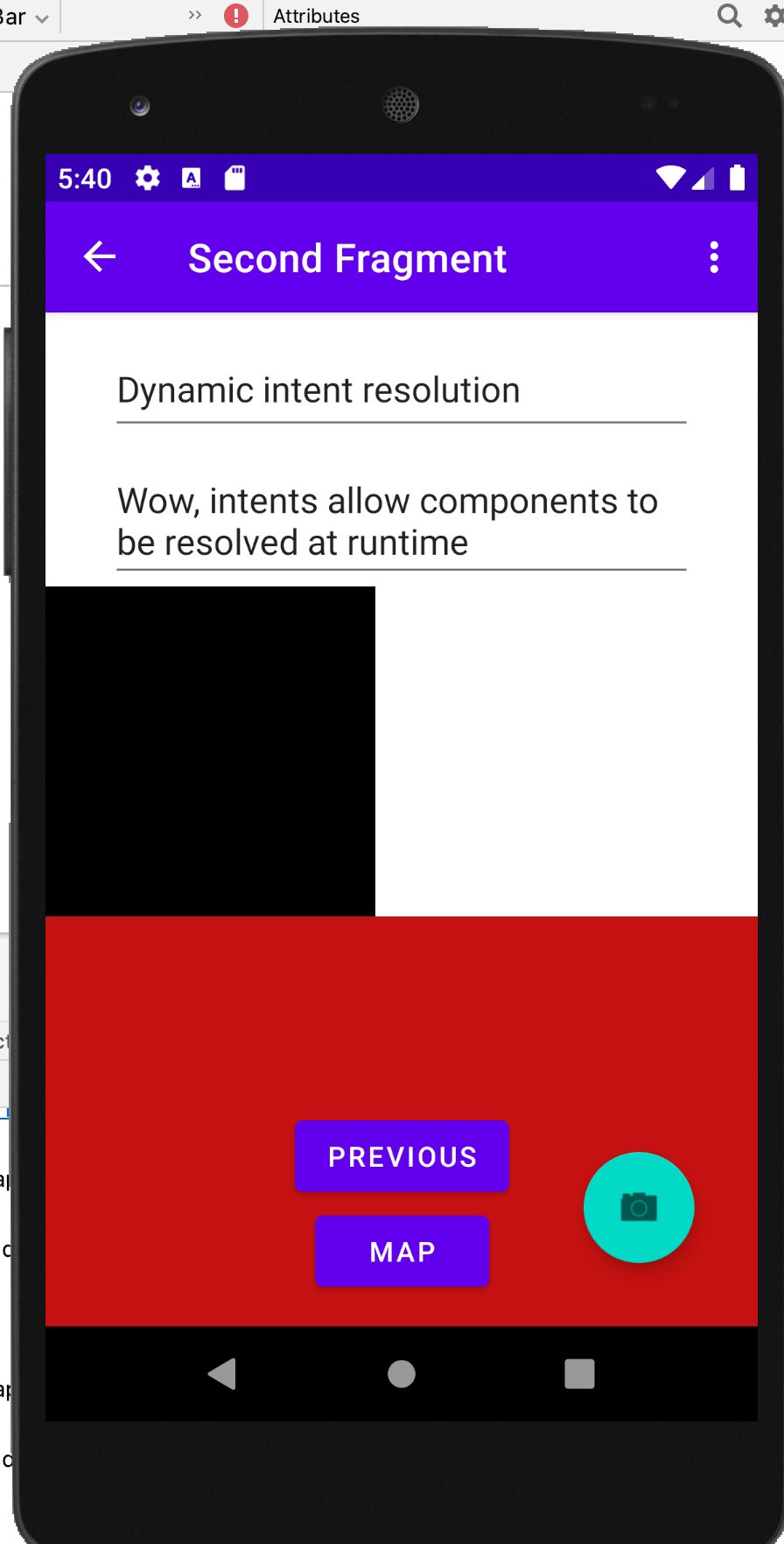
CodePudding user response:
The problem is you are using layout_gravity in your xml code that works in some view groups like FrameLayout
but it doesn't work in ConstraintLayout
. And the second fact is you don't need to use nested ConstraontLayouts and one will handle All of your ui requirements. So for the first one that is AppBar
you can use app:layout_constraintTop_toTopOf="parent"
and for content note, you can constraint it to bottom of the appBar as well. Like this: app:layout_constraintTop_toBottomOf="@ id/appBar"
and also for the image thumbnail use this: app:layout_constraintTop_toBottomOf="@ id/contentNote"
together with app:layout_constraintStart_toStartOf="parent"
and for the last one as fragmentContainer you can use this app:layout_constraintTop_toBottomOf="@ id/imageView"
.
This way all of them will be constrainted to each other and works well. And for any other constraints for them related to the parent layout, you can add their constraints as well.
CodePudding user response:
Your constraints are wrong in the ImageView
.
Try getting rid of the following line
app:layout_constraintTop_toTopOf="parent"
in your ImageView
as this line of code is trying to pull the top of your image all the way to the top of the parent.
Lmk if that works. Otherwise, I can send you a complete layout code snippet instead.