I am making a news app, with the use of News API, where it shows the top news of the day at the app's main screen. I created a UITableView
to store the photos and the titles of the articles but neither of them are appearing correctly.
The code bellow is how I fetch the data and store the properties of the cells in an array called topNewsArray
, containing the cells properties inside structs named CellArticle
. If there is no image to store, it will display a newspaper icon provided by SF Symbols.
func getTopNews() {
currentLocation = Locale.current.regionCode?.lowercased() ?? "us"
let urlString: String = "https://newsapi.org/v2/top-headlines?country=\(currentLocation)&apiKey=\(apiKey)"
let url = URL(string: urlString)
// Check if url is available.
guard url != nil else {
return
}
// Create URLSession to download and upload data.
let session = URLSession.shared
// Create a task that retrieves the contents of the specified URL.
let dataTask = session.dataTask(with: url!) { data, response, error in
// Check for errors.
if error == nil && data != nil {
// Parse JSON.
let decoder = JSONDecoder()
do {
let topNewsFeed = try decoder.decode(NewsFeed.self, from: data!)
let topNewsCount: Int = (topNewsFeed.articles?.count)!
// Add news to the topNewsArray.
for i in 0..<topNewsCount {
let cellTitle = topNewsFeed.articles?[i].title
if topNewsFeed.articles?[i].urlToImage != nil {
let cellImage = topNewsFeed.articles?[i].urlToImage
self.topNewsArray.append(CellArticle(title: cellTitle, imageName: cellImage))
