I am creating a custom Numpad keyboard through xib.
The numpad has 4 rows: 3 rows with 5 buttons and 1 last row with 4 buttons.
I want to make all the buttons round.
Here is how I initialize the Numpad view:
override init(frame: CGRect) {
super.init(frame: frame)
initializeSubviews()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
initializeSubviews()
}
func initializeSubviews() {
let xibFileName = "Numpad"
let view = Bundle.main.loadNibNamed(xibFileName, owner: self, options: nil)![0] as! UIView
self.addSubview(view)
view.frame = self.bounds
}
Here is how I initialize the Calculator Button:
class CalculatorButton: UIButton {
override var isHighlighted: Bool {
didSet {
if isHighlighted {
alpha = 0.5
} else {
UIView.animate(withDuration: 0.5, delay: 0.0, options: .curveLinear) {
self.alpha = 1.0
}
}
}
}
override init(frame: CGRect) {
super.init(frame: frame)
setup()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
setup()
}
private func setup() {
clipsToBounds = true
layer.cornerRadius = frame.width / 2
}
}
And this is how I load the numpad in VC:
override func viewDidLoad() {
super.viewDidLoad()
textField.delegate = self
let numpadView = Numpad(frame: CGRect(x: 0, y: 0, width: 0, height: 300))
textField.inputView = numpadView
}
But when numpad appears, buttons doesn't have an equal width and a height, thus I receive:
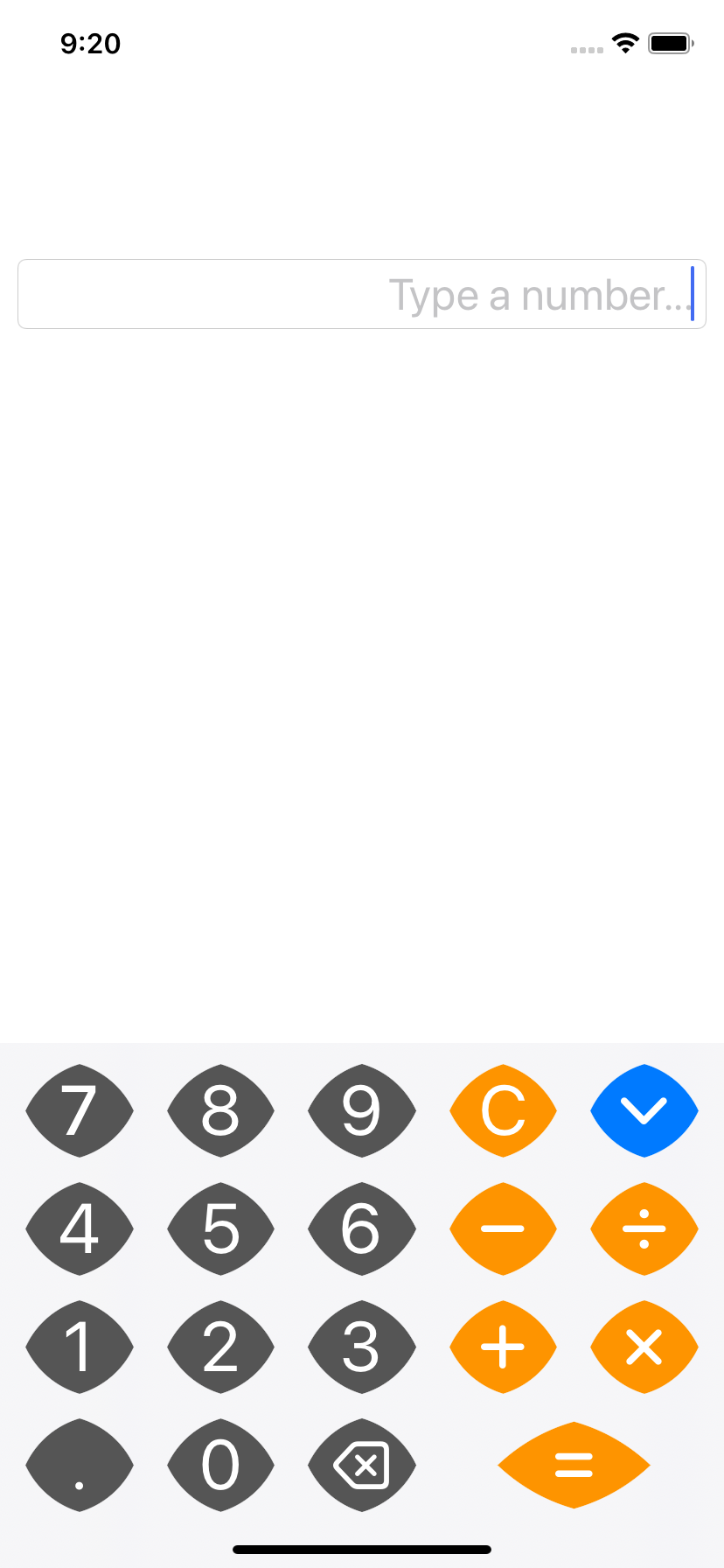
To solve the problem I tried to add an Aspect Ratio
with 1:1 Multiplier to force width and a height to be equal to receive a proper round buttons. But it's not working - the height and width are still the different when I load the numpad.
What should I do to receive rounded buttons at any numpad width and a height which can be set?
Here is the test project on Github: CLICK
CodePudding user response:
You need to add this to the CalculatorButton
class
override func layoutSubviews() {
super.layoutSubviews()
clipsToBounds = true
layer.cornerRadius = frame.height / 2
}
To adjust buttons width and height equal
1- Select 7 button and make aspect constraint 1:1 to itself
2- Delete bottom constraint of top most stackView
Here Xib