}
else {
self.topNewsArray.append(CellArticle(title: cellTitle, imageName: "newspaper"))
}
print("News Title: ", cellTitle!, "\n")
}
}
catch {
print("Error in JSON Parsing.")
}
}
}
// Make the API Call.
dataTask.resume()
}
The message from the print("News Title: ", cellTitle!, "\n")
does work, showing all the top news of the day.
So I think the problem is at the tableView
function, where I typed the following code to populate it with the cells containing the data from their respective CellArticle
from the topNewsArray
:
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let currentArticle = topNewsArray[indexPath.row]
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as! CustomTableViewCell
cell.label.text = currentArticle.title
if currentArticle.imageName == "newspaper" {
cell.iconImageView.image = UIImage(systemName: "newspaper")
}
else {
let urlToImage = URL(string: currentArticle.imageName!)
let dataImage = try? Data(contentsOf: urlToImage!)
cell.iconImageView.image = UIImage(data: dataImage!)
}
return cell
}
The result I expected is something like this at the Main storyboard:
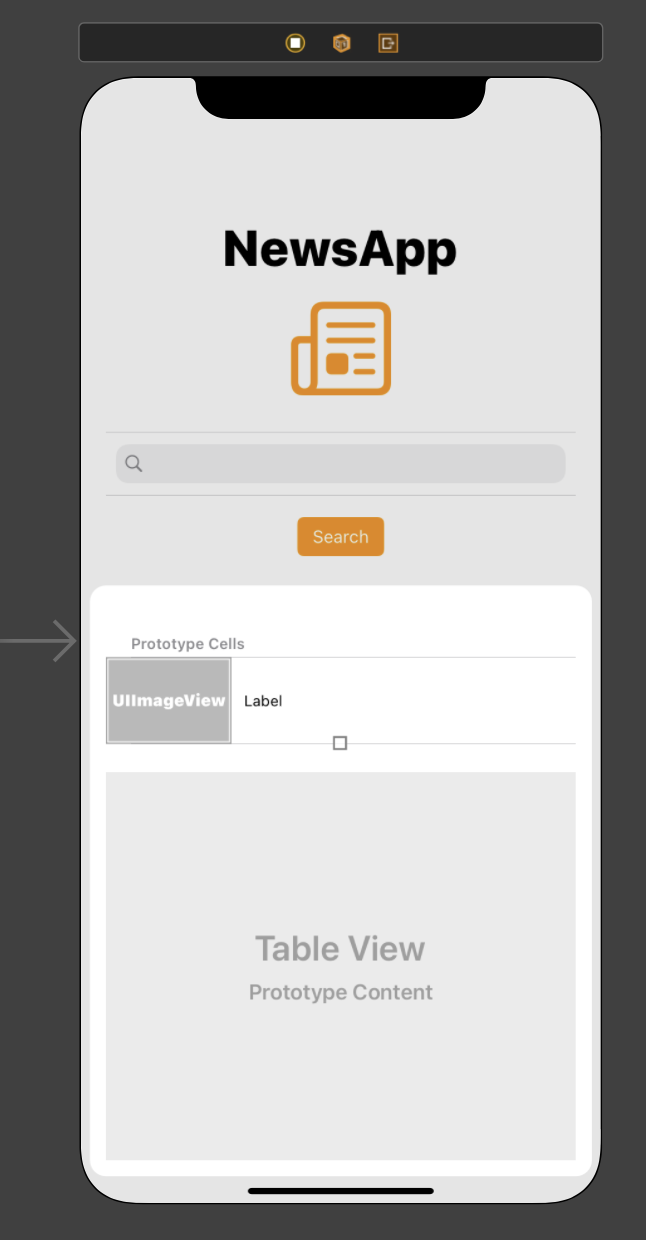
But I am getting an empty table, like this:
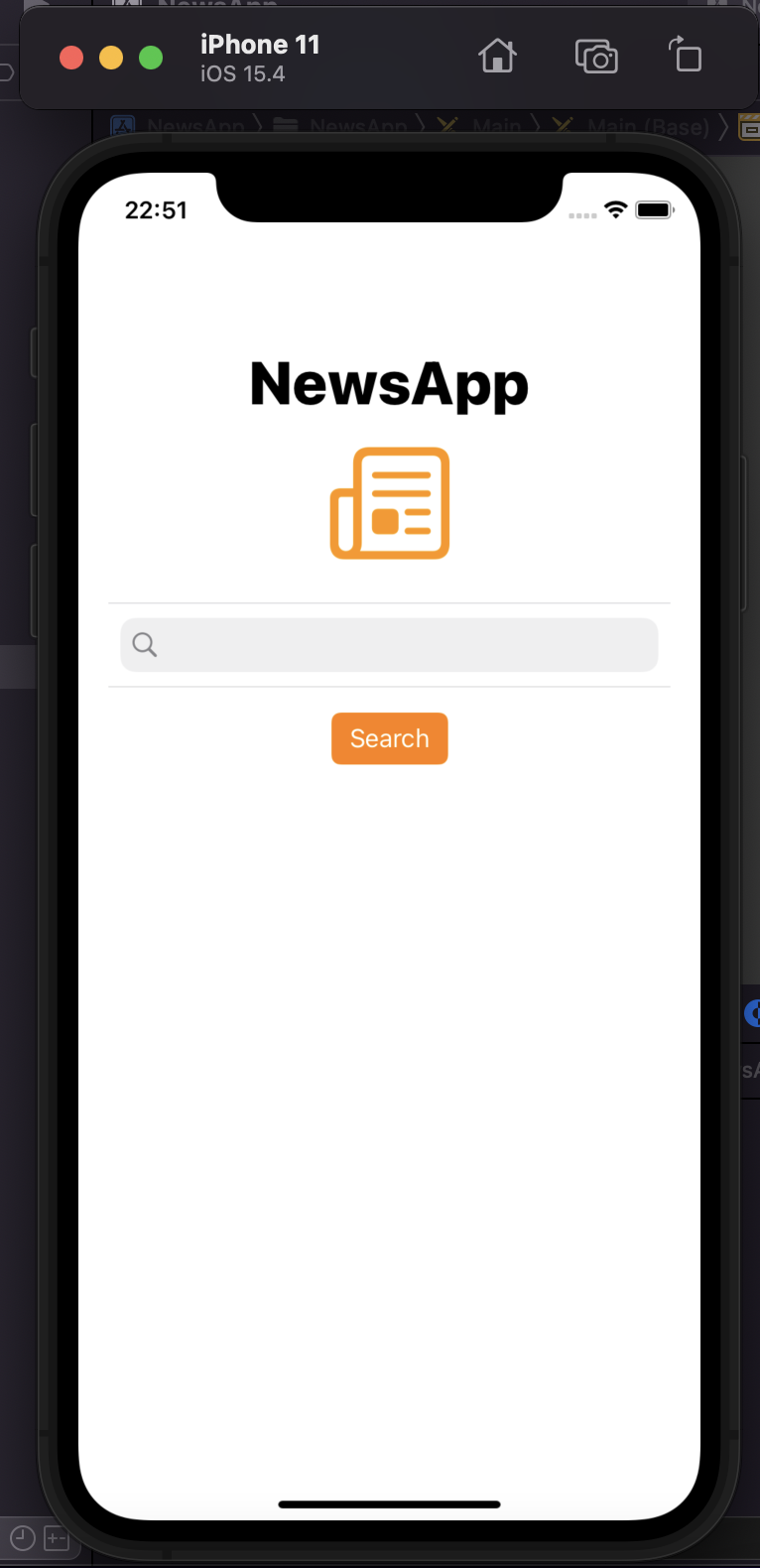
CodePudding user response:
Solved it, just needed to put this code after the for loop
DispatchQueue.main.async {
self.table.reloadData()
}
CodePudding user response:
You just have to add this in your for loop:
self.tableView.reloadData()
where tableView will be the name of your table view